Puzzle 29 | (Car Wheel Puzzle)
Last Updated :
20 Mar, 2024
Puzzle: A car has 4 tyres and 1 spare tyre. Each tyre can travel a maximum distance of 20000 km before wearing off. What is the maximum distance the car can travel before you are forced to buy a new tyre? You are allowed to change tyres (using the spare tyre) an unlimited number of times.Â
Â
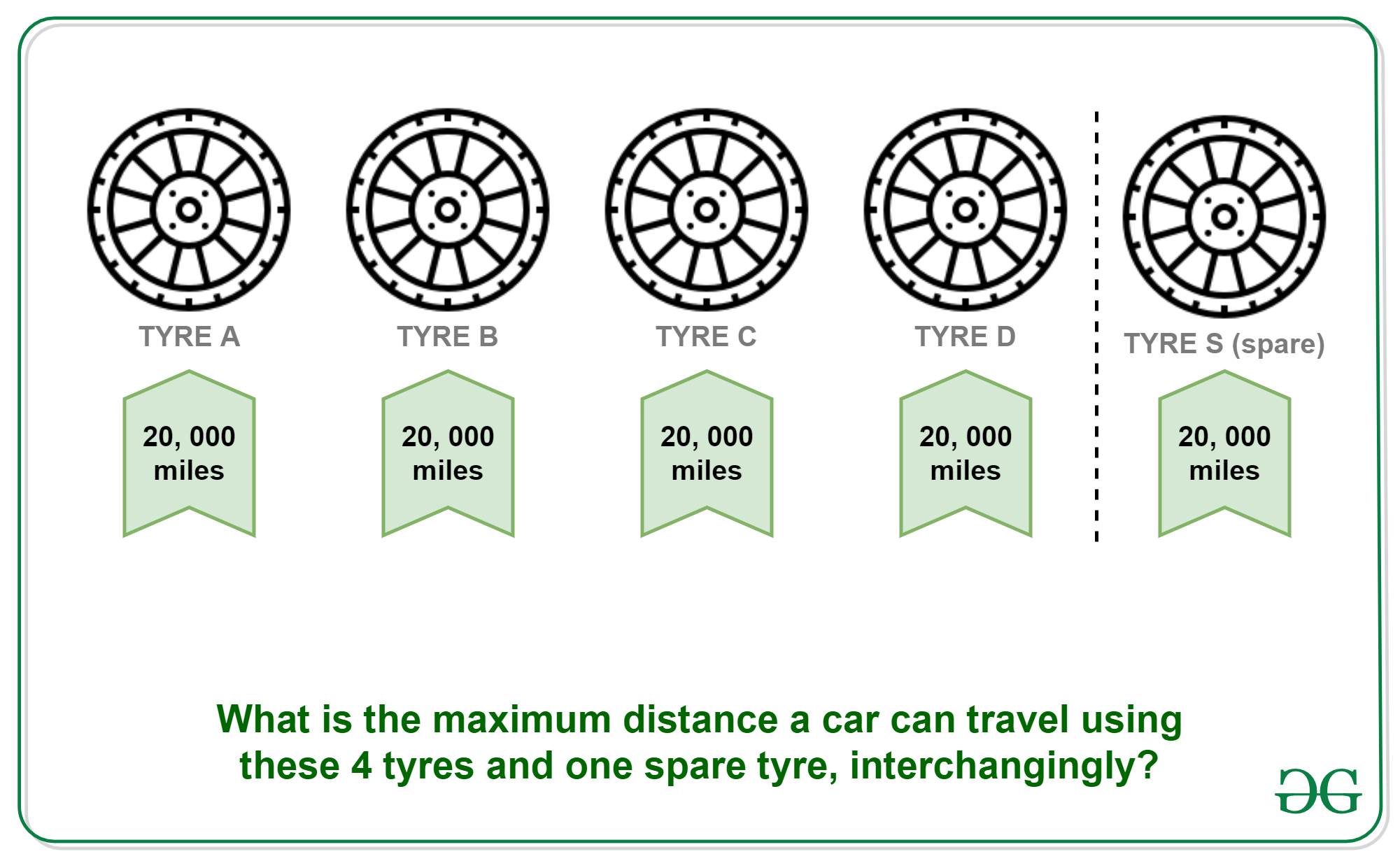
Answer: 25000 kms
Solution: Divide the lifetime of the spare tyre into 4 equal part i.e., 5000 and swap it at each completion of 5000 km distance.Â
Let four tyres be named as A, B, C and D and spare tyre be S.
