React Chakra UI Form Switch
Last Updated :
19 Feb, 2024
Chakra UI Form Switch is a component provided by Chakra UI for creating toggle switches in React applications. It is an alternative to checkboxes, allowing users to switch between enabled and disabled states. Using the props of the Chakra UI Form Switch we can customize its appearance by changing the state, behavior, sizes, appearance, etc. In this article, we will explore the practical implementation of the Chakra UI Form Switch component by demonstrating all the types of switches.
Prerequisites:
Approach:
We have created different Chakra UI Form Switch components like a size-based, disabling switch, focused switch, invalid, read-only state, etc. Using this Chakra UI Form Switch component, we can embed it into the real-time application as an alternative to the traditional checkboxes. This increases the modern perspective of the application and enables the user to engage with the application.
Steps to Create React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app chakra
Step 2: After creating your project folder(i.e. chakra), move to it by using the following command:
cd chakra
Step 3: After creating the React application, Install the required package using the following command:
npm i @chakra-ui/react @emotion/react@^11 @emotion/styled@^11 framer-motion@^6
Project Structure:
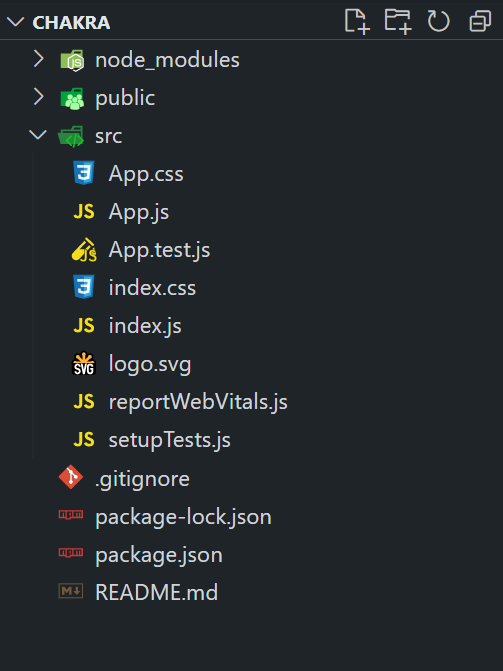
The updated dependencies are in the package.json file.
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.3",
"@emotion/styled": "^11.11.0",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"framer-motion": "^6.5.1",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: Below is the practical implementation of the Chakra UI Form Switch.
Javascript
import React from 'react' ;
import {
ChakraProvider,
Box,
Flex,
FormControl,
FormLabel,
Switch,
Stack,
Heading,
} from '@chakra-ui/react' ;
function App() {
return (
<ChakraProvider>
<Box p={5}>
<Heading as= "h1" mb={4}
color= "green.500" >
