Chakra UI Form Control
Last Updated :
04 Feb, 2024
Chakra UI is a popular React component library that provides a set of customizable components for building user interfaces. In this article, we will talk about Chakra UI’s form control, a feature that simplifies the building and management of forms in web applications.
Prerequisites:
Below are the different approaches that we have discussed for creating Form Controls in Chakra UI.
The most basic idea to implement Chakra UI’s form involves Chakra’s components like ‘FormControl’, ‘FormLabel’, and‘Input’. These built-in components help create a simple form.
Syntax:
<FormControl>
<FormLabel>Email address</FormLabel>
<Input
type="email"
name="email"
/>
</FormControl>
You can also implement several checkboxes to select from; using the ‘Radio’ component provided; wrapped inside ‘HStack’ component. The entire things will be further wrapped inside a ‘RadioGroup’ component.
<FormControl>
<FormLabel>Favourite Tech</FormLabel>
<RadioGroup>
<HStack spacing="24px">
<Radio value="Reactjs">Reactjs</Radio>
<Radio value="Nextjs">Nextjs</Radio>
<Radio value="Expressjs">Expressjs</Radio>
</HStack>
</RadioGroup>
</FormControl>
In this approach Chakra UI helps you implement form validation. It displays a form validation message by using ‘FormErrorMessage’ component along the ‘Input’.
Syntax:
<FormControl isInvalid={isError}>
<Input type='email'/>
{!isError ? (
<></>
) : (
<FormErrorMessage>Email is required.</FormErrorMessage>
)}
</FormControl>
Chakra UI also provides input of numbers only through ‘NumberInput’ and ‘NumberInputField’. It also provides increment and decrement button through ‘NumberIncrementStepper’ and ‘NumberDecrementStepper’ wrapped inside ‘NumberInputStepper’
Syntax:
<FormControl>
<FormLabel>Amount</FormLabel>
<NumberInput max={50} min={10}>
<NumberInputField />
<NumberInputStepper>
<NumberIncrementStepper />
<NumberDecrementStepper />
</NumberInputStepper>
</NumberInput>
</FormControl>
Chakra UI also makes it easy to implement required fields using ‘FormLabel’ with an asterisk by using isRequired props.
Syntax:
<FormControl isRequired>
<FormLabel>First name</FormLabel>
<Input
name="firstName"
placeholder="First name"
/>
</FormControl>
Chakra UI also makes it easy to make a field with predefined set of options. You can use ‘Select’ component used by Chakra UI.
Syntax:
<FormControl>
<Select placeholder="Select States">
<option>Rajsthan</option>
<option>Uttrakhand</option>
</Select>
</FormControl>
Steps to Create React App and Installing Module
Step 1: Create React app by using the command provided below:
npx create-react-app my-react-app
Enter the app using the following the command:
cd my-react-app
Step 2: Install the Chakra UI dependencies by running the following command:
npm i @chakra-ui/react
Project Structure:
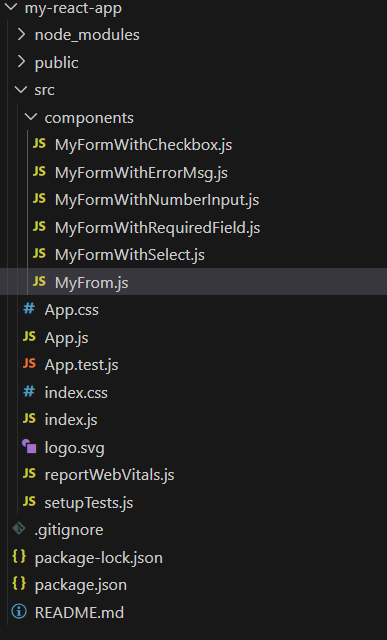
The updated dependencies should look like the one provided below. Open package.json and verify the following.
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: Below is the example to show the Form Control.
Javascript
import React, { useState } from "react" ;
import {
FormControl, FormLabel,
Input, FormHelperText
}
from "@chakra-ui/react" ;
const MyForm = () => {
const [formData, setFormData] = useState({
email: "" ,
});
const handleSubmit = (e) => {
e.preventDefault();
console.log( "Form Data:" , formData);
};
const handleInputChange = (e) => {
const { name, value } = e.target;
setFormData((prevData) => ({
...prevData,
[name]: value,
}));
};
return (
<div>
<form onSubmit={handleSubmit}>
<FormControl>
<FormLabel>
Email address
</FormLabel>
<Input
type= "email"
name= "email"
value={formData.email}
onChange={handleInputChange}
/>
<FormHelperText>
We'll never share your email.