Â
- 5000 KMs: Replace A with S. Remaining distances (A, B, C, D, S) : 15000, 15000, 15000, 15000, 20000.Â
Â
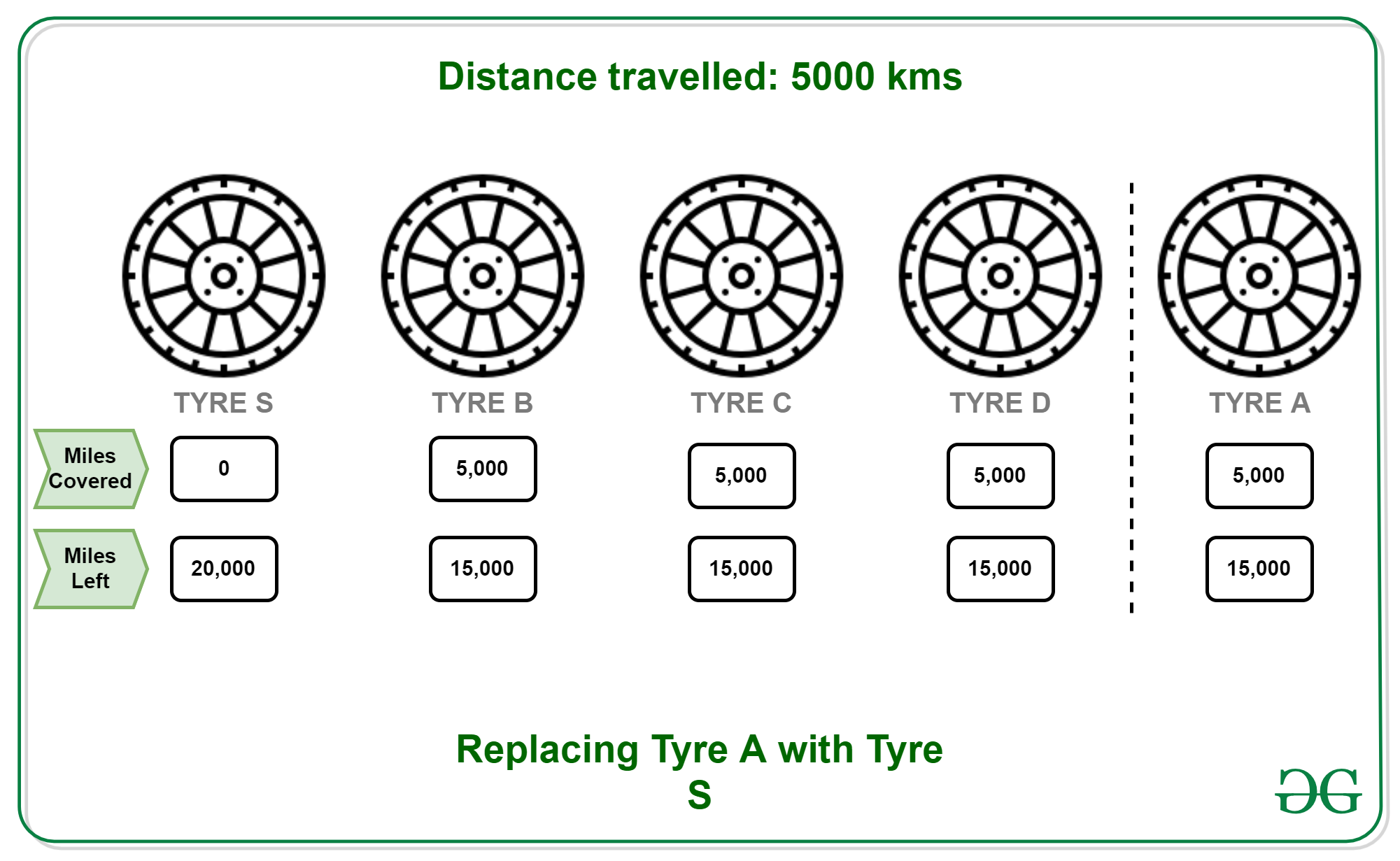
- 10000 KMs: Put A back to its original position and replace B with S. Remaining distances (A, B, C, D, S) : 15000, 10000, 10000, 10000, 15000.Â
Â
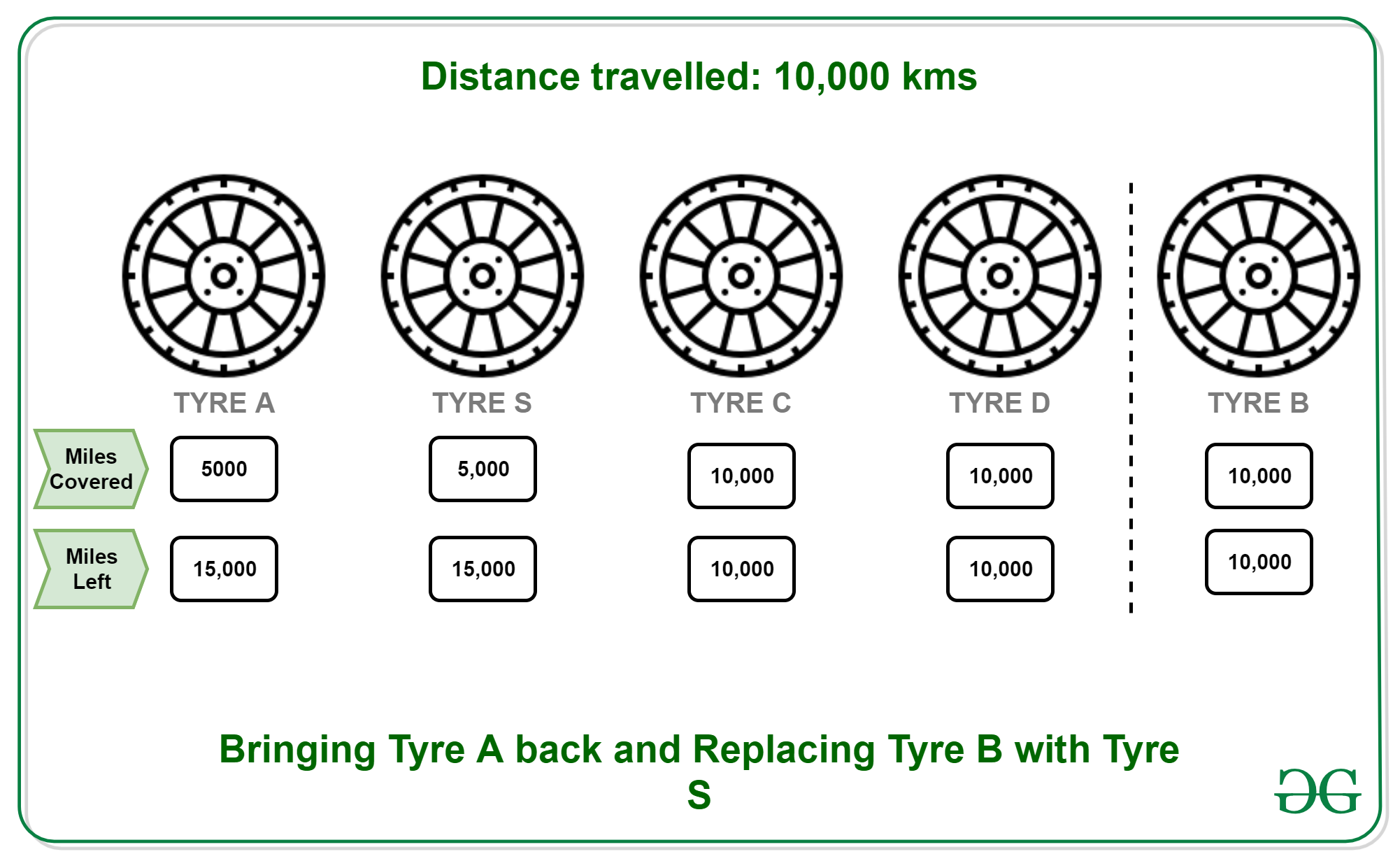
