React Chakra UI Form Number Input
Last Updated :
19 Feb, 2024
React Chakra UI Form Number Input is a powerful and user-friendly component tailored for efficient numeric data entry in web applications. Built on the Chakra UI foundation, this component seamlessly integrates into React applications, providing developers with a simple yet customizable solution for handling number input within forms. The NumberInput component is similar to the Input component, but it has controls for incrementing or decrementing numeric values. It plays a crucial role in enhancing user experience by facilitating precise and controlled numeric input.
Prerequisites:
Approach:
We will build different types of NumberInput using Chakra UI with React js. We will be using components like Input, FormLabel and FormControl and so on with different size and style properties.
Steps to Create the Project:
Step 1: Create a React application using the following command:
npx create-react-app gfg
Step 2: After creating your project folder(i.e. my-app), move to it by using the following command:
cd gfg
Step 3: After creating the React application, Install the required package using the following command:
npm i @chakra-ui/react @emotion/react@^11 @emotion/styled@^11 framer-motion@^6
Project Structure:
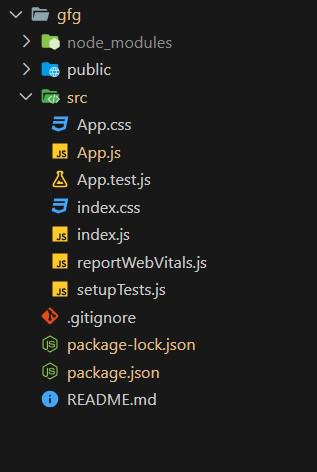
Project Structure
The updated dependencies in package.json will look like this:
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.3",
"@emotion/styled": "^11.11.0",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"framer-motion": "^11.0.3",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: Below is the basic example of the Chakra UI Form Number Input:
Javascript
import React from 'react' ;
import { ChakraProvider, Text, Box } from '@chakra-ui/react' ;
import {
NumberInput,
NumberInputField,
NumberInputStepper,
NumberIncrementStepper,
NumberDecrementStepper,
} from '@chakra-ui/react'
function App() {
return (
<ChakraProvider>
<Text color= "#2F8D46"
fontSize= "2rem"
textAlign= "center"
fontWeight= "400"
my= "1rem" >
GeeksforGeeks - React JS Chakra UI concepts
</Text>
<Box w= "55%" mx= "auto" >
<Text fontSize= "1rem"
fontWeight= "400"
my= "0.5rem" >
Common Number Input
</Text>
<NumberInput mb= "1rem" >
<NumberInputField />
<NumberInputStepper>
<NumberIncrementStepper />
<NumberDecrementStepper />
</NumberInputStepper>
</NumberInput>
<Text fontSize= "1rem"
fontWeight= "400"
my= "0.5rem" >
Setting a minimum and maximum value
</Text>
<NumberInput defaultValue={15} min={10} max={20} mb= "1rem" >
<NumberInputField />
<NumberInputStepper>
<NumberIncrementStepper />
<NumberDecrementStepper />
</NumberInputStepper>
</NumberInput>
<Text fontSize= "1rem"
fontWeight= "400"
my= "0.5rem" >
Setting the step size
</Text>
<NumberInput mb= "1rem" step={5} defaultValue={15} min={10} max={30}>
<NumberInputField />
<NumberInputStepper>
<NumberIncrementStepper />
<NumberDecrementStepper />
</NumberInputStepper>
</NumberInput>
<Text fontSize= "1rem"
fontWeight= "400"
my= "0.5rem" >
Adjusting the precision of the value
</Text>
<NumberInput mb= "1rem" defaultValue={15} precision={2} step={0.2}>
<NumberInputField />
<NumberInputStepper>
<NumberIncrementStepper />
<NumberDecrementStepper />
</NumberInputStepper>
</NumberInput>
</Box>
</ChakraProvider>
);
}
export default App;
|
Steps to run the application:
npm start
Output:
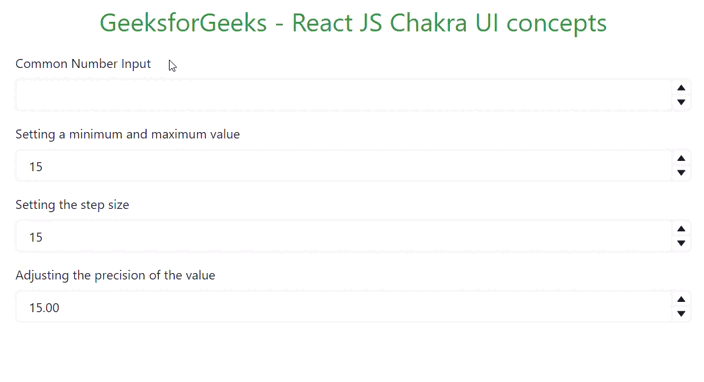
Chakra UI Form Number Input
Share your thoughts in the comments
Please Login to comment...