GeeksforGeeks
</Heading>
<Heading as= "h3" mb={4}>
Chakra UI Form Switch
</Heading>
<Flex
direction={{
base: 'column' ,
md: 'row'
}}
alignItems={{
base: 'center' ,
md: 'flex-start'
}}
mb={4}
>
<FormLabel htmlFor= 'email-alerts'
mb={{ base: '2' , md: '0' }}>
Receive Email Alerts?
</FormLabel>
<Switch id= 'email-alerts'
colorScheme= 'green' />
</Flex>
<Stack align= 'center' direction= 'row'
spacing={4} mb={4}>
<Switch size= 'sm' colorScheme= 'teal' />
<Switch size= 'md' colorScheme= 'teal' />
<Switch size= 'lg' colorScheme= 'teal' />
</Stack>
<Stack direction= 'row' spacing={4} mb={4}>
<Switch colorScheme= 'red' size= 'lg' />
<Switch colorScheme= 'teal' size= 'lg' />
</Stack>
<Stack
direction={{ base: 'column' , md: 'row' }}
spacing={4}
mb={4}
>
<FormControl as={Stack} spacing={4}
mb={{ base: '4' , md: '0' }}>
<FormLabel htmlFor= 'isChecked' >
Receive Updates:
</FormLabel>
<Switch id= 'isChecked'
isChecked colorScheme= 'purple' />
<FormLabel htmlFor= 'isDisabled' >
Disable Notifications:
</FormLabel>
<Switch id= 'isDisabled' isDisabled
defaultChecked colorScheme= 'blue' />
<FormLabel htmlFor= 'isFocusable' >
Focusable (Disabled):
</FormLabel>
<Switch id= 'isFocusable' isFocusable
isDisabled colorScheme= 'green' />
</FormControl>
<FormControl as={Stack} spacing={4}>
<FormLabel htmlFor= 'isInvalid' >
Invalid State:
</FormLabel>
<Switch id= 'isInvalid' isInvalid
colorScheme= 'red' />
<FormLabel htmlFor= 'isReadOnly' >
Read-Only State:
</FormLabel>
<Switch id= 'isReadOnly' isReadOnly
colorScheme= 'orange' />
<FormLabel htmlFor= 'isRequired' >
Required Field:
</FormLabel>
<Switch id= 'isRequired' isRequired
colorScheme= 'teal' />
</FormControl>
</Stack>
</Box>
</ChakraProvider>
);
}
export default App;
|
Step to run the application: Run the application using the following command:
npm run start
Output: Now go to http://localhost:3000 in your browser:
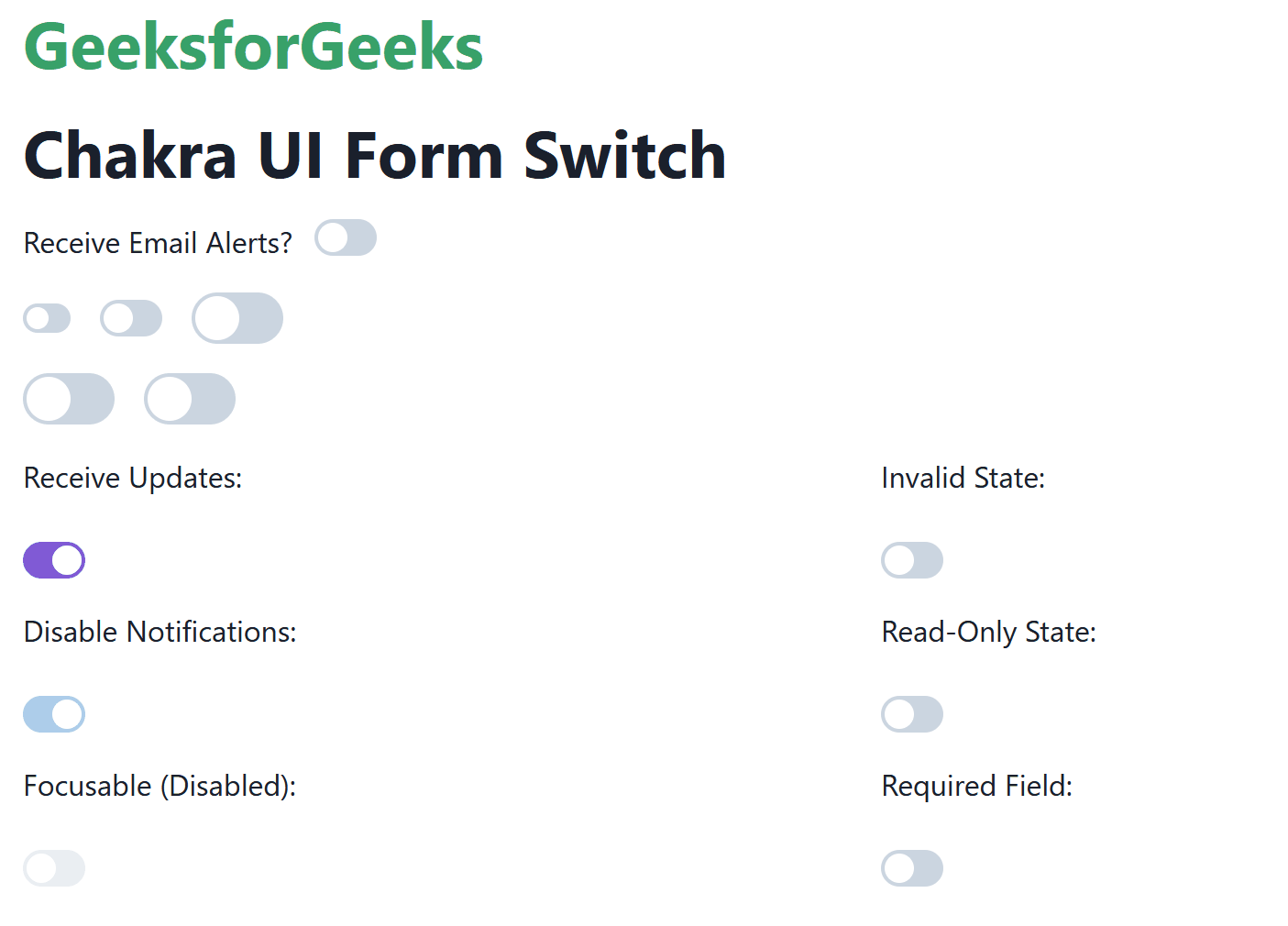
Share your thoughts in the comments
Please Login to comment...