Constructor in C++ is a special method that is invoked automatically at the time of object creation. It is used to initialize the data members of new objects generally. The constructor in C++ has the same name as the class or structure. It constructs the values i.e. provides data for the object which is why it is known as a constructor.
- Constructor is a member function of a class, whose name is the same as the class name.
- Constructor is a special type of member function that is used to initialize the data members for an object of a class automatically when an object of the same class is created.
- Constructor is invoked at the time of object creation. It constructs the values i.e. provides data for the object that is why it is known as a constructor.
- Constructors do not return value, hence they do not have a return type.
- A constructor gets called automatically when we create the object of the class.
- Constructors can be overloaded.
- A constructor can not be declared virtual.
Syntax of Constructors in C++
The prototype of the constructor looks like this:
<class-name> (list-of-parameters);
The constructor can be defined inside the class declaration or outside the class declaration
Syntax for Defining the Constructor Within the Class
<class-name> (list-of-parameters)
{
// constructor definition
}
Syntax for Defining the Constructor Outside the Class
<class-name>: :<class-name>(list-of-parameters)
{
// constructor definition
}
Examples of Constructors in C++
The below examples demonstrate how to declare constructors for a class in C++:
Example 1: Defining the Constructor Within the Class
C++
#include <iostream>
using namespace std;
class student {
int rno;
char name[50];
double fee;
public :
student()
{
cout << "Enter the RollNo:" ;
cin >> rno;
cout << "Enter the Name:" ;
cin >> name;
cout << "Enter the Fee:" ;
cin >> fee;
}
void display()
{
cout << endl << rno << "\t" << name << "\t" << fee;
}
};
int main()
{
student s;
s.display();
return 0;
}
|
Output
Enter the RollNo:121
Enter the Name:Geeks
Enter the Fee:5000
121 Geeks 5000
 Example 2: Defining the Constructor Outside the Class
C++
#include <iostream>
using namespace std;
class student {
int rno;
char name[50];
double fee;
public :
student();
void display();
};
student::student()
{
cout << "Enter the RollNo:" ;
cin >> rno;
cout << "Enter the Name:" ;
cin >> name;
cout << "Enter the Fee:" ;
cin >> fee;
}
void student::display()
{
cout << endl << rno << "\t" << name << "\t" << fee;
}
int main()
{
student s;
s.display();
return 0;
}
|
Output
Enter the RollNo:11
Enter the Name:Aman
Enter the Fee:10111
11 Aman 10111
Note: We can make the constructor defined outside the class as inline to make it equivalent to the in class definition. But note that inline is not an instruction to the compiler, it is only the request which compiler may or may not implement depending on the circumtances.
Characteristics of Constructors in C++
The following are some main characteristics of the constructors in C++:
- The name of the constructor is the same as its class name.
- Constructors are mostly declared in the public section of the class though they can be declared in the private section of the class.
- Constructors do not return values; hence they do not have a return type.
- A constructor gets called automatically when we create the object of the class.
- Constructors can be overloaded.
- A constructor can not be declared virtual.
- A constructor cannot be inherited.
- The addresses of the Constructor cannot be referred to.
- The constructor makes implicit calls to new and delete operators during memory allocation.
Types of Constructor in C++
Constructors can be classified based on in which situations they are being used. There are 4 types of constructors in C++:
- Default Constructor
- Parameterized Constructor
- Copy Constructor
- Move Constructor
Â
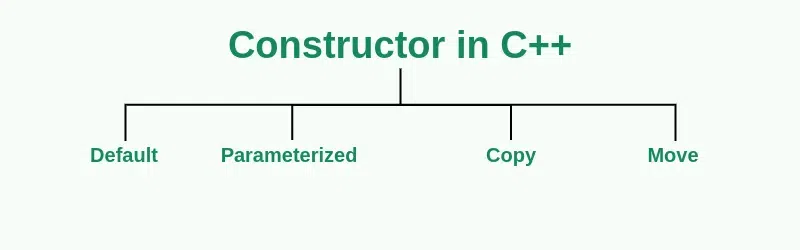
Â
Let us understand the types of constructors in C++ by taking a real-world example. Suppose you went to a shop to buy a marker. When you want to buy a marker, what are the options? The first one you go to a shop and say give me a marker. So just saying give me a marker means that you did not set which brand name and which color, you didn’t mention anything just say you want a marker. So when we said just I want a marker whatever the frequently sold marker is there in the market or his shop he will simply hand over that. And this is what a default constructor is!
