Chakra UI Form Editable
Last Updated :
04 Feb, 2024
Chakra UI Form Editable are components that look like normal form UI controls but transform into editable form control fields as soon as the user clicks or focuses on it.
It consists of 3 components to handle the functions of UI. These components are exported in the required files.
Editable
: This is the editable wrapper component of any context.
EditableInput
: This is the editable input when you click or focus on the text.
EditableTextarea
: This is the editable text area to handle multi-line text input of any context.
EditablePreview
: The is the read-only view of any given component.
Prerequisite:
Approach:
We created the basic form having one input field, a textarea and a submit button, and then we used the Editable component from the Chakra UI library and placed it for our two input fields and the submit button.The developer can add more form input controls as per the need.
Steps to Create React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app gfg-form-editable
Step 2: After creating your project folder(i.e. gfg-form-editable), move to it by using the following command:
cd gfg-form-editable
Step 3: After creating the React application, Install the required package using the following command:
npm i @chakra-ui/react @emotion/react@^11 @emotion/styled@^11 framer-motion@^6
Project Structure:
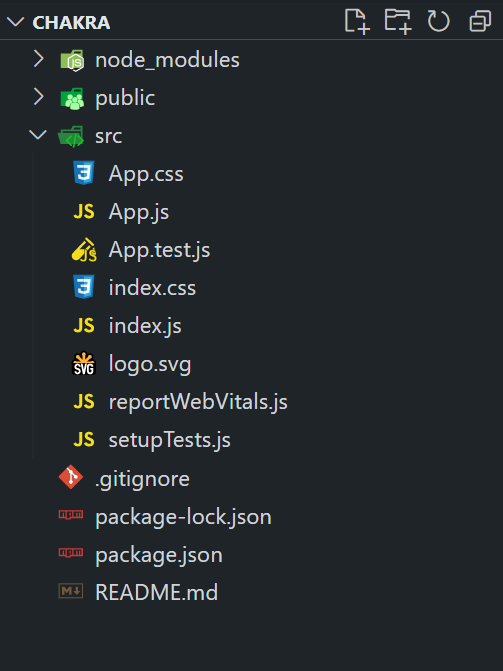
The updated dependencies in the package.json file.
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.3",
"@emotion/styled": "^11.11.0",
"framer-motion": "^6.5.1",
"react": "^18.2.0",
"react-dom": "^18.2.0"
}
Example: Write the following codes in the respective files.
Javascript
import {
ChakraProvider, Text
} from "@chakra-ui/react" ;
import Form from "./Form" ;
function App() {
return (
<ChakraProvider>
<Text
color= "#2F8D46"
fontSize= "2rem"
textAlign= "center"
fontWeight= "400"
my= "1rem" >
GeeksforGeeks -
React JS Chakra UI concepts
</Text>
<Form />
</ChakraProvider>
);
}
export default App;
|
Javascript
import React,
{ useState } from "react" ;
import {
Button, Box, Flex,
Text, Input,
Editable,
EditableInput,
EditableTextarea,
EditablePreview,
FormControl, FormLabel,
Stack, Textarea,
} from "@chakra-ui/react" ;
const Form =
() => {
const [formData, setFormData] = useState({
firstName: "" ,
});
const handleInputChange =
(field, value) => {
setFormData({
...formData,
[field]: value,
});
};
const handleSubmit =
() => {
console.log( "Form Submitted:" , formData);
alert( "Form submitted successfully!" );
setFormData({
firstName: "" ,
address: "" ,
});
};
return (
<Box bg= "lightgrey" w= "100%" p={4}>
<Flex justifyContent= "center" >
<Flex
direction= "column"
alignItems= "center"
w={
{
base: "90%" ,
md: "70%" , lg: "50%"
}
}>
<Text as= "h2" fontWeight= "bold" >
Form Editable by Chakra UI
</Text>
<Editable defaultValue= ''
textAlign= 'center'
fontSize= '2xl' >
<FormControl>
<FormLabel>
First name:
</FormLabel>
<EditablePreview />
<Input
placeholder= "First name"
onChange={
(e) =>
handleInputChange( "firstName" ,
e.target.value)} />
<EditableInput />
</FormControl>
<FormControl>
<FormLabel>Address:</FormLabel>
<Textarea
placeholder= "Enter your address"
onChange={
(e) => handleInputChange( "address" ,
e.target.value)
} />
<EditableTextarea />
</FormControl>
</Editable>
<Button
variant= "solid"
mt= "5"
colorScheme= "green"
size= "md"
onClick={handleSubmit}>
Submit form
</Button>
</Flex>
</Flex>
</Box>
);
};
export default Form;
|
Step to run the application:
npm run start
Output: Now go to http://localhost:3000 in your browser:
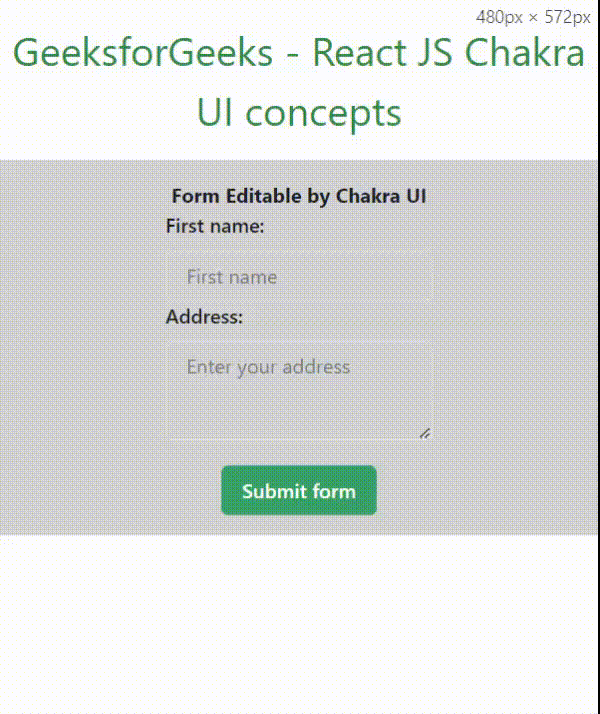
Console Output:
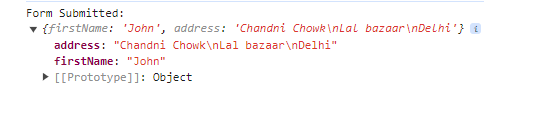
Share your thoughts in the comments
Please Login to comment...