Python OpenCV | cv2.putText() method
Last Updated :
04 Jan, 2023
OpenCV-Python is a library of Python bindings designed to solve computer vision problems. cv2.putText()
method is used to draw a text string on any image.
Syntax: cv2.putText(image, text, org, font, fontScale, color[, thickness[, lineType[, bottomLeftOrigin]]])
Parameters:
image: It is the image on which text is to be drawn.
text: Text string to be drawn.
org: It is the coordinates of the bottom-left corner of the text string in the image. The coordinates are represented as tuples of two values i.e. (X coordinate value, Y coordinate value).
font: It denotes the font type. Some of font types are FONT_HERSHEY_SIMPLEX, FONT_HERSHEY_PLAIN, , etc.
fontScale: Font scale factor that is multiplied by the font-specific base size.
color: It is the color of text string to be drawn. For BGR, we pass a tuple. eg: (255, 0, 0) for blue color.
thickness: It is the thickness of the line in px.
lineType: This is an optional parameter.It gives the type of the line to be used.
bottomLeftOrigin: This is an optional parameter. When it is true, the image data origin is at the bottom-left corner. Otherwise, it is at the top-left corner.
Return Value: It returns an image.
Image used for all the below examples:
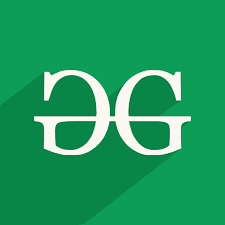
Example #1:
import cv2
path = r 'C:\Users\Rajnish\Desktop\geeksforgeeks\geeks.png'
image = cv2.imread(path)
window_name = 'Image'
font = cv2.FONT_HERSHEY_SIMPLEX
org = ( 50 , 50 )
fontScale = 1
color = ( 255 , 0 , 0 )
thickness = 2
image = cv2.putText(image, 'OpenCV' , org, font,
fontScale, color, thickness, cv2.LINE_AA)
cv2.imshow(window_name, image)
|
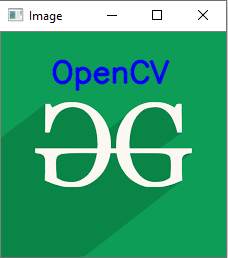
Example #2:
import cv2
path = r 'C:\Users\Rajnish\Desktop\geeksforgeeks\geeks.png'
image = cv2.imread(path)
window_name = 'Image'
text = 'GeeksforGeeks'
font = cv2.FONT_HERSHEY_SIMPLEX
org = ( 00 , 185 )
fontScale = 1
color = ( 0 , 0 , 255 )
thickness = 2
image = cv2.putText(image, text, org, font, fontScale,
color, thickness, cv2.LINE_AA, False )
image = cv2.putText(image, text, org, font, fontScale,
color, thickness, cv2.LINE_AA, True )
cv2.imshow(window_name, image)
|
Output:
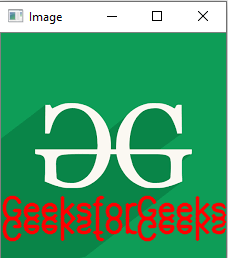
Share your thoughts in the comments
Please Login to comment...