</FormHelperText>
</FormControl>
<button className= "btn"
type= "submit" >Submit</button>
</form>
</div>
);
};
export default MyForm;
|
Javascript
import React, { useState } from "react" ;
import {
FormControl,
FormLabel,
Radio,
RadioGroup,
HStack,
} from "@chakra-ui/react" ;
const MyFormWithCheckbox = () => {
const [selectedCharacter, setSelectedCharacter] = useState( "Itachi" );
const handleSubmit = (e) => {
e.preventDefault();
console.log( "Selected Character:" ,
selectedCharacter);
};
return (
<div>
<form onSubmit={handleSubmit}>
<FormControl>
<FormLabel>Favourite Tech</FormLabel>
<RadioGroup
onChange={(value) => setSelectedCharacter(value)}
>
<HStack spacing= "24px" >
<Radio value= "Reactjs" >Reactjs</Radio>
<Radio value= "Nextjs" >Nextjs</Radio>
<Radio value= "Expressjs" >Expressjs</Radio>
</HStack>
</RadioGroup>
<button className= "btn" type= "submit" >Submit</button>
</FormControl>
</form>
</div>
);
};
export default MyFormWithCheckbox;
|
Javascript
import React, { useState } from "react" ;
import {
FormControl, FormLabel,
Input, FormErrorMessage
}
from "@chakra-ui/react" ;
function MyFormWithErrorMsg() {
const [input, setInput] = useState( '' );
const handleInputChange = (e) =>
setInput(e.target.value);
const handleSubmit = (e) => {
e.preventDefault();
console.log( "Form Data:" , { email: input });
};
const isError = input === '' ;
return (
<form onSubmit={handleSubmit}>
<FormControl isInvalid={isError}>
<FormLabel>Email</FormLabel>
<Input type= 'email' value={input}
onChange={handleInputChange} />
{!isError ? (
<></>
) : (
<FormErrorMessage>
Email is required.
</FormErrorMessage>
)}
</FormControl>
<button className= "btn" type= "submit" >Submit</button>
</form>
);
}
export default MyFormWithErrorMsg;
|
Javascript
import React, { useState } from "react" ;
import {
FormControl, FormLabel,
NumberInput,
NumberInputStepper,
NumberIncrementStepper,
NumberInputField,
NumberDecrementStepper
}
from "@chakra-ui/react" ;
const MyFormWithNumberInput = () => {
const [enteredAmount, setEnteredAmount] = useState(10);
const handleAmountChange = (value) => {
setEnteredAmount(value);
};
const handleSubmit = (e) => {
e.preventDefault();
console.log( "Entered Amount:" , enteredAmount);
};
return (
<form onSubmit={handleSubmit}>
<FormControl>
<FormLabel>Amount</FormLabel>
<NumberInput max={50} min={10}
value={enteredAmount}
onChange={handleAmountChange}>
<NumberInputField />
<NumberInputStepper>
<NumberIncrementStepper />
<NumberDecrementStepper />
</NumberInputStepper>
</NumberInput>
</FormControl>
<button type= "submit" >Submit</button>
</form>
);
};
export default MyFormWithNumberInput;
|
Javascript
import React, { useState } from "react" ;
import {
FormControl, FormLabel,
Input
}
from "@chakra-ui/react" ;
const MyFormWithRequiredField = () => {
const [formData, setFormData] = useState({
firstName: "" ,
});
const handleInputChange = (e) => {
