How to Center an Element Horizontally and Vertically ?
Last Updated :
06 Mar, 2024
We will learn how to center an element horizontally and vertically. Centering an element both horizontally and vertically is a common task in web development. It ensures that the element is always displayed at the center of the webpage, regardless of the screen size. Whether you’re aligning text, images, or other content, mastering these techniques will enhance your layout skills. There are several approaches to achieving this goal in CSS. We will discuss a few of the approaches.
Before that, let’s first create an HTML structure, by using a div inside the body tag and giving it a class of container, and inside the container another div with the class element. We will try to center the element class and the container class takes up the total height of the viewport height. Let’s see the HTML code.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Centering an element horizontally and vertically
</ title >
< style >
.container {
height: 100vh;
/* Set the height of the container to the viewport height */
}
.element {
background-color: #308D46;
padding: 20px;
color: #fff;
}
</ style >
</ head >
< body >
< div class = "container" >
< div class = "element" >GEEKS FOR GEEKS</ div >
</ div >
</ body >
</ html >
|
There are several ways to center an element both horizontally and vertically on a web page, but the most common approach is to use CSS. There are a few methods using CSS:
Examples of Centering an Element Horizontally and Vertically
1. Using Flexbox:
Flexbox is a powerful layout model that simplifies centering. To center an element both horizontally and vertically, follow these steps:Â
- Set the display property of the parent element to “flex” and set the justify-content and align-items properties to “center“.Â
- Flexbox: It is a layout mode in CSS that is used to align and distribute space among items in a container. It is a one-dimensional layout and is used to align elements either horizontally or vertically.
- justify-content: Used in Flexbox that defines how the browser distributes free space between and around content items along the main axis of the container.
- align-items: It is a CSS property used in Flexbox that defines how the browser distributes free space between and around content items along the cross-axis of the container.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Centering an element using Flexbox
</ title >
< style >
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
/* Set the height of the container to the viewport height */
}
.element {
background-color: #308D46;
padding: 20px;
color: #fff;
}
</ style >
</ head >
< body >
< div class = "container" >
< div class = "element" >GEEKS FOR GEEKS</ div >
</ div >
</ body >
</ html >
|
Output:
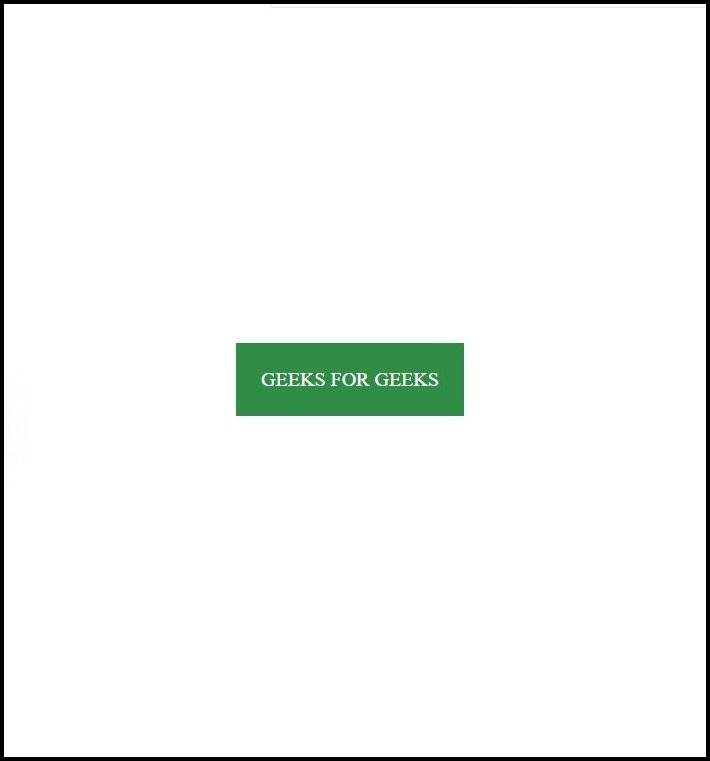
Center an Element Horizontally and Vertically using flexbox
2. Using Grid:
- Set the display property of the parent element to “grid” and use the “place-items“ property to center the child element.
- grid: It is a layout mode in CSS that is used to align and distribute space among items in a container. It is a two-dimensional layout and is used to align elements both horizontally and vertically.
- place-items: It is a CSS property used in the grid that sets both the align-items and justify-items properties of the grid items in a container.
