How to Call an Action From Within Another Action in Vuex ?
Last Updated :
13 Jan, 2024
Vuex is a state management library for Vue applications. As we know passing props can be tedious for complex applications with many components, Vuex makes this interaction very seamless and scalable. In Vuex, actions are functions that perform asynchronous operations and commit mutations to modify the state. In this article, we will learn How to call an action from within another action in Vuex.
Approach 1: Dispatching from Component
In this approach, you dispatch the second action from the component that calls the first action. This is a straightforward method and is commonly used in Vuex.
Syntax:
const store = new Vuex.Store({
actions: {
firstAction(context) {
// Your first action logic
// and Call the second action
},
secondAction() {
// Your second action logic
},
},
});
new Vue({
el: '#app',
store,
methods: {
callFirstAction() {
// Call the first action
},
},
});
Example: The below code example uses the component dispatch approch to call an action from within another action in Vuex.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< script src =
</ script >
< script src =
</ script >
</ head >
< body >
< div id = "app" >
< h2 style = "color: green;" >
GeeksforGeeks
</ h2 >
< h2 >
How to call an action from within
another action in Vuex?
</ h2 >
< button @ click = "callFirstAction" >
Call First Action
</ button >
</ div >
< script >
const store = new Vuex.Store({
actions: {
firstAction(context) {
console.log
('Executing first action');
// Your first action logic
// Call the second action
context.dispatch('secondAction');
},
secondAction() {
console.log
('Executing second action');
// Your second action logic
},
},
});
new Vue({
el: '#app',
store,
methods: {
callFirstAction() {
console.log
('Calling first action from component');
// Call the first action
this.$store.dispatch('firstAction');
},
},
});
</ script >
</ body >
</ html >
|
Output:
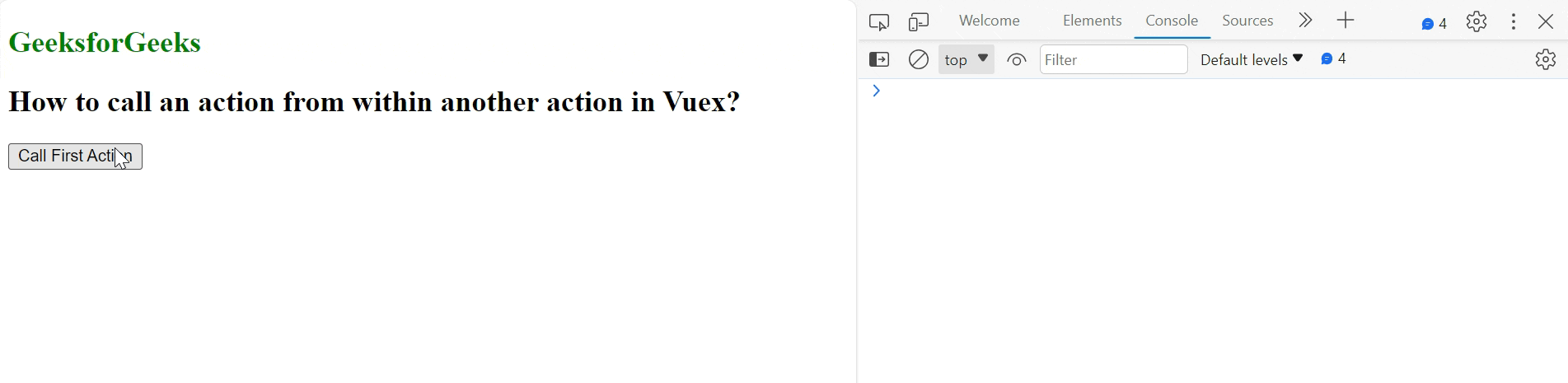
Approach 2: Using root parameter
In this example, the root parameter is used in the dispatch call within the firstAction to specify that secondAction should be called from the root of the store. This way, you can directly call actions from the root store, regardless of the module hierarchy
Syntax:
const store = new Vuex.Store({
state: {
},
mutations: {
},
actions: {
firstAction({ commit, dispatch }) {
},
secondAction({ commit }) {
}
}
});
Example: The below code example implements the root parameter approach to call an action within another action in Vuex.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >
Vuex Root Action Call
</ title >
</ head >
< body >
< div id = "app" >
< h2 style = "color: green;" >
GeeksforGeeks
</ h2 >
< h2 >
How to call an action from within
another action in Vuex template?