The second method is you go to a shop and say I want a marker red in color and XYZ brand. So you are mentioning this and he will give you that marker. So in this case you have given the parameters. And this is what a parameterized constructor is!
Then the third one you go to a shop and say I want a marker like this(a physical marker on your hand). So the shopkeeper will see that marker. Okay, and he will give a new marker for you. So copy of that marker. And that’s what a copy constructor is!
Now, assume that you don’t to buy a new marker but instead take ownership of your friend’s marker. It means taking ownership of already present resources instead of getting a new one. That’s what a move constructor is!
1. Default Constructor in C++
A default constructor is a constructor that doesn’t take any argument. It has no parameters. It is also called a zero-argument constructor.
Syntax of Default Constructor
className() {
// body_of_constructor
}
Â
Examples of Default Constructor
The below examples demonstrate how to use the default constructors in C++.
Example 1:
CPP
#include <iostream>
using namespace std;
class construct {
public :
int a, b;
construct()
{
a = 10;
b = 20;
}
};
int main()
{
construct c;
cout << "a: " << c.a << endl << "b: " << c.b;
return 1;
}
|
Note: Even if we do not define any constructor explicitly, the compiler will automatically provide a default constructor implicitly.
Example 2:
C++
#include <iostream>
using namespace std;
class student {
int rno;
char name[50];
double fee;
public :
};
int main()
{
student s;
return 0;
}
|
Output
(no output)
As we can see, we are able the object of class student is created without passing any argument even when we haven’t defined any explicit default constructor for it.
2. Parameterized Constructor in C++
Parameterized Constructors make it possible to pass arguments to constructors. Typically, these arguments help initialize an object when it is created. To create a parameterized constructor, simply add parameters to it the way you would to any other function. When you define the constructor’s body, use the parameters to initialize the object.
Syntax of Parameterized Constructor
className (parameters...) {
// body
}
Examples of Parameterized Constructor
The below examples demonstrate how to use the parameterized constructors in C++.
Example 1: Defining Parameterized Constructor Inside the Class.
CPP
#include <iostream>
using namespace std;
class Point {
private :
int x, y;
public :
Point( int x1, int y1)
{
x = x1;
y = y1;
}
int getX() { return x; }
int getY() { return y; }
};
int main()
{
Point p1(10, 15);
cout << "p1.x = " << p1.getX()
<< ", p1.y = " << p1.getY();
return 0;
}
|
Output
p1.x = 10, p1.y = 15
Example 2: Defining Parameterized Constructor Outside the Class.
C++
#include <iostream>
#include <string.h>
using namespace std;
class student {
int rno;
char name[50];
double fee;
public :
student( int , char [], double );
void display();
};
student::student( int no, char n[], double f)
{
rno = no;
strcpy (name, n);
fee = f;
}
void student::display()
{
cout << endl << rno << "\t" << name << "\t" << fee;
}
int main()
{
student s(1001, "Ram" , 10000);
s.display();
return 0;
}
|
When an object is declared in a parameterized constructor, the initial values have to be passed as arguments to the constructor function. The normal way of object declaration may not work. The parameterized constructors can be called explicitly or implicitly:
Example e = Example(0, 50); // Explicit call
Example e(0, 50); // Implicit call
When the parameterized constructor is defined and no default constructor is defined explicitly, the compiler will not implicitly create the default constructor and hence create a simple object as:
Student s;
will flash an error.
Example 3:
C++
#include <iostream>
#include <string.h>
using namespace std;
class student {
int rno;
char name[50];
double fee;
public :
student( int no, char n[], double f)
{
rno = no;
strcpy (name, n);
fee = f;
}
};
int main()
{
student s;
return 0;
}
|
Output
main.cpp: In function ‘int main()’:
main.cpp:25:13: error: no matching function for call to ‘student::student()’
25 | student s; // this will cause error
| ^
main.cpp:14:5: note: candidate: ‘student::student(int, char*, double)’
14 | student(int no, char n[], double f)
| ^~~~~~~
main.cpp:14:5: note: candidate expects 3 arguments, 0 provided
main.cpp:8:7: note: candidate: ‘constexpr student::student(const student&)’
8 | class student {
| ^~~~~~~
main.cpp:8:7: note: candidate expects 1 argument, 0 provided
main.cpp:8:7: note: candidate: ‘constexpr student::student(student&&)’
main.cpp:8:7: note: candidate expects 1 argument, 0 provided ^~
Important Note: Whenever we define one or more non-default constructors( with parameters ) for a class, a default constructor( without parameters ) should also be explicitly defined as the compiler will not provide a default constructor in this case. However, it is not necessary but it’s considered to be the best practice to always define a default constructor.