- 15000 KMs: Put B back to its original position and replace C with S. Remaining distances (A, B, C, D, S) : 10000, 10000, 5000, 5000, 10000.Â
Â
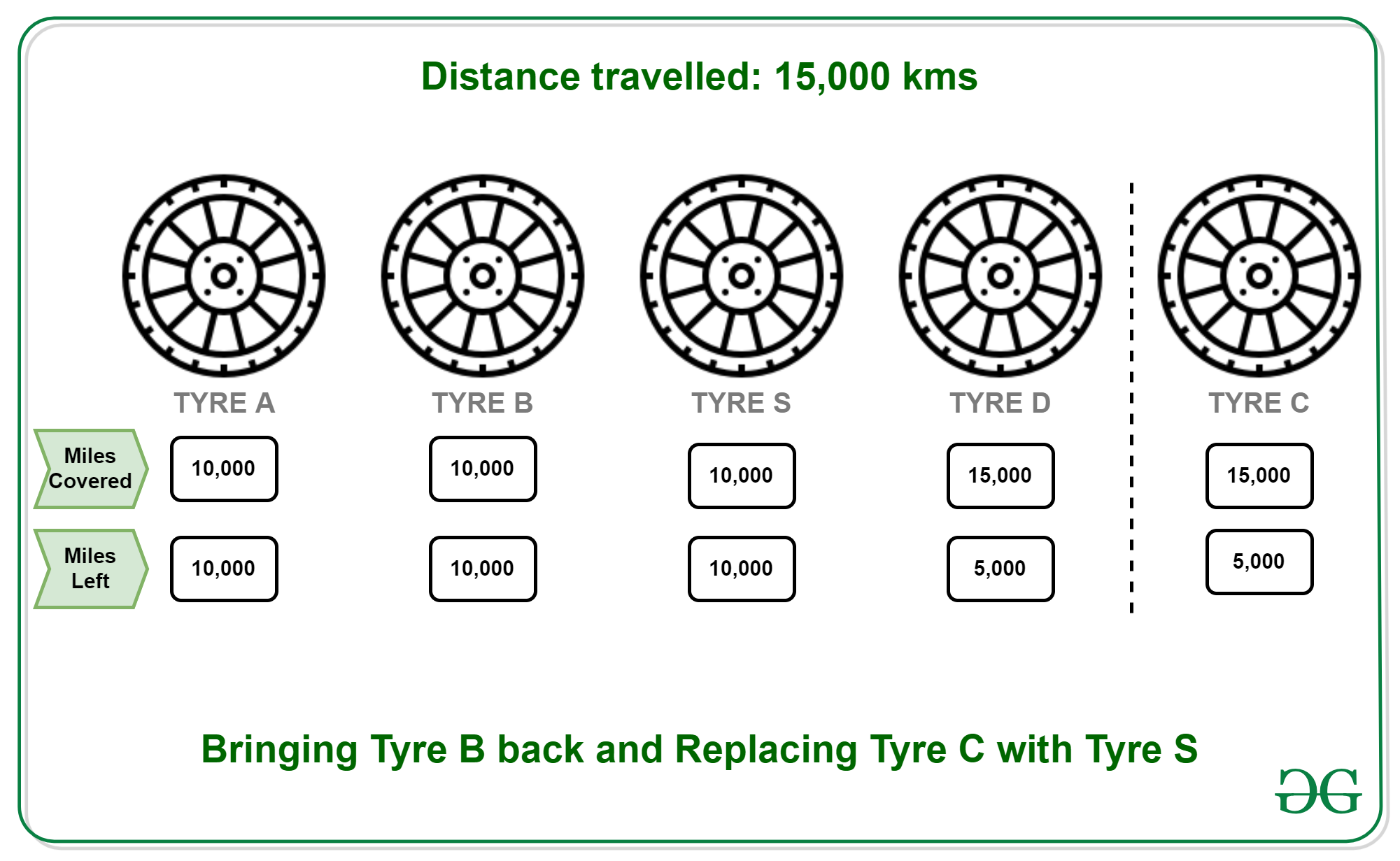
- 20000 KMs: Put C back to its original position and replace D with S. Remaining distances (A, B, C, D, S) : 5000, 5000, 5000, 0, 5000.Â