</ h2 >
< p >Counter: {{ counter }}</ p >
< button @ click = "callActions" >
Call Actions
</ button >
</ div >
< script src =
</ script >
< script src =
</ script >
< script >
const store = new Vuex.Store({
state: {
counter: 0
},
mutations: {
incrementCounter(state) {
state.counter++;
},
decrementCounter(state) {
state.counter--;
}
},
actions: {
firstAction({ commit, dispatch }) {
// Increment counter in the
// first action
commit('incrementCounter');
// Directly call another action
// from the root of the store
dispatch('secondAction', null, { root: true });
},
secondAction({ commit }) {
// Log a message and decrement counter
// in the second action
setTimeout(() => {
console.log('Second action executed.');
commit('decrementCounter');
}, 2000);
}
}
});
new Vue({
el: '#app',
store,
computed: {
counter() {
return store.state.counter;
}
},
methods: {
callActions() {
store.dispatch('firstAction');
}
}
});
</ script >
</ body >
</ html >
|
Output:
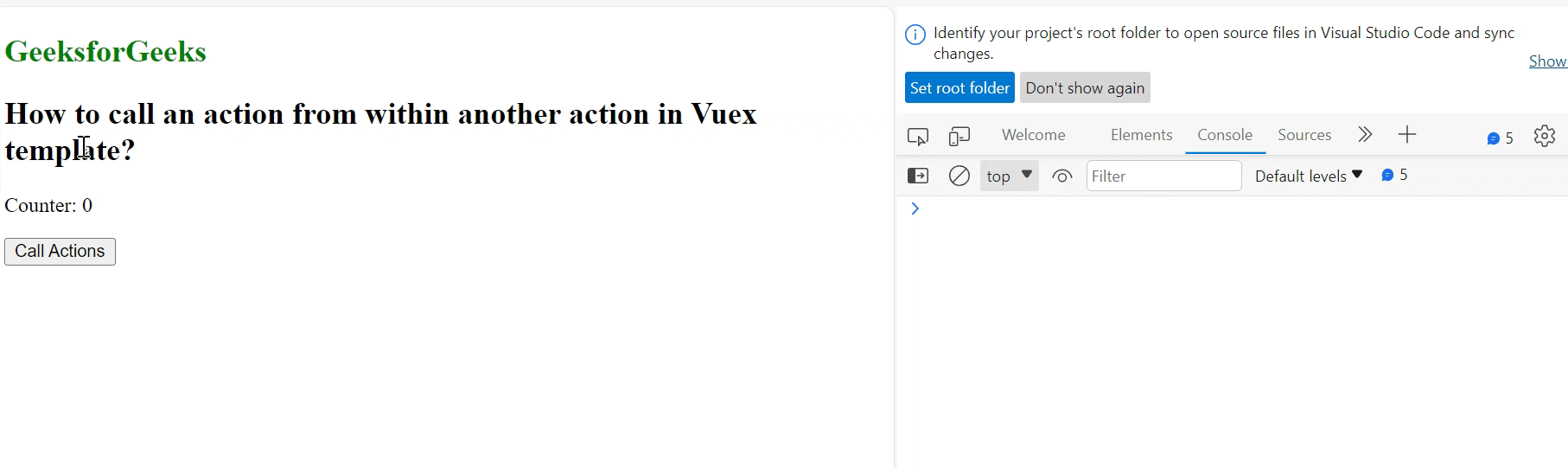
Conclusion
In both cases, the Vue instance includes a method (callFirstAction) that dispatches the first action (‘firstAction’). The Vuex store defines two actions (firstAction and secondAction), and within firstAction, the second action is dispatched using context.dispatch(‘secondAction’).
Share your thoughts in the comments
Please Login to comment...