Python OpenCV | cv2.imshow() method
Last Updated :
04 Jan, 2023
OpenCV-Python is a library of Python bindings designed to solve computer vision problems. cv2.imshow() method is used to display an image in a window. The window automatically fits the image size.
Syntax: cv2.imshow(window_name, image)
Parameters:
window_name: A string representing the name of the window in which image to be displayed.
image: It is the image that is to be displayed.
Return Value: It doesn’t returns anything.
Image used for all the below examples:
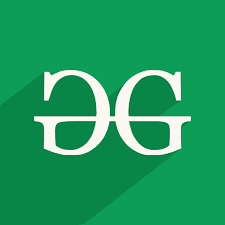
Example #1:
Python3
import cv2
path = r 'C:\Users\Rajnish\Desktop\geeksforgeeks.png'
image = cv2.imread(path)
window_name = 'image'
cv2.imshow(window_name, image)
cv2.waitKey( 0 )
cv2.destroyAllWindows()
|
Output:
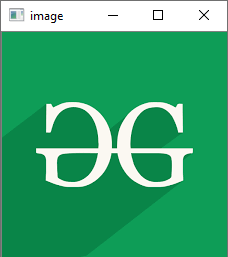
Example #2:
Python3
import cv2
path = r 'C:\Users\Rajnish\Desktop\geeksforgeeks.png'
image = cv2.imread(path, 0 )
window_name = 'image'
cv2.imshow(window_name, image)
cv2.waitKey( 0 )
cv2.destroyAllWindows()
|
Output:
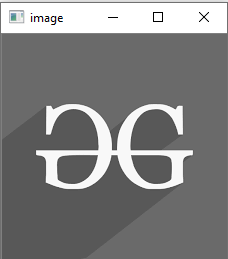
Note: While using Google Colab, one may run into an error of “imshow disabled for collab”, in that case, it’s suggested to use imshow method from colabs patches by importing it first,
Python3
from google.colab.patches import cv2_imshow
window_name = "Image"
cv2_imshow(img)
|
Share your thoughts in the comments
Please Login to comment...