R Vectors
Last Updated :
20 Dec, 2023
R Vectors are the same as the arrays in R language which are used to hold multiple data values of the same type. One major key point is that in R Programming Language the indexing of the vector will start from ‘1’ and not from ‘0’. We can create numeric vectors and character vectors as well.Â
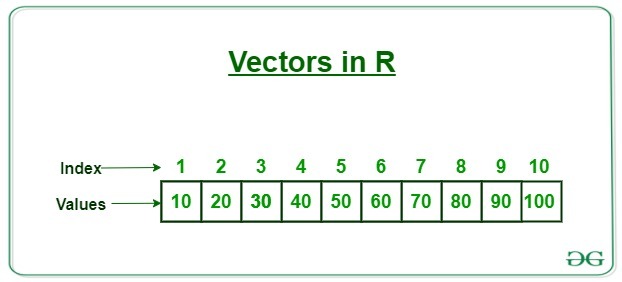
R – Vector
Creating a vector
A vector is a basic data structure that represents a one-dimensional array. to create a array we use the “c” function which the most common method use in R Programming Language.
R
X<- c (61, 4, 21, 67, 89, 2)
cat ( 'using c function' , X, '\n' )
Y<- seq (1, 10, length.out = 5)
cat ( 'using seq() function' , Y, '\n' )
Z<- 2:7
cat ( 'using colon' , Z)
|
Output:
using c function 61 4 21 67 89 2
using seq() function 1 3.25 5.5 7.75 10
using colon 2 3 4 5 6 7
Types of R vectors
Vectors are of different types which are used in R. Following are some of the types of vectors:
Numeric vectors
Numeric vectors are those which contain numeric values such as integer, float, etc.Â
R
v1<- c (4, 5, 6, 7)
typeof (v1)
v2<- c (1L, 4L, 2L, 5L)
typeof (v2)
|
Output:
[1] "double"
[1] "integer"
Character vectors
Character vectors in R contain alphanumeric values and special characters.Â
R
v1<- c ( 'geeks' , '2' , 'hello' , 57)
typeof (v1)
|
Output:
[1] "character"
Logical vectors
Logical vectors in R contain Boolean values such as TRUE, FALSE and NA for Null values.Â
R
v1<- c ( TRUE , FALSE , TRUE , NA )
typeof (v1)
|
Output:
[1] "logical"
Length of R vector
In R, the length of a vector is determined by the number of elements it contains. we can use the length()
function to retrieve the length of a vector.
R
x <- c (1, 2, 3, 4, 5)
length (x)
y <- c ( "apple" , "banana" , "cherry" )
length (y)
z <- c ( TRUE , FALSE , TRUE , TRUE )
length (z)
|
Output:
> length(x)
[1] 5
> length(y)
[1] 3
> length(z)
[1] 4
Accessing R vector elements
Accessing elements in a vector is the process of performing operation on an individual element of a vector. There are many ways through which we can access the elements of the vector. The most common is using the ‘[]’, symbol.
Note: Vectors in R are 1 based indexing unlike the normal C, python, etc format.
R
X<- c (2, 5, 18, 1, 12)
cat ( 'Using Subscript operator' , X[2], '\n' )
Y<- c (4, 8, 2, 1, 17)
cat ( 'Using combine() function' , Y[ c (4, 1)], '\n' )
|
Output:
Using Subscript operator 5
Using combine() function 1 4
Modifying a R vector
Modification of a Vector is the process of applying some operation on an individual element of a vector to change its value in the vector. There are different ways through which we can modify a vector:Â
R
X<- c (2, 7, 9, 7, 8, 2)
X[3] <- 1
X[2] <-9
cat ( 'subscript operator' , X, '\n' )
X[1:5]<- 0
cat ( 'Logical indexing' , X, '\n' )
X<- X[ c (3, 2, 1)]
cat ( 'combine() function' , X)
|
Output:
subscript operator 2 9 1 7 8 2
Logical indexing 0 0 0 0 0 2
combine() function 0 0 0
Deleting a R vector
Deletion of a Vector is the process of deleting all of the elements of the vector. This can be done by assigning it to a NULL value.Â
R
M<- c (8, 10, 2, 5)
M<- NULL
cat ( 'Output vector' , M)
|
Output:
Output vector NULL
Sorting elements of a R Vector
sort() function is used with the help of which we can sort the values in ascending or descending order.Â
R
X<- c (8, 2, 7, 1, 11, 2)
A<- sort (X)
cat ( 'ascending order' , A, '\n' )
B<- sort (X, decreasing = TRUE )
cat ( 'descending order' , B)
|
Output:
ascending order 1 2 2 7 8 11
descending order 11 8 7 2 2 1
Share your thoughts in the comments
Please Login to comment...