const { name, value } = e.target;
setFormData((prevData) => ({
...prevData,
[name]: value,
}));
};
const handleSubmit = (e) => {
e.preventDefault();
console.log( "Form Data:" , formData);
};
return (
<form onSubmit={handleSubmit}>
<FormControl isRequired>
<FormLabel>First name</FormLabel>
<Input
name= "firstName"
placeholder= "First name"
value={formData.firstName}
onChange={handleInputChange}
/>
</FormControl>
<button className= "btn"
type= "submit" >Submit
</button>
</form>
);
};
export default MyFormWithRequiredField;
|
Javascript
import React, { useState } from "react" ;
import { FormControl, Select }
from "@chakra-ui/react" ;
const MyFormWithSelect = () => {
const [selectedCountry, setSelectedCountry] = useState( "" );
const handleSelectChange = (e) => {
setSelectedCountry(e.target.value);
};
const handleSubmit = (e) => {
e.preventDefault();
console.log( "Selected Country:" ,
selectedCountry);
};
return (
<form onSubmit={handleSubmit}>
<FormControl>
<Select
placeholder= "Select States"
value={selectedCountry}
onChange={handleSelectChange}
>
<option>Rajsthan</option>
<option>Uttrakhand</option>
</Select>
</FormControl>
<button className= "btn"
type= "submit" >Submit</button>
</form>
);
};
export default MyFormWithSelect;
|
Javascript
import "./App.css" ;
import MyForm
from "./components/MyForm" ;
import MyFormWithCheckbox
from "./components/MyFormWithCheckbox" ;
import MyFormWithErrorMsg
from "./components/MyFormWithErrorMsg" ;
import MyFormWithNumberInput
from "./components/MyFormWithNumberInput" ;
import MyFormWithRequiredField
from "./components/MyFormWithRequiredField" ;
import MyFormWithSelect
from "./components/MyFormWithSelect" ;
function App() {
return (
<div className= "base" >
<div className= "app" ><MyForm /></div>
<div className= "app" ><MyFormWithCheckbox /></div>
<div className= "app" ><MyFormWithErrorMsg /></div>
<div className= "app" ><MyFormWithRequiredField /></div>
<div className= "app" ><MyFormWithSelect /></div>
<div className= "app" ><MyFormWithNumberInput /></div>
</div>
);
}
export default App;
|
Javascript
import React from 'react' ;
import ReactDOM from 'react-dom/client' ;
import './index.css' ;
import App from './App' ;
import { ChakraProvider } from '@chakra-ui/react' ;
const root = ReactDOM.createRoot(document.getElementById( 'root' ));
root.render(
<ChakraProvider>
<App />
</ChakraProvider>
);
|
CSS
.base {
margin : 20px ;
}
.app {
max-width : 800px ;
margin : auto ;
padding : 20px ;
border : 2px solid gray ;
border-radius: 20px ;
margin-top : 6px ;
}
.btn {
border-radius: 10px ;
padding : 6px 12px ;
margin-top : 20px ;
background-color : skyblue;
}
.btn:hover {
background-color : white ;
color : black ;
border : 1px solid black ;
}
|
Start your application using the following command:
npm start
Output:
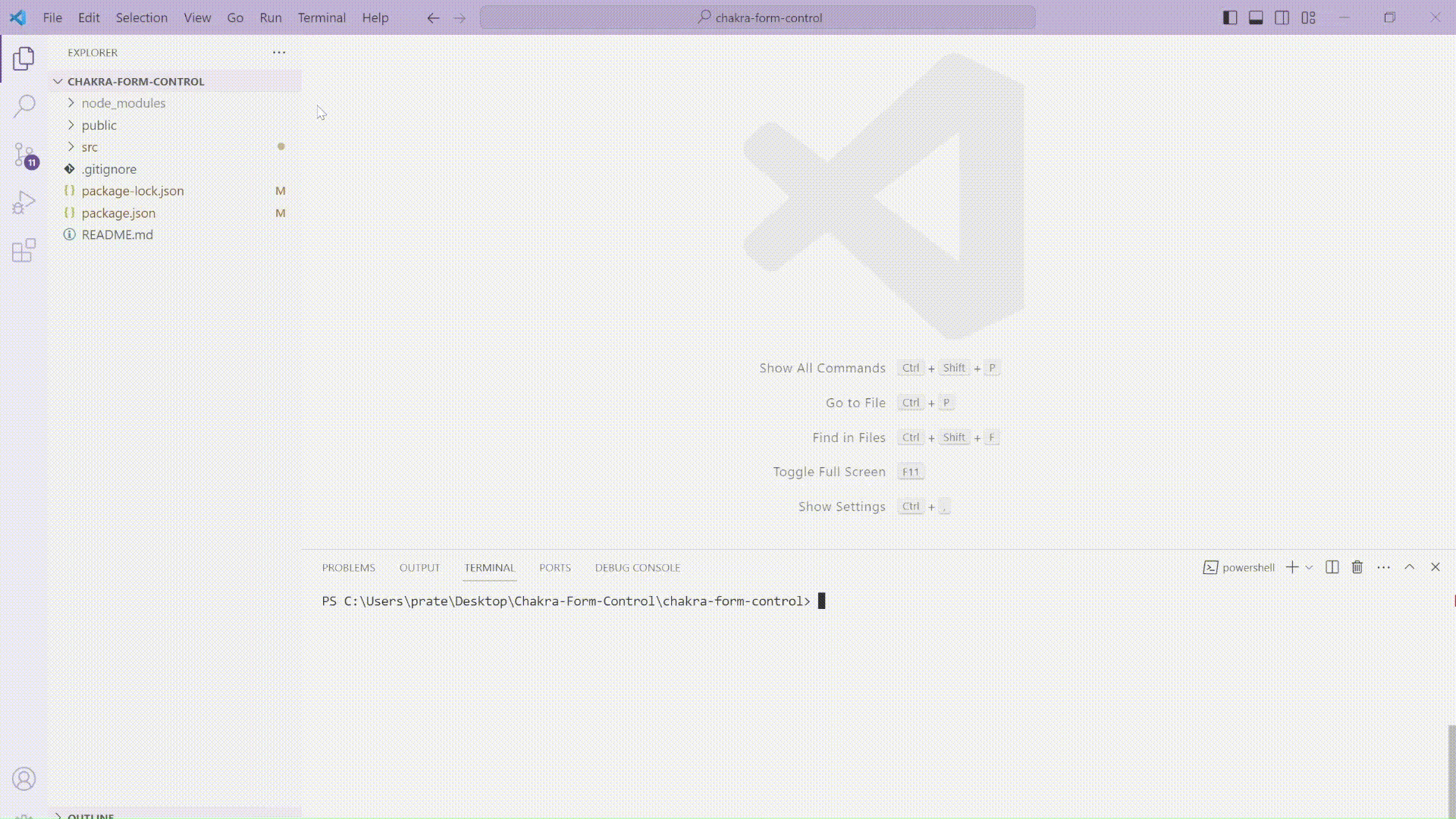
Output
Share your thoughts in the comments
Please Login to comment...