Object Oriented Programming (OOPs) Concept in Java
Last Updated :
21 Jul, 2023
As the name suggests, Object-Oriented Programming or OOPs refers to languages that use objects in programming, they use objects as a primary source to implement what is to happen in the code. Objects are seen by the viewer or user, performing tasks assigned by you. Object-oriented programming aims to implement real-world entities like inheritance, hiding, polymorphism etc. in programming. The main aim of OOP is to bind together the data and the functions that operate on them so that no other part of the code can access this data except that function.
-Concept-in-Java.webp)
Let us discuss prerequisites by polishing concepts of method declaration and message passing. Starting off with the method declaration, it consists of six components:
- Access Modifier: Defines the access type of the method i.e. from where it can be accessed in your application. In Java, there are 4 types of access specifiers:
- public: Accessible in all classes in your application.
- protected: Accessible within the package in which it is defined and in its subclass(es) (including subclasses declared outside the package).
- private: Accessible only within the class in which it is defined.
- default (declared/defined without using any modifier): Accessible within the same class and package within which its class is defined.
- The return type: The data type of the value returned by the method or void if it does not return a value.
- Method Name: The rules for field names apply to method names as well, but the convention is a little different.
- Parameter list: Comma-separated list of the input parameters that are defined, preceded by their data type, within the enclosed parentheses. If there are no parameters, you must use empty parentheses ().
- Exception list: The exceptions you expect the method to throw. You can specify these exception(s).
- Method body: It is the block of code, enclosed between braces, that you need to execute to perform your intended operations.
Message Passing: Objects communicate with one another by sending and receiving information to each other. A message for an object is a request for execution of a procedure and therefore will invoke a function in the receiving object that generates the desired results. Message passing involves specifying the name of the object, the name of the function and the information to be sent.
Master OOP in Java Write cleaner, more modular, and reusable Java code by building a foundation in object-oriented programming with Educative’s interactive course Learn Object-Oriented Programming in Java. Sign up at Educative.io with the code GEEKS10 to save 10% on your subscription.
Now that we have covered the basic prerequisites, we will move on to the 4 pillars of OOPs which are as follows. But, let us start by learning about the different characteristics of an Object-Oriented Programming Language.
OOPS concepts are as follows:
- Class
- Object
- Method and method passing
- Pillars of OOPs
