Python | Morphological Operations in Image Processing (Opening) | Set-1
Last Updated :
04 Jan, 2023
Morphological operations are used to extract image components that are useful in the representation and description of region shape. Morphological operations are some basic tasks dependent on the picture shape. It is typically performed on binary images. It needs two data sources, one is the input image, the second one is called structuring component. Morphological operators take an input image and a structuring component as input and these elements are then combines using the set operators. The objects in the input image are processed depending on attributes of the shape of the image, which are encoded in the structuring component.
Opening is similar to erosion as it tends to remove the bright foreground pixels from the edges of regions of foreground pixels. The impact of the operator is to safeguard foreground region that has similarity with the structuring component, or that can totally contain the structuring component while taking out every single other area of foreground pixels. Opening operation is used for removing internal noise in an image.
Opening is erosion operation followed by dilation operation.

Syntax: cv2.morphologyEx(image, cv2.MORPH_OPEN, kernel)
Parameters:
-> image: Input Image array.
-> cv2.MORPH_OPEN: Applying the Morphological Opening operation.
-> kernel: Structuring element.
Below is the Python code explaining Opening Morphological Operation –
Python3
import cv2
import numpy as np
screenRead = cv2.VideoCapture( 0 )
while ( 1 ):
_, image = screenRead.read()
hsv = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
blue1 = np.array([ 110 , 50 , 50 ])
blue2 = np.array([ 130 , 255 , 255 ])
mask = cv2.inRange(hsv, blue1, blue2)
res = cv2.bitwise_and(image, image, mask = mask)
kernel = np.ones(( 5 , 5 ), np.uint8)
opening = cv2.morphologyEx(mask, cv2.MORPH_OPEN, kernel)
cv2.imshow( 'Mask' , mask)
cv2.imshow( 'Opening' , opening)
if cv2.waitKey( 1 ) & 0xFF = = ord ( 'a' ):
break
cv2.destroyAllWindows()
screenRead.release()
|
Input Frame:
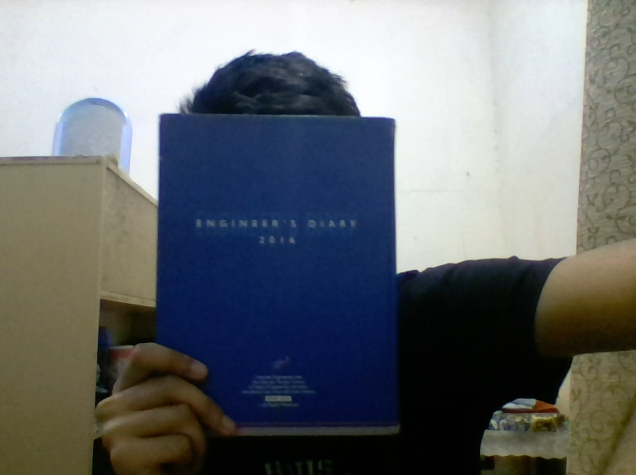
Mask:
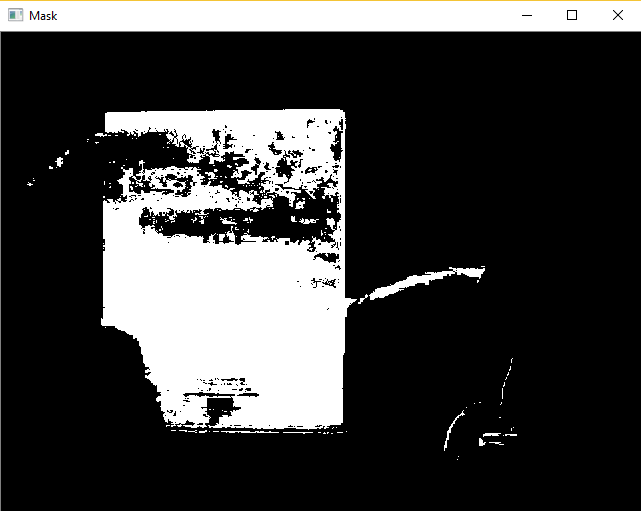
Output Frame:
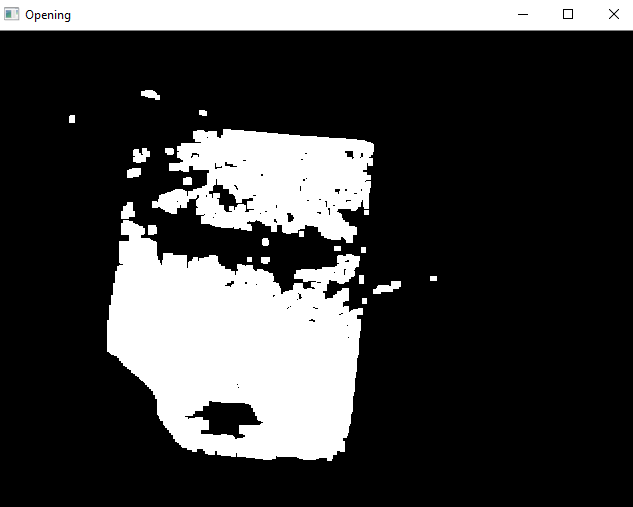
The system recognizes the defined blue book as the input as removes and simplifies the internal noise in the region of interest with the help of the Opening function.
Share your thoughts in the comments
Please Login to comment...