Chakra UI Form
Last Updated :
05 Feb, 2024
Forms are an integral part of web development, enabling users to interact with websites and applications seamlessly. However, crafting forms that are both functional and aesthetically pleasing can be a daunting task. This is where Chakra UI, a popular React component library, comes into play. With its intuitive design system and comprehensive set of components, Chakra UI offers developers a hassle-free way to build elegant forms.
Prerequisites:
Approach : Using Chakra UI Form Components Directly
This approach involves utilizing Chakra UI’s built-in form components such as Input, Textarea, Select, and Checkbox to construct forms. Developers can customize these components according to their requirements using Chakra UI’s extensive styling options.
Steps to create React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app my-app
Step 2: After creating your project folder(i.e. my-app), move to it by using the following command:
cd my-app
Step 3: After creating the React application, Install the required package using the following command:
npm install @chakra-ui/react @emotion/react @emotion/styled framer-motion
Project Structure:
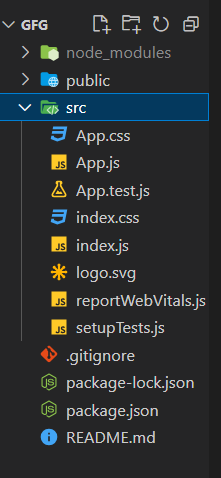
The updated depenedencies in package.json file will look like:
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.3",
"@emotion/styled": "^11.11.0",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"framer-motion": "^11.0.3",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: The example implements Form using Chakra UI in React
Javascript
import { Input, Button } from '@chakra-ui/react' ;
import './LoginForm.css' ;
function LoginForm() {
return (
<form className= "login-form" >
<Input className= "input-field"
placeholder= "Username" />
<Input className= "input-field"
type= "password" placeholder= "Password" />
<Button className= "login-button"
type= "submit" >Login</Button>
</form>
);
}
export default LoginForm;
|
CSS
.login-form {
display : flex;
flex- direction : column;
align-items: center ;
justify- content : center ;
width : 300px ;
height : 300px ;
margin : 0 auto ;
padding : 20px ;
border : 1px solid #ccc ;
border-radius: 5px ;
}
.input-field {
width : 100% ;
margin-bottom : 15px ;
padding : 10px ;
border : 1px solid #ccc ;
border-radius: 5px ;
}
.login-button {
width : 100% ;
padding : 10px ;
background-color : #4CAF50 ;
color : white ;
border : none ;
border-radius: 5px ;
cursor : pointer ;
transition: background-color 0.3 s ease;
}
.login-button:hover {
background-color : #45a049 ;
}
|
Step to run the application: Run the application using the following command:
npm run start
Output: Now go to http://localhost:3000 in your browser:
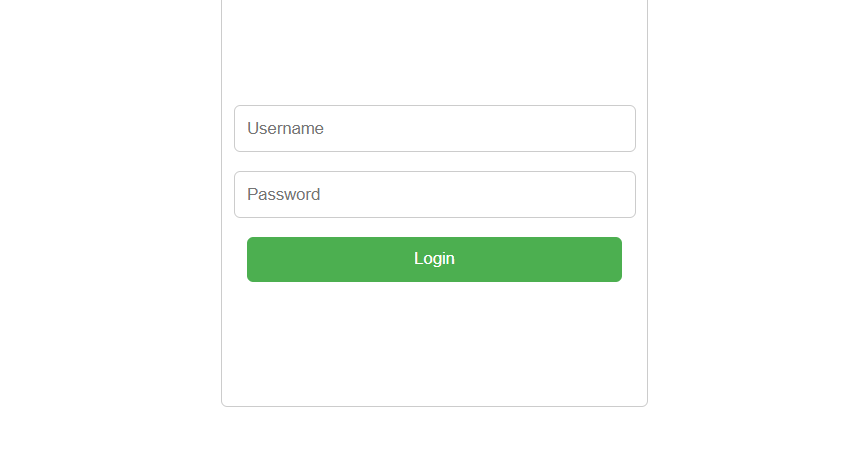
Share your thoughts in the comments
Please Login to comment...