Python | Pandas Extracting rows using .loc[]
Last Updated :
30 Sep, 2019
Python is a great language for doing data analysis, primarily because of the fantastic ecosystem of data-centric Python packages. Pandas is one of those packages and makes importing and analyzing data much easier.
Pandas provide a unique method to retrieve rows from a Data frame. DataFrame.loc[]
method is a method that takes only index labels and returns row or dataframe if the index label exists in the caller data frame.
Syntax: pandas.DataFrame.loc[]
Parameters:
Index label: String or list of string of index label of rows
Return type: Data frame or Series depending on parameters
To download the CSV used in code, click here.
Example #1: Extracting single Row
In this example, Name column is made as the index column and then two single rows are extracted one by one in the form of series using index label of rows.
import pandas as pd
data = pd.read_csv( "nba.csv" , index_col = "Name" )
first = data.loc[ "Avery Bradley" ]
second = data.loc[ "R.J. Hunter" ]
print (first, "\n\n\n" , second)
|
Output:
As shown in the output image, two series were returned since there was only one parameter both of the times.
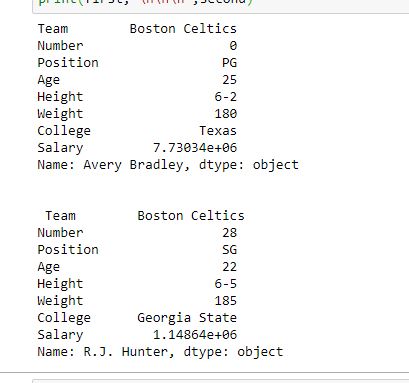
Example #2: Multiple parameters
In this example, Name column is made as the index column and then two single rows are extracted at the same time by passing a list as parameter.
import pandas as pd
data = pd.read_csv( "nba.csv" , index_col = "Name" )
rows = data.loc[[ "Avery Bradley" , "R.J. Hunter" ]]
print ( type (rows))
rows
|
Output:
As shown in the output image, this time the data type of returned value is a data frame. Both of the rows were extracted and displayed like a new data frame.
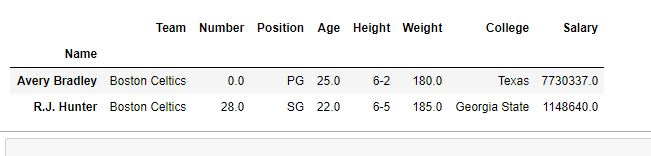
Example #3: Extracting multiple rows with same index
In this example, Team name is made as the index column and one team name is passed to .loc method to check if all values with same team name have been returned or not.
import pandas as pd
data = pd.read_csv( "nba.csv" , index_col = "Team" )
rows = data.loc[ "Utah Jazz" ]
print ( type (rows))
rows
|
Output:
As shown in the output image, All rows with team name “Utah Jazz” were returned in the form of a data frame.
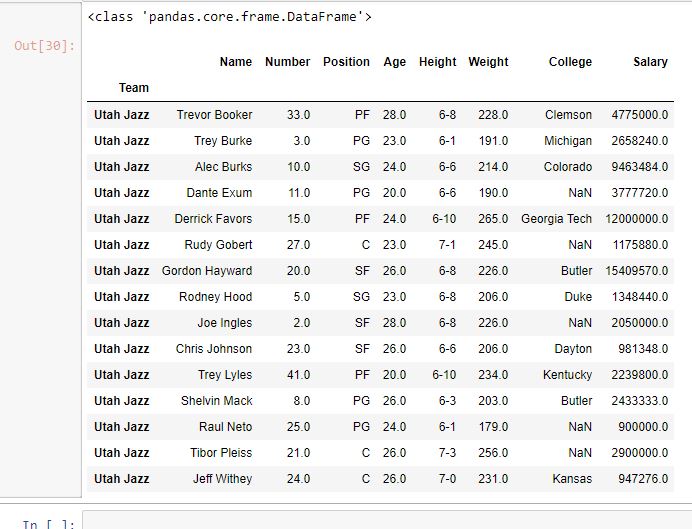
Example #4: Extracting rows between two index labels
In this example, two index label of rows are passed and all the rows that fall between those two index label have been returned (Both index labels Inclusive).
import pandas as pd
data = pd.read_csv( "nba.csv" , index_col = "Name" )
rows = data.loc[ "Avery Bradley" : "Isaiah Thomas" ]
print ( type (rows))
rows
|
Output:
As shown in the output image, all the rows that fall between passed two index labels are returned in the form of a data frame.
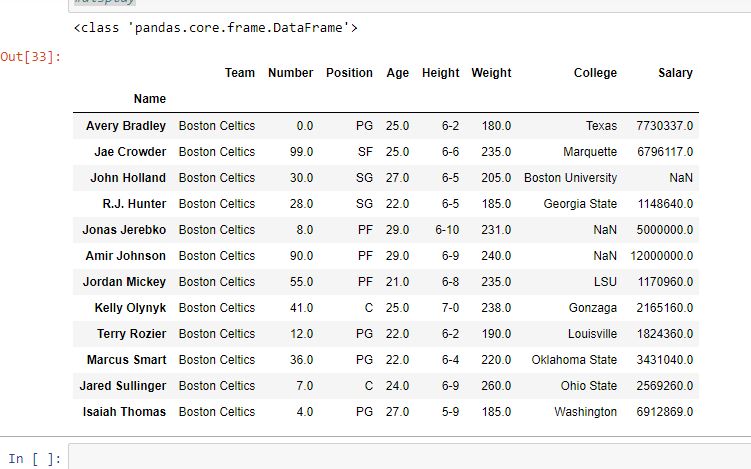
Share your thoughts in the comments
Please Login to comment...