Top Software Development Topics to prepare for Interview
Last Updated :
27 Mar, 2024
Software development refers to a set of computer science activities dedicated to the process of creating, designing, deploying, and supporting software.
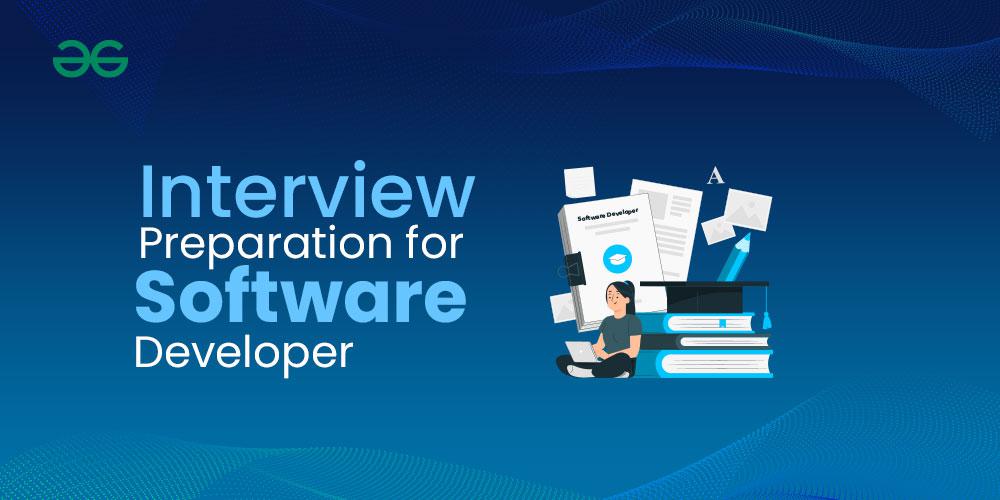
Programming language
Before attending an interview for a technical career, you do not need to know any specific programming language. However, familiarity with a major language is usually required for success. You should be familiar with the syntax of programming languages such as
You should also know some of the languages’ nuances, such as how memory management works or the most commonly used collections, libraries, etc.
The majority of software development labor requires storing and making data accessible effectively and efficiently. This requires a strong background in data structures. You’ll need to understand the inner workings of common data structures and be able to compare and contrast their use in various applications. You will be expected to know the runtimes for common operations as well as how they use memory.
For your interview, you don’t have to know all the algorithms off by heart. However, it will be much simpler to answer some of the questions if you have a solid understanding of the most popular algorithms. Consider revising common algorithms such as divide and conquer, breadth-first search vs. depth-first search, and traversals, and make sure you understand the trade-offs for each. Knowing the runtimes, theoretical limitations and basic implementation strategies of different classes of algorithms is more important than memorizing the specific details of any given algorithm.
Running Times (Oh, Omega, Theta notation) |
Link |
Bit Algorithm |
Link |
Sorting Algorithm |
Link |
Searching Algorithm |
Link |
Recursion Algorithm |
Link |
Backtracking Algorithm |
Link |
Divide and Conquer Algorithm |
Link |
Mathematical Algorithm |
Link |
Kruskal Minimum Spanning Tree (MST) |
Link |
Prim’s Minimum Spanning Tree |
Link |
Dijkstra’s Shortest Path |
Link |
Huffman Coding |
Link |
Job Sequencing Problem |
Link |
Minimum Number of Platforms Required for a Railway/Bus Station |
Link |
Longest Increasing Subsequence |
Link |
Longest Common Subsequence |
Link |
Min Cost Path |
Link |
Coin Change |
Link |
Matrix Chain Multiplication |
Link |
0-1 Knapsack Problem |
Link |
Longest Palindromic Subsequence |
Link |
Palindrome Partitioning |
Link |
KMP Algorithm |
Link |
Robin Karp Algorithm |
Link |
Aho-Corasick Algorithm |
Link |
Z Algorithm |
Link |
Bellman-Ford Algorithm |
Link |
Breadth First Search |
Link |
Depth First Search |
Link |
Floyd Warshall Algorithm |
Link |
Johnson’s Algorithm |
Link |
Karp’s minimum mean (or average) weight cycle algorithm |
Link |
Dial’s Algorithm |
Link |
Boruvka’s Algorithm |
Link |
Kahn’s Algorithm for Topological Sorting |
Link |
Karger’s Algorithm |
Link |
Tarjan’s Algorithm |
Link |
Kosaraju’s algorithm |
Link |
Nowadays System Design has become the favorite topic of interviewers. it is one of the most important concepts because if you want to create scalable and robust software you should be aware of the basic concept of system Design. it is a blueprint of software in which we deeply go through the actual requirements of the client and then deeply analyze the architecture, components, modules, interfaces, and data for a system to satisfy specified requirements. let’s discuss the High-Level Design(HLD) and Low-Level Design(LLD).
