How to install React-Bootstrap in React Application ?
Last Updated :
15 Mar, 2024
React Bootstrap is a popular library that let us use the Bootstrap framework’s power and flexibility to React.js applications. By combining the flexibility of React with the UI components provided by Bootstrap, you can create responsive and visually appealing user interfaces with ease.
In this article, we’ll see the process of installing React Bootstrap in a React.js application.
What is React Bootstrap?
React Bootstrap is a replacement of Bootstrap JavaScript which effectively embeds Bootstrap components into a React Application without the need of external libraries like JQuery. Each component of React Bootstrap is a true React Component and this module is one of the oldest React libraries which keeps evolving with time and helps to create an excellent UI.
Syntax:
import { Container } from "react-bootstrap";
<Container>
...component codes
</Container>
React Bootstrap Installation
Step 1: Go to the official website of React bootstrap to get the installation commands and CDN links.
Step 2: In your existing React project install react bootstrap using the following command.
npm install react-bootstrap bootstrap
Step 3: If you also want to use normal Bootstrap along with React Bootstrap you can add these CDN links in your public/index.html file.
<script src=”https://cdn.jsdelivr.net/npm/react/umd/react.production.min.js” crossorigin></script>
<script
src=”https://cdn.jsdelivr.net/npm/react-dom/umd/react-dom.production.min.js”
crossorigin></script>
<script
src=”https://cdn.jsdelivr.net/npm/react-bootstrap@next/dist/react-bootstrap.min.js”
crossorigin></script>
<link
rel=”stylesheet”
href=”https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/css/bootstrap.min.css”
integrity=”sha384-T3c6CoIi6uLrA9TneNEoa7RxnatzjcDSCmG1MXxSR1GAsXEV/Dwwykc2MPK8M2HN”
crossorigin=”anonymous”
/>
Step 4: To use the React Bootstrap add the following import in either index.js or App.js in src folder.
import 'bootstrap/dist/css/bootstrap.min.css';
Now you are ready to use React Bootstarp in your React project.
Use React Bootstrap in Project:
Step 1: Create a React Application using the below command.
npx create-react-app app-name
cd app-name
Step 2: Now in the application, you need to install the below library.
npm install react-bootstrap bootstrap
Now we will able to use features of React Bootstrap.
The updated Dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"bootstrap": "^5.3.3",
"react": "^18.2.0",
"react-bootstrap": "^2.10.1",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: Create the required files as shown in folder structure and add the following codes.
Javascript
//App.js
import "./App.css";
import { Container, Row, Col, Card } from "react-bootstrap";
import { Post } from "./Post";
function App() {
return (
<div className="App">
<Container fluid>
<Container>
<Row>
{Post.map((v, i) => {
return <Cardd pitems={v} key={i} />;
})}
</Row>
</Container>
</Container>
</div>
);
}
export default App;
function Cardd({ pitems }) {
if (!pitems) {
return null;
}
return (
<div className="col-sm-3 my-3">
<Col>
<Card style={{ width: "18rem", height: "15rem"}}>
<Card.Body>
<Card.Title>{pitems.name}</Card.Title>
<Card.Subtitle className="mb-2 text-muted">
{pitems.email}
</Card.Subtitle>
<Card.Text>{pitems.body}</Card.Text>
<Card.Link>{pitems.Product}</Card.Link>
</Card.Body>
</Card>
</Col>
</div>
);
}
Javascript
//Post.js
export let Post = [
{
postId: 1,
id: 1,
name: "ReactJS",
email: "Eliseo@gardner.biz",
body: `React is one of the most popular JavaScript
libraries for building dynamic user interfaces.
Whether you are a beginner or an experienced
developer, React Tutorial will significantly enhance
your development skills.`,
},
{
postId: 2,
id: 2,
name: "AngularJS",
email: "Jayne_Kuhic@sydney.com",
body: `Angular is a popular open-source Typescript
framework created by Google for developing web
applications. Front-end developers use
frameworks like Angular or React to present
and manipulate data efficiently and dynamically.`,
},
{
postId: 3,
id: 3,
name: "NodeJS",
email: "Nikita@garfield.biz",
body: `NodeJS is a JavaScript-based server-side
runtime environment. It is developed by
Ryan Dahi in the year 2009 and v20.9 is the
latest version of Node.js.`,
},
{
postId: 4,
id: 4,
name: "ExpressJS",
email: "Lew@alysha.tv",
body: "Express makes the development of
Node application very easy and it is very
simple to use.It provides a simple and
efficient way to build web applications
and APIs using JavaScript.`,
},
{
postId: 5,
id: 1,
name: "MongoDB",
email: "Eliseo@gardner.biz",
body: `MongoDB, the most popular NoSQL database,
is an open-source document-oriented database.
The term ‘NoSQL’ means ‘non-relational’.`,
},
{
postId: 6,
id: 2,
name: "Mongoose",
email: "Jayne_Kuhic@sydney.com",
body: `Mongoose is an Object Data Modeling (ODM)
library for MongoDB. MongoDB is a NoSQL database
and Mongoose is used to interact with MongoDB
by providing a schema-based solution.`,
},
];
To start the application run the following command.
npm start
Output:
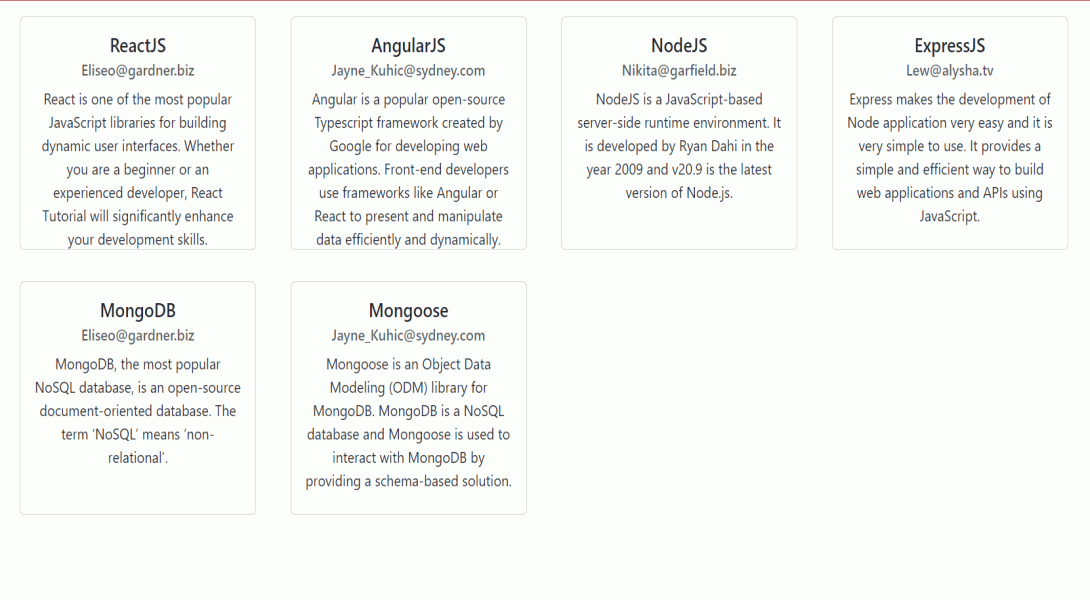
How to install React Bootstrap in react.js Application
Share your thoughts in the comments
Please Login to comment...