React Bootstrap Transition Utilities
Last Updated :
18 Jan, 2024
React Bootstrap Transition Utilities provide a streamlined way to incorporate transitions into your user interface. Whether you want to add a fade-in effect or smoothly animate elements, these utilities offer a convenient solution. Import the utility components, such as Fade
or Collapse
, and apply them to your elements, allowing for a visually appealing and responsive UI that engages users with smooth and polished transitions.
Collapse |
A transition component in React Bootstrap that enables the creation of a collapsible element with a smooth animation. |
Fade |
A transition component in React Bootstrap that facilitates the creation of a fading effect for elements. |
Slide |
A transition component in React Bootstrap that allows the creation of a sliding effect for elements. |
Syntax:
import { Collapse } from "react-bootstrap";
<Collapse ...>
{/* ... */}
</Collapse>
Transition API Properties
in |
Controls whether the transition is currently active or not. |
mountOnEnter |
If set to true , unmounts the component when the in prop is false. |
unmountOnExit |
If set to true , unmounts the component when the transition is complete. |
timeout |
Sets the duration of the transition. It defines the time the transition takes to complete, specified in milliseconds. |
Steps to create React Application:
Step 1: Create a React app using the following command
npx create-react-app projectname
cd projectname
Step 2 :Â After creating the React app move to our project directory using the following command
cd myapp
Step 3: Install the dependencies
npm install react-bootstrap bootstrap
Step 4: Import Bootstrap CSS in App.js file
import "bootstrap/dist/css/bootstrap.css ";
Step 5: The updated dependencies in package.json file will look like
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"bootstrap": "^5.3.2",
"react": "^18.2.0",
"react-bootstrap": "^2.10.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: This example shows implementation to collapse toggle animation to an element or component.
Javascript
import { useState } from 'react' ;
import Button from 'react-bootstrap/Button' ;
import Collapse from 'react-bootstrap/Collapse' ;
import Card from 'react-bootstrap/Card' ;
import 'bootstrap/dist/css/bootstrap.min.css' ;
function Example() {
const [open, setOpen] = useState( false );
return (
<Card className= "mx-auto mt-5" style={{ width: '20rem' }}>
<Card.Body>
<h1 className= "text-center text-success" >GeeksforGeeks</h1>
<Button
onClick={() => setOpen(!open)}
aria-controls= "example-collapse-text"
aria-expanded={open}
className= "btn-danger mx-auto d-block"
>
Click
</Button>
<Collapse in ={open}>
<div id= "example-collapse-text" >
Web development refers to the creating, building,
and maintaining of websites. It includes aspects
such as web design, web publishing, web programming,
and database management. One of the most famous stacks
used for Web Development is the MERN stack, providing
an end-to-end framework for developers to work with .
Each technology in this stack plays a significant
role in the development of web applications.
</div>
</Collapse>
</Card.Body>
</Card>
);
}
export default Example;
|
Output:
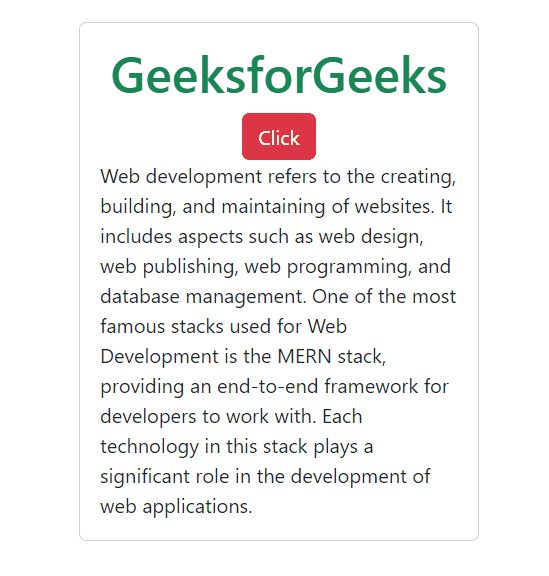
Example 2: This example shows implementation to add a fade animation to a child element or component
Javascript
import { useState } from 'react' ;
import Button from 'react-bootstrap/Button' ;
import Fade from 'react-bootstrap/Fade' ;
import { Container, Card } from 'react-bootstrap' ;
import 'bootstrap/dist/css/bootstrap.min.css' ;
function Example() {
const [open, setOpen] = useState( false );
return (
<Card className= 'mx-auto' style={{ width: "50%" }}>
<h1 className=
'text-center text-success' >
GeeksforGeeks
</h1>
<Container className= "text-center mt-5" >
<Button
onClick={() => setOpen(!open)}
aria-controls= "example-fade-text"
aria-expanded={open}
variant= "danger"
className= "mb-3" >
Toggle Text
</Button>
<Fade in ={open}>
<div id= "example-fade-text"
className= "p-3 bg-light rounded" >
Web development refers to the creating,
building, and maintaining
of websites. It includes aspects such
as web design, web publishing,
web programming, and database management.
One of the most famous stacks
used for Web Development is the MERN stack,
providing an end-to-end
framework for developers to work with .
</div>
</Fade>
</Container>
</Card>
);
}
export default Example;
|
Output:
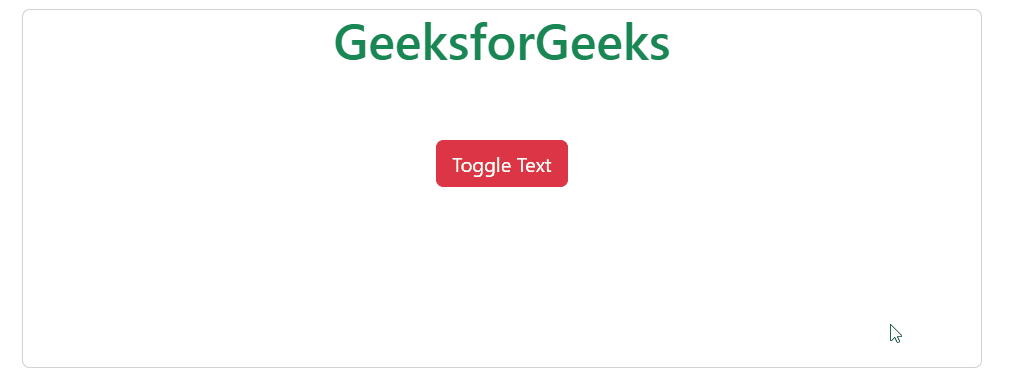
Share your thoughts in the comments
Please Login to comment...