Â
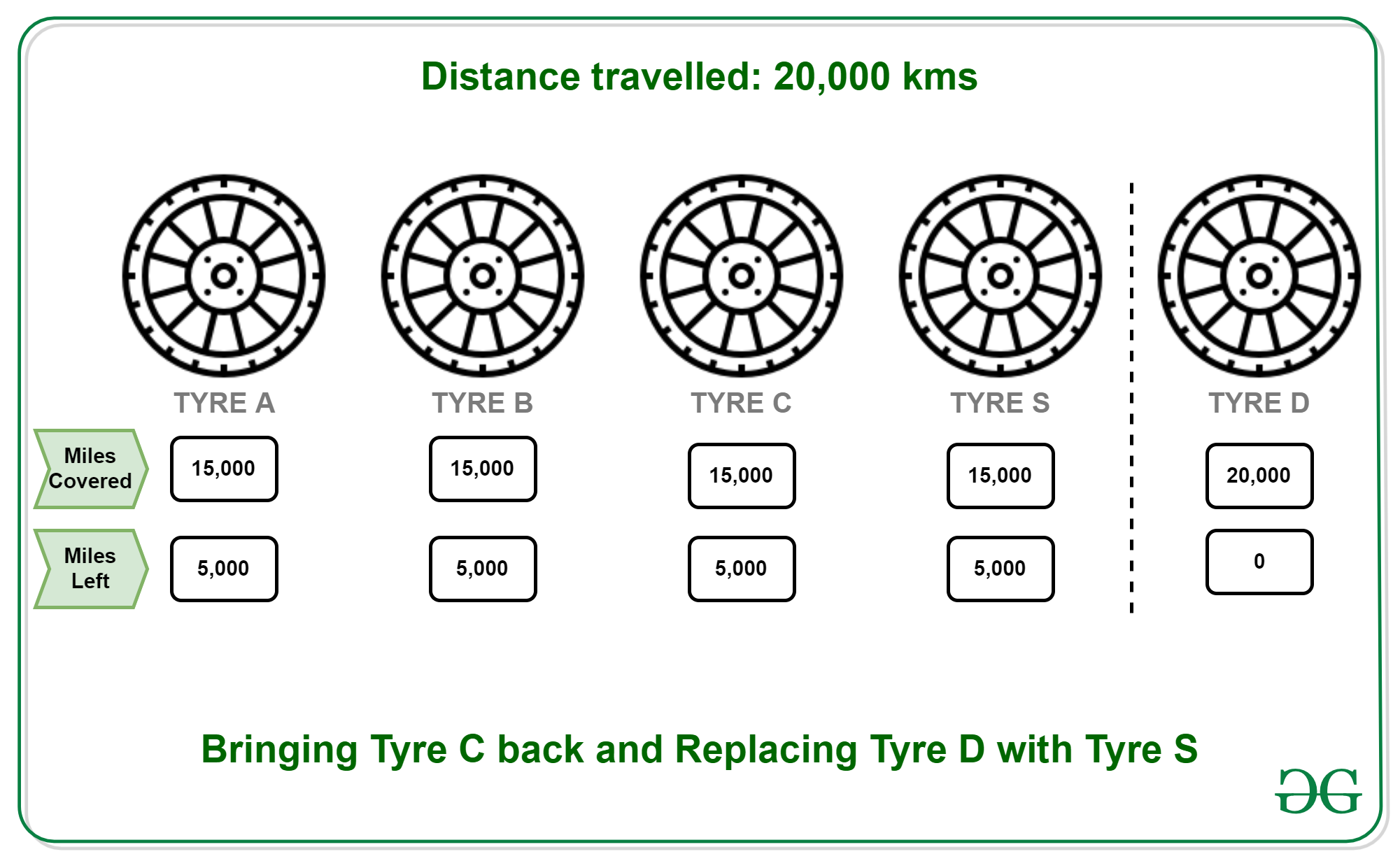
- 25000 KMs: Every tyre is now worn out completely.Â
Â
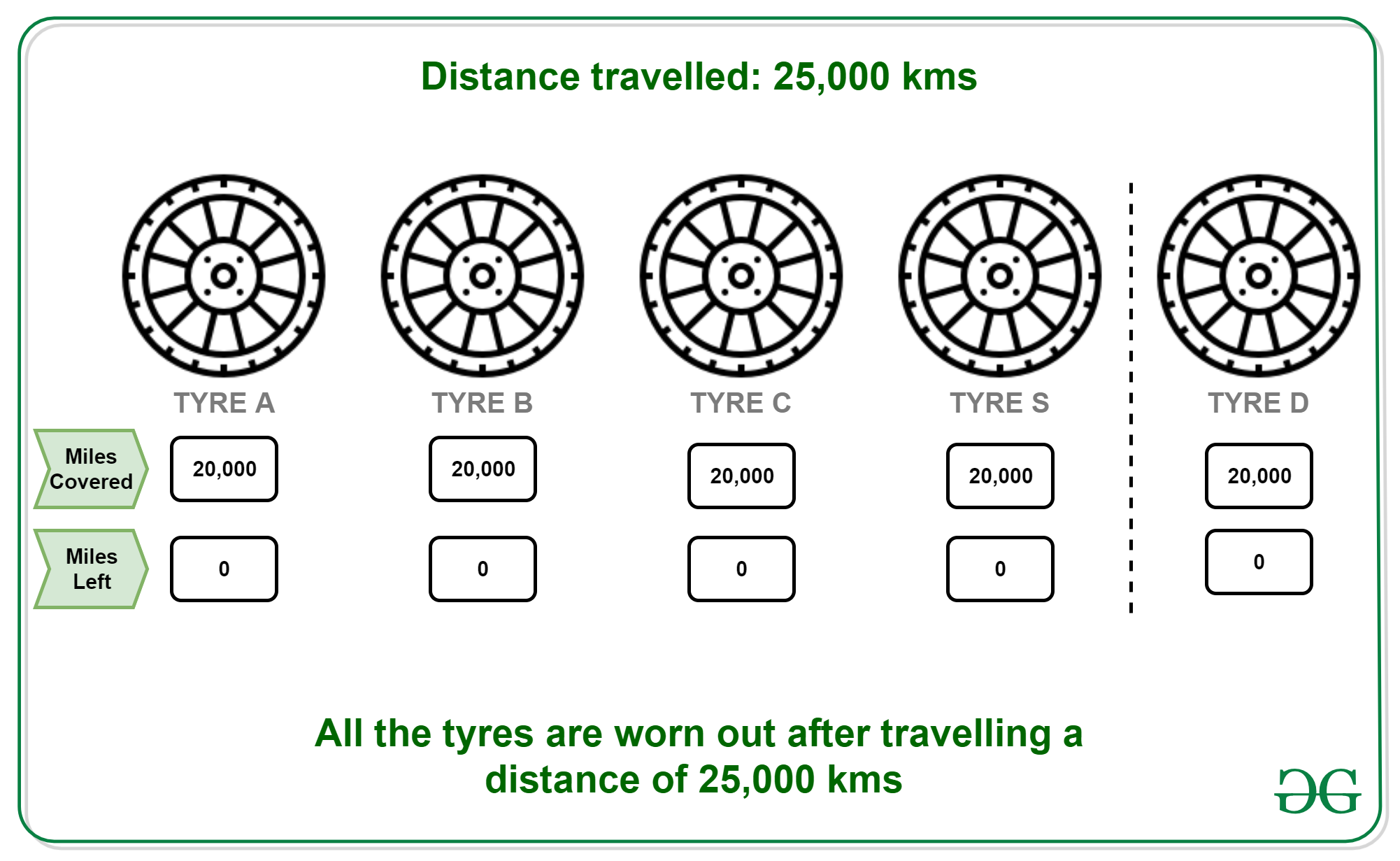
All tyres are used to their full strength.Â
Â
Â
Related Amazon interview question
There are n pencils, each having l length. Each can write 4 kilometres. After writing 4 kilometers it has l/4 length. One can join 4 pencils which are having l/4 length and can make 1 pencil. One can’t make pencil of pieces if remaining pieces are 3 or 2 or 1 in number but one can include these remaining pieces whenever needed.Â
Write a relation independent of l, length of the given pencil, for how much one can write from n pencils.Â
Examples:Â
Â
Input: 4
Output: 20
Â
Recursive Approach:Â
Suppose we use 3 pencils that will 12 and generate 3 used pencils, now if the remaining pencils are greater than zero at least 1 unused pencil can be used with those 3 unused to write 4 and that will generate 1 more unused pencil. This will keep repeating.