- Low-Level Design(LLD): in LLD the programmer involved in the internal implementation of software and it will create solutions for software-specific problems and designs like what kind of specific t and algorithms are would be best for the software.
- High-Level Design(HLD): in HLD the programmer deeply goes through the overall performance of the system not only the development of its specific requirements it also checks different factors like scalability, and robustness and it describes the overall description/architecture of the application.
It’s a good idea to clear the cobwebs and practice coding with a pen and paper if your skills in programming without an IDE or in a particular language are rusty. Expect to be asked to write syntactically correct code rather than pseudo code. The most important thing a software development engineer does is write scalable, robust, and well-tested code. These are the main evaluation criteria for your code. A good rule of thumb is to check for edge cases and make sure that no bad input can go through. This is your chance to show off your coding ability.
Good System design is paramount to extensible, bug-free, durable code. We know that there are nearly infinite ways to resolve any software issue, but when it comes to software extensibility and maintenance, good software development is essential. One way to build lasting software is to use object-oriented design best practices. You should have a working knowledge of a few common and useful design patterns and know how to write software in an object-oriented way. You probably won’t be asked to give detailed descriptions of how specific design patterns work, but expect to have to defend your design choices.
Topics
|
Link
|
1. What is Object Oriented Programming (OOP)? |
Link |
2. Why OOPs? |
Link |
3. What is a Class? |
Link |
4. What is an Object? |
Link |
5. What are the main features of OOPs? |
Link |
6. What is Encapsulation? |
Link |
7. What is Abstraction? |
Link |
8. What is Polymorphism? |
Link |
9. What is Inheritance? What is its purpose? |
Link |
10. What are access specifiers? What is their significance in OOPs? |
Link |
11. What are the advantages and disadvantages of OOPs? |
Link |
12. What other paradigms of programming exist besides OOPs? |
Link |
13. What is the difference between Structured Programming and Object Oriented Programming? |
Link |
14. What are some commonly used Object Oriented Programming Languages? |
Link |
15. What are the different types of Polymorphism? |
Link |
16. What is the difference between overloading and overriding? |
Link |
17. Are there any limitations on Inheritance? |
Link |
18. What different types of inheritance are there? |
Link |
19. What is an interface? |
Link |
20. How is an abstract class different from an interface? |
Link |
21. How much memory does a class occupy? |
Link |
22. Is it always necessary to create objects from class? |
Link |
23. What is the difference between a structure and a class in C++? |
Link |
24. What is a Constructor? |
Link |
25. What are the various types of constructors in C++? |
Link |
26. What is a destructor? |
Link |
27. Can we overload the constructor in a class? |
Link |
28. Can we overload the destructor in a class? |
Link |
29. What is the virtual function? |
Link |
30. What is pure virtual function? |
Link |
The vast majority of software written by Software Development Engineers (SDEs) is stored on data-storage systems. A lot of the issues faced by techs come from figuring out the best way to get and hold data for the future. You’ll need to be familiar with the general concepts of databases and their uses. The more familiar you are with the trade-offs that exist between a relational database and a non-relational database, the better off you’ll be.
