Chakra UI Feedback Toast
Last Updated :
30 Jan, 2024
Chakra Feedback Toast allows developers to display brief, non-intrusive messages to users. The Chakra toast is designed to provide lightweight feedback about an operation or notification. For example, a toast can alert a user that their settings were saved successfully after submitting a form. Toasts will display for a few seconds and then automatically dismiss. This keeps them from blocking the user interface or disrupting workflow.
Prerequisites:
Why Use Feedback Toast?
- Non-Disruptive User Experience: Toasts provide essential information without interrupting the user’s workflow, enhancing the overall UX.
- Customizable and Flexible: Chakra UI’s Feedback Toasts can be customized in terms of duration, position, and appearance, making them versatile for various use cases.
- Accessibility-Friendly: Like other components in Chakra UI, the Feedback Toast is accessible, ensuring that it’s usable by all, including individuals with disabilities.
- Easy to Implement: Chakra UI’s Feedback Toast is straightforward to implement in React applications, requiring minimal setup.
Implementing Feedback Toast in a React Application:
Step 1: Set Up Your React Application: If you’re starting from scratch, create a new React application:
npx create-react-app my-chakra-app
cd my-chakra-app
Step 2: Install Chakra UI: Ensure that Chakra UI is installed in your project:
npm install @chakra-ui/react @emotion/react @emotion/styled framer-motion
Step 3: Setup ChakraProvider: In your index.js or App.js, wrap your application with ChakraProvider:
Javascript
import { ChakraProvider } from '@chakra-ui/react' ;
ReactDOM.render(
<ChakraProvider>
<App />
</ChakraProvider>,
document.getElementById( 'root' )
);
|
Step 4: Using Feedback Toast: In your App.js, import the useToast hook from Chakra UI:
import { useToast } from '@chakra-ui/react';
Step 5: Customize the Toast: Chakra UI’s Feedback Toast is highly customizable. You can adjust the title, description, status, duration, position, and more to fit your application’s needs.
Then, implement the Feedback Toast within a component. In this example, a button triggers a toast notification with a success message.
Javascript
import React from 'react' ;
import { Button, useToast }
from '@chakra-ui/react' ;
function App() {
const toast = useToast();
const showToast = () => {
toast({
title: "Action successful" ,
description: "Your changes have been saved." ,
status: "success" ,
duration: 5000,
isClosable: true ,
position: "bottom-left"
});
};
return (
<div style={{ padding: 20 }}>
<Button colorScheme= "blue" onClick={showToast}>
Show Toast
</Button>
</div>
);
}
export default App;
|
Output:
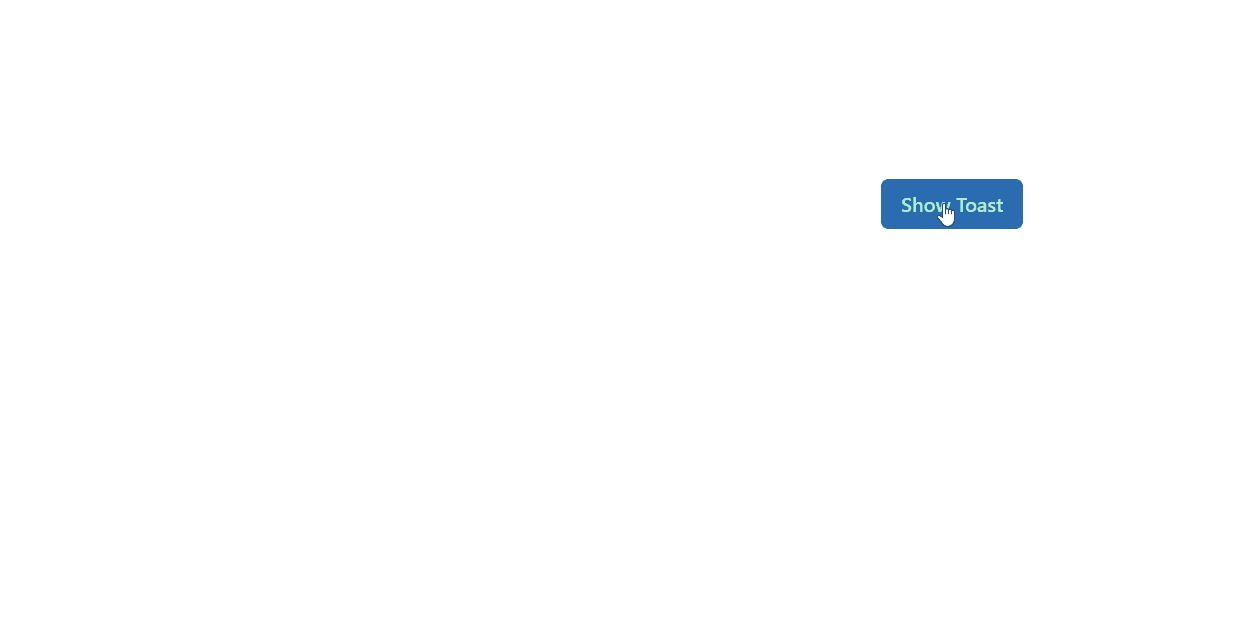
Output
Practical Use Cases:
Feedback Toasts are useful in various scenarios:
- Confirmation Messages: After a user completes an action like submitting a form or saving a document.
- Error Messages: To alert users about issues or errors without needing their immediate attention.
- System Notifications: For informing users about system updates, connectivity issues, etc.
Chakra UI’s Feedback Toast component is an essential tool for modern web applications. It balances the need for conveying important information without disrupting the user experience. With its ease of implementation, customization options, and accessibility features, the Feedback Toast is ideal for developers looking to enhance their application’s interactivity and user satisfaction. As web development continues to advance, components like the Feedback Toast will be pivotal in shaping user-friendly, engaging web applications.
Share your thoughts in the comments
Please Login to comment...