Python OpenCV | cv2.erode() method
Last Updated :
04 Jan, 2023
OpenCV-Python is a library of Python bindings designed to solve computer vision problems. cv2.erode()
method is used to perform erosion on the image. The basic idea of erosion is just like soil erosion only, it erodes away the boundaries of foreground object (Always try to keep foreground in white). It is normally performed on binary images. It needs two inputs, one is our original image, second one is called structuring element or kernel which decides the nature of operation. A pixel in the original image (either 1 or 0) will be considered 1 only if all the pixels under the kernel is 1, otherwise it is eroded (made to zero).
Syntax: cv2.erode(src, kernel[, dst[, anchor[, iterations[, borderType[, borderValue]]]]])
Parameters:
src: It is the image which is to be eroded .
kernel: A structuring element used for erosion. If element = Mat(), a 3 x 3 rectangular structuring element is used. Kernel can be created using getStructuringElement.
dst: It is the output image of the same size and type as src.
anchor: It is a variable of type integer representing anchor point and it’s default value Point is (-1, -1) which means that the anchor is at the kernel center.
borderType: It depicts what kind of border to be added. It is defined by flags like cv2.BORDER_CONSTANT, cv2.BORDER_REFLECT, etc.
iterations: It is number of times erosion is applied.
borderValue: It is border value in case of a constant border.
Return Value: It returns an image.
Image used for all the below examples:
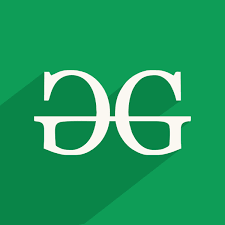
Example #1:
import cv2
import numpy as np
path = r 'C:\Users\Rajnish\Desktop\geeksforgeeks\geeks.png'
image = cv2.imread(path)
window_name = 'Image'
kernel = np.ones(( 5 , 5 ), np.uint8)
image = cv2.erode(image, kernel)
cv2.imshow(window_name, image)
|
Output:
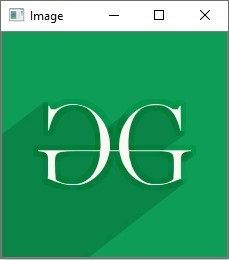
Example #2:
import cv2
import numpy as np
path = r 'C:\Users\Rajnish\Desktop\geeksforgeeks\geeks.png'
image = cv2.imread(path)
window_name = 'Image'
kernel = np.ones(( 6 , 6 ), np.uint8)
image = cv2.erode(image, kernel, cv2.BORDER_REFLECT)
cv2.imshow(window_name, image)
|
Output:
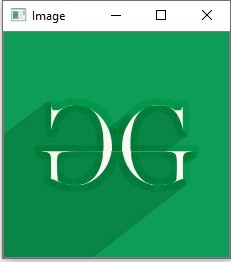
Share your thoughts in the comments
Please Login to comment...