Topics
|
Link
|
1. What are the advantages of DBMS over traditional file-based systems? |
Link |
2. What are super, primary, candidate, and foreign keys? |
Link |
3.What is database normalization? |
Link |
4. Why is the use of DBMS recommended? Explain by listing some of its major advantages. |
Link |
5. What are the differences between DDL, DML, and DCL in SQL? |
Link |
6. What is the difference between having and where clause? |
Link |
7. How to print duplicate rows in a table? |
Link |
8. What is Join? |
Link |
9.What is a view in SQL? How to create a view? |
Link |
10. What is the difference between Trigger and Stored Procedure? |
Link |
11. What is a transaction? What are ACID properties? |
Link |
12. What are clustered and non-clustered Indexes? |
Link |
13. What is CLAUSE in SQL? |
Link |
14. Why are cursors necessary in embedded SQL? |
Link |
15. What is the purpose of normalization in DBMS? |
Link |
16. What is the difference between a database schema and a database state? |
Link |
17. Explain the concepts of a Primary key and a Foreign Key. |
Link |
18.What are the main differences between the Primary key and the Unique Key? |
Link |
19. What is the use of the DROP command and what are the differences between DROP, TRUNCATE, and DELETE commands? |
Link |
20. Explain Entity, Entity Type, and Entity Set in DBMS. |
Link |
21. What are the different levels of abstraction in the DBMS? |
Link |
22. What is the E-R model in the DBMS? |
Link |
23. What is a functional dependency in the DBMS? |
Link |
24. What are 1NF, 2NF, 3NF, and BCNF in DBMS? |
Link |
25. What is RDBMS? |
Link |
26. What are the different types of relationships in the DBMS? |
Link |
27. What is the main goal of RAID technology? |
Link |
Systems in any scalable enterprise operate under very tight tolerances at high loads. As a result, you’ll need to be familiar with some of the basics of distributed computing. Understanding topics like service-oriented architectures (SOA), map-reduce (map reduction), distributed caching (DGC), and load balancing (LFB) will help you to address some of the more complex distributed architecture questions you may face.
You don’t need to know how to build your operating system from scratch, but you should be familiar with some OS topics that can affect code performance (e.g. memory management, processes, threads, synchronization, paging, and multithreading).
Topic
|
Link
|
1. What is a process and process table? |
Link |
2. What are the different states of the process? |
Link |
3. What is a Thread? |
Link |
4. What are the differences between process and thread? |
Link |
5. What are the benefits of multithreaded programming? |
Link |
6. What is Thrashing? |
Link |
7. What is Buffer? |
Link |
8. What is virtual memory? |
Link |
9. Explain the main purpose of an operating system. |
Link |
10. What is demand paging? |
Link |
11. What is a kernel? |
Link |
12. What are the different scheduling algorithms? |
Link |
13. What is the time-sharing system? |
Link |
14. Briefly explain FCFS. |
Link |
15. What is the RR scheduling algorithm? |
Link |
16. What is Banker’s algorithm? |
Link |
17. State the main difference between logical and physical address space. |
Link |
18. What is the basic function of paging? |
Link |
19. What is the Direct Access Method? |
Link |
20. What is caching? |
Link |
21. What is spooling? |
Link |
22. What is the functionality of an Assembler? |
Link |
23. What is GUI? |
Link |
24. What is a pipe and when is it used? |
Link |
25. What is a bootstrap program in the OS? |
Link |
26. What are the different IPC mechanisms? |
Link |
27. What is the difference between preemptive and non-preemptive scheduling? |
Link |
28. What is the difference between the Operating system and the kernel? |
Link |
60. Write the name of synchronization techniques. |
Link |
30. What is Context Switching? |
Link |
Internet topics
Every company demands that their Software development engineers understand the basics of the Internet. Learn how browsers work at the highest level. Learn about DNS lookup, TCP/IP, socket connections, etc.
You’ll likely be asked questions about data-based modeling, training/testing procedures, error checking, and statistics. For instance, you’ll be asked, “What is a problem definition for machine learning?” You’ll then be asked to define the problem as a machine learning problem and then come up with a solution. You’ll want to think about data sources, annotations, modeling techniques, and pitfalls. It’s also worth revisiting your favorite ML/AI textbooks to ensure you’re familiar with the basics of AI/ML techniques and algorithms.
Some Machine Learning Topics are mentioned below:
Topic
|
Link
|
1. What is Machine Learning? |
Link |
2. What are some real-life applications of clustering algorithms? |
Link |
3. How to choose an optimal number of clusters? |
Link |
4. What is a Hypothesis in Machine Learning? |
Link |
5. How do measure the effectiveness of the clusters? |
Link |
6. Why do we take smaller values of the learning rate? |
Link |
7. What is Overfitting in Machine Learning and how can it be avoided? |
Link |
8. Why we cannot use linear regression for a classification task? |
Link |
9. Why do we perform normalization? |
Link |
10. What are some of the hyperparameters of the random forest regressor which help to avoid overfitting? |
Link |
Conclusion
We understand this was a relatively long list of topics to review. You should bear in mind that your interviewers won’t be evaluating your ability to memorize all the details of each of these topics. They will be analyzing your ability to apply what you know and solve problems efficiently and effectively. Given that you sometimes have only limited time to prepare for a technical interview, we recommend reviewing computer science fundamentals and practicing coding outside of an integrated development environment. This will likely yield the best results for your time.
Share your thoughts in the comments
Please Login to comment...