Uses of Parameterized Constructor
- It is used to initialize the various data elements of different objects with different values when they are created.
- It is used to overload constructors.
Default Arguments with C++ Parameterized Constructor
Just like normal functions, we can also define default values for the arguments of parameterized constructors. All the rules of the default arguments will be applied to these parameters.
Example 3: Defining Parameterized Constructor with Default Values
C++
#include <iostream>
using namespace std;
class GFG {
private :
int data;
public :
GFG( int x = 0) { data = x; }
int getData() { return data; }
};
int main()
{
GFG obj1;
GFG obj2(25);
cout << "First Object Data: " << obj1.getData() << endl;
cout << "Second Object Data: " << obj2.getData()
<< endl;
return 0;
}
|
Output
First Object Data: 0
Second Object Data: 25
As we can see, when the default values are assigned to every argument of the parameterized constructor, it is legal to create the object without passing any parameters just like default constructors. So, this type of constructor works as both a default and parameterized constructor.
3. Copy Constructor in C++
A copy constructor is a member function that initializes an object using another object of the same class.
Syntax of Copy Constructor
Copy constructor takes a reference to an object of the same class as an argument.
ClassName (ClassName &obj)
{
// body_containing_logic
}
Just like the default constructor, the C++ compiler also provides an implicit copy constructor if the explicit copy constructor definition is not present. Here, it is to be noted that, unlike the default constructor where the presence of any type of explicit constructor results in the deletion of the implicit default constructor, the implicit copy constructor will always be created by the compiler if there is no explicit copy constructor or explicit move constructor is present.
Examples of Copy Constructor
The below examples demonstrate how to use the copy constructors in C++.
Example 1: Illustration of Implicit Copy Constructor
C++
#include <iostream>
using namespace std;
class Sample {
int id;
public :
Sample( int x) { id = x; }
void display() { cout << "ID=" << id; }
};
int main()
{
Sample obj1(10);
obj1.display();
cout << endl;
Sample obj2(obj1);
obj2.display();
return 0;
}
|
Example 2: Defining of Explicit Copy Constructor
C++
#include <iostream>
using namespace std;
class Sample {
int id;
public :
Sample() {}
Sample( int x) { id = x; }
Sample(Sample& t) { id = t.id; }
void display() { cout << "ID=" << id; }
};
int main()
{
Sample obj1(10);
obj1.display();
cout << endl;
Sample obj2(obj1);
obj2.display();
return 0;
}
|
Example 3: Defining of Explicit Copy Constructor with Parameterized Constructor
C++
#include <iostream>
#include <string.h>
using namespace std;
class student {
int rno;
char name[50];
double fee;
public :
student( int , char [], double );
student(student& t)
{
rno = t.rno;
strcpy (name, t.name);
fee = t.fee;
}
void display();
};
student::student( int no, char n[], double f)
{
rno = no;
strcpy (name, n);
fee = f;
}
void student::display()
{
cout << endl << rno << "\t" << name << "\t" << fee;
}
int main()
{
student s(1001, "Manjeet" , 10000);
s.display();
student manjeet(s);
manjeet.display();
return 0;
}
|
Output
1001 Manjeet 10000
1001 Manjeet 10000
Uses of Copy Constructor
- Constructs a new object by copying values from an existing object.
- Can be used to perform deep copy.
- Modify specific attributes during the copy process if needed.
4. Move Constructor in C++
The move constructor is a recent addition to the family of constructors in C++. It is like a copy constructor that constructs the object from the already existing objects., but instead of copying the object in the new memory, it makes use of move semantics to transfer the ownership of the already created object to the new object without creating extra copies.
It can be seen as stealing the resources from other objects.
Syntax of Move Constructor in C++
className (className&& obj) {
// body of the constructor
}
The move constructor takes the rvalue reference of the object of the same class and transfers the ownership of this object to the newly created object.