Â
if(n-3 >= 1){
f(n) = f(n-3) + 12 + 4
}
else{
// Used pencil that cannot be used
f(n) = 4*n
}
Below is the implementation of the above approach:Â
C++
#include <iostream>
int count( int n) {
if (n < 4) {
return (n * 4);
}
else {
return (16 + count(n - 3));
}
}
int main() {
int input_number = 7;
int result = count(input_number);
std::cout << "Result for n = " << input_number << ": " << result << std::endl;
return 0;
}
|
C
int count( int n)
{
if (n < 4) {
return (n * 4);
}
else {
return (16 + count(n - 3));
}
}
|
Java
static int count( int n)
{
if (n < 4 ) {
return (n * 4 );
}
else {
return ( 16 + count(n - 3 ));
}
}
|
Python3
def count( n):
if (n < 4 ):
return (n * 4 )
else :
return ( 16 + count(n - 3 ))
|
C#
static int count( int n)
{
if (n < 4) {
return (n * 4);
}
else {
return (16 + count(n - 3));
}
}
|
Javascript
function count(n) {
if (n < 4) {
return n * 4;
} else {
return 16 + count(n - 3);
}
}
|
Mathematical Approach O(1):Â
Above relation can be optimized in O(1)
Â
// x is max no of time we can
//subtract 3 without n-3 <= 3
n - 3*x <= 3
x > (n-3)/3
i.e. n/3 - 1 if it divides exactly
else n/3
Below is the implementation of the above approach:Â Â
CPP
#include <bits/stdc++.h>
using namespace std;
int count( int n)
{
int x = (n / 3) - 1;
if (n % 3) {
x++;
}
return (4 * x + 4 * n);
}
int main()
{
int n = 5;
cout << count(n) << endl;
}
|
Java
class GFG
{
static int count( int n)
{
int x = (n / 3 ) - 1 ;
if (n % 3 > 0 )
{
x++;
}
return ( 4 * x + 4 * n);
}
public static void main(String[] args)
{
int n = 5 ;
System.out.print(count(n) + "\n" );
}
}
|
Python3
def count(n):
x = (n / / 3 ) - 1 ;
if (n % 3 > 0 ):
x + = 1 ;
return ( 4 * x + 4 * n);
if __name__ = = '__main__' :
n = 5 ;
print (count(n));
|
C#
using System;
class GFG
{
static int count( int n)
{
int x = (n / 3) - 1;
if (n % 3 > 0)
{
x++;
}
return (4 * x + 4 * n);
}
public static void Main(String[] args)
{
int n = 5;
Console.Write(count(n) + "\n" );
}
}
|
Javascript
<script>
function count(n)
{
let x = Math.floor(n / 3) - 1;
if (n % 3 > 0)
{
x++;
}
return (4 * x + 4 * n);
}
let n = 5;
document.write(count(n) + "<br>" );
</script>
|
Share your thoughts in the comments
Please Login to comment...