HTML
<!DOCTYPE html>
< html >
< head >
< style >
.container {
display: grid;
/* set height of the container to the viewport height */
height: 100vh;
/* to center the grid items both horizontally and vertically */
place-items: center;
}
.element {
background-color: #308D46;
padding: 20px;
color: #fff;
}
</ style >
</ head >
< body >
< div class = "container" >
< div class = "element" >GEEKS FOR GEEKS</ div >
</ div >
</ body >
</ html >
|
Output:
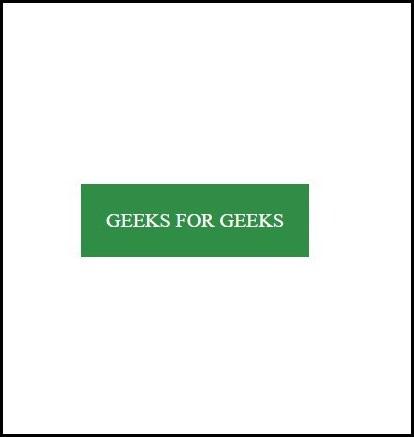
Center an Element Horizontally and Vertically using grid
3. Using position and transform:Â
- Set the position property of the child element to “absolute” and use the transform property to translate the element to the center of the parent element.
- absolute: It is a value of the position property used in CSS which takes an element out of the normal document flow and positions it relative to the nearest positioned ancestor.
- transform: It is a CSS property used to modify the appearance of an element. In the context of centering, the translate() function of the transform property is used to move an element horizontally and vertically.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Centering an element using Flexbox</ title >
< style >
.element {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background-color: #308D46;
padding: 20px;
color: white;
}
</ style >
</ head >
< body >
< div class = "container" >
< div class = "element" >GEEKS FOR GEEKS</ div >
</ div >
</ body >
</ html >
|
Output:
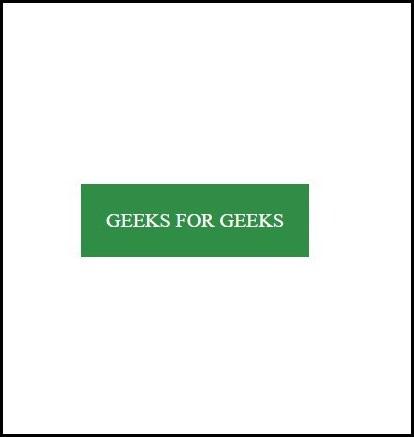
Center an Element Horizontally and Vertically using position and transform
4. Using JavaScript:Â Â
The code first selects the element to be centered using its ID. It then defines a function to calculate and set the position of the element relative to the window dimensions. This function is called both on page load and whenever the window is resized, ensuring the element remains centered dynamically as the window size changes.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Center Element with JavaScript</ title >
< style >
#element {
width: 250px;
height: 100px;
background-color: green;
color: #fff;
text-align: center;
line-height: 100px;
font-size: 24px;
}
</ style >
</ head >
< body >
< div id = "element" >GEEKS FOR GEEKS</ div >
< script >
window.addEventListener('load', () => {
const elem = document.getElementById('element');
function centerElement() {
const elemWidth = elem.offsetWidth;
const elemHeight = elem.offsetHeight;
const windowWidth = window.innerWidth;
const windowHeight = window.innerHeight;
elem.style.position = 'absolute';
elem.style.top = `${(windowHeight - elemHeight) / 2}px`;
elem.style.left = `${(windowWidth - elemWidth) / 2}px`;
}
// Call centerElement initially
centerElement();
// Call centerElement whenever the window is resized
window.addEventListener('resize', centerElement);
});
</ script >
</ body >
</ html >
|
Output:
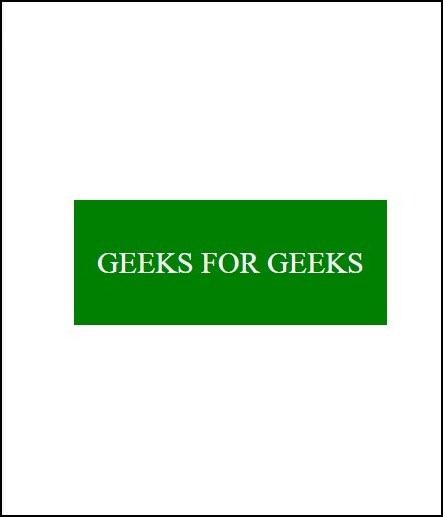
Center an Element Horizontally and Vertically using javascript
Share your thoughts in the comments
Please Login to comment...