Like a copy constructor, the compiler will create a move constructor for each class that does not have any explicit move constructor.
Examples of Move Constructor
The below examples demonstrate how to use the move constructors in C++.
Example 1: Defining Move Constructor
C++
#include <iostream>
using namespace std;
class Box {
public :
int * data;
Box( int value)
{
data = new int ;
*data = value;
}
Box(Box&& other) noexcept
{
cout << "Move Constructor Called" << endl;
data = other.data;
other.data = nullptr;
}
~Box() { delete data; }
};
int main()
{
Box originalBox(42);
Box newBox(move(originalBox));
cout << "newBox.data: " << *newBox.data;
return 0;
}
|
Output
Move Constructor Called
newBox.data: 42
Uses of Move Constructor
- Instead of making copies, move constructors efficiently transfer ownership of these resources.
- This prevents unnecessary memory copying and reduces overhead.
- You can define your own move constructor to handle specific resource transfers.
Destructors in C++
A destructor is also a special member function as a constructor. Destructor destroys the class objects created by the constructor. Destructor has the same name as their class name preceded by a tilde (~) symbol. It is not possible to define more than one destructor. The destructor is only one way to destroy the object created by the constructor. Hence destructor can-not be overloaded. Destructor neither requires any argument nor returns any value. It is automatically called when the object goes out of scope. Â Destructors release memory space occupied by the objects created by the constructor. In destructor, objects are destroyed in the reverse of object creation.
Syntax of Destructors in C++
Like constructors, destructors can also be defined either inside or outside of the class.
The syntax for defining the destructor within the class
~ <class-name>(){}
The syntax for defining the destructor outside the class
<class-name>: : ~<class-name>(){}
Examples of Destructors in C++
The below examples demonstrate how to use the destructors in C++.
Example 1: Defining a Simple Destructor
C++
#include <iostream>
using namespace std;
class Test {
public :
Test() { cout << "\n Constructor executed" ; }
~Test() { cout << "\n Destructor executed" ; }
};
main()
{
Test t;
return 0;
}
|
Output
Constructor executed
Destructor executed
Example 2: Counting the Number of Times Object is Created and Destroyed
C++
#include <iostream>
using namespace std;
int count = 0;
class Test {
public :
Test()
{
count++;
cout << "No. of Object created: " << count << endl;
}
~Test()
{
cout << "No. of Object destroyed: " << count
<< endl;
--count;
}
};
int main()
{
Test t, t1, t2, t3;
return 0;
}
|
Output
No. of Object created: 1
No. of Object created: 2
No. of Object created: 3
No. of Object created: 4
No. of Object destroyed: 4
No. of Object destroyed: 3
No. of Object destroyed: 2
No. of Object destroyed: 1
Characteristics of Destructors in C++
The following are some main characteristics of destructors in C++:
- Destructor is invoked automatically by the compiler when its corresponding constructor goes out of scope and releases the memory space that is no longer required by the program.
- Destructor neither requires any argument nor returns any value therefore it cannot be overloaded.
- Destructor  cannot be declared as static and const;
- Destructor should be declared in the public section of the program.
- Destructor is called in the reverse order of its constructor invocation.
Frequently Asked Questions on C++ Constructors
What Are the Functions That Are Generated by the Compiler by Default, If We Do Not Provide Them Explicitly?
The functions that are generated by the compiler by default if we do not provide them explicitly are:
- Default Constructor
- Copy Constructor
- Move Constructors
- Assignment Operator
- Destructor
Can We Make the Constructors Private?
Yes, in C++, constructors can be made private. This means that no external code can directly create an object of that class.
How Constructors Are Different from a Normal Member Function?
A constructor is different from normal functions in following ways:Â
- Constructor has same name as the class itself
- Default Constructors don’t have input argument however, Copy and Parameterized Constructors have input arguments
- Constructors don’t have return type
- A constructor is automatically called when an object is created.
- It must be placed in public section of class.
- If we do not specify a constructor, C++ compiler generates a default constructor for object (expects no parameters and has an empty body).
Can We Have More Than One Constructor in a Class?
Yes, we can have more than one constructor in a class. It is called Constructor Overloading.
Related Articles:
Â
Share your thoughts in the comments
Please Login to comment...