Python OpenCV | cv2.imread() method
Last Updated :
30 May, 2023
OpenCV-Python is a library of Python bindings designed to solve computer vision problems. cv2.imread() method loads an image from the specified file. If the image cannot be read (because of the missing file, improper permissions, or unsupported or invalid format) then this method returns an empty matrix.
Example:
Python3
import cv2
image = cv2.imread( "jg.png" )
cv2.imshow( "Image" , image)
cv2.waitKey( 0 )
cv2.destroyAllWindows()
|
Output:
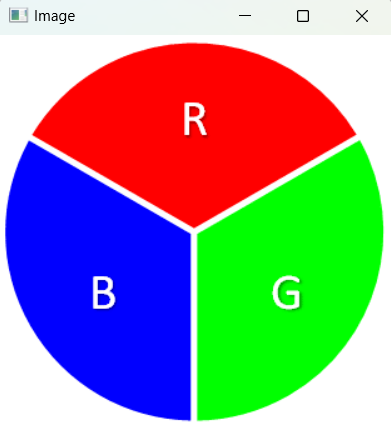
Â
Syntax of OpenCV cv2.imread() Method
Syntax: cv2.imread(filename, flag)Â
Parameters:
- filename: The path to the image file.
- flag: The flag specifies the way how the image should be read.
- cv2.IMREAD_COLOR – It specifies to load a color image. Any transparency of image will be neglected. It is the default flag. Alternatively, we can pass integer value 1 for this flag.
- cv2.IMREAD_GRAYSCALE – It specifies to load an image in grayscale mode. Alternatively, we can pass integer value 0 for this flag.Â
- cv2.IMREAD_UNCHANGED – It specifies to load an image as such including alpha channel. Alternatively, we can pass integer value -1 for this flag.
Return Value:
The cv2.imread() function return a NumPy array if the image is loaded successfully.
Examples of OpenCV cv2.imread() MethodÂ
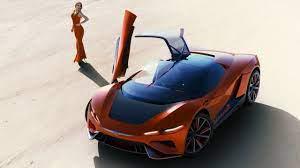
Input Image
Python OpenCV Read Image – cv2 imread()
In this example, we are reading the image as a color image.
Python3
import cv2
image = cv2.imread( "gfg.jpeg" )
cv2.imshow( "Image" , image)
cv2.waitKey( 0 )
cv2.destroyAllWindows()
|
Output:
Â
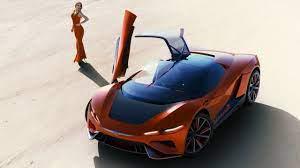
image
Â
Python OpenCV Read image grayscale
In this example, we are reading the image as a greyscale image. Both color and grayscale images are acceptable as input.
Python3
import cv2
image = cv2.imread( "gfg.jpeg" ,cv2.IMREAD_GRAYSCALE)
cv2.imshow( "Image" , image)
cv2.waitKey( 0 )
cv2.destroyAllWindows()
|
Output:
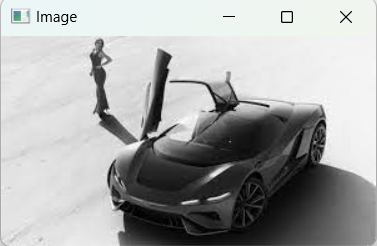
Image
Python OpenCV | Read PNG Image with Transparency
In this example, we are reading the image with the transparency channel.
Python3
import cv2
image = cv2.imread( "gfg.jpeg" ,cv2.IMREAD_UNCHANGED)
cv2.imshow( "Image" , image)
cv2.waitKey( 0 )
cv2.destroyAllWindows()
|
Output:
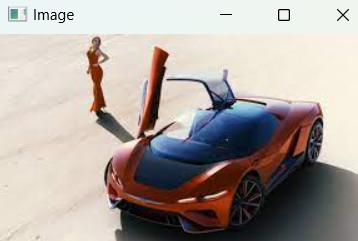
image
imread() and Color Channels
A NumPy array is produced after reading an image file with the cv2.imread() method. By default, the image is saved in the BGR color space. As a result, the blue, green, and red color channels, respectively, correspond to the first three channels of the NumPy array.
r1 = image[:,:,0] # get blue channel
g1 = image[:,:,1] # get green channel
b1 = image[:,:,2] # get red channel
Share your thoughts in the comments
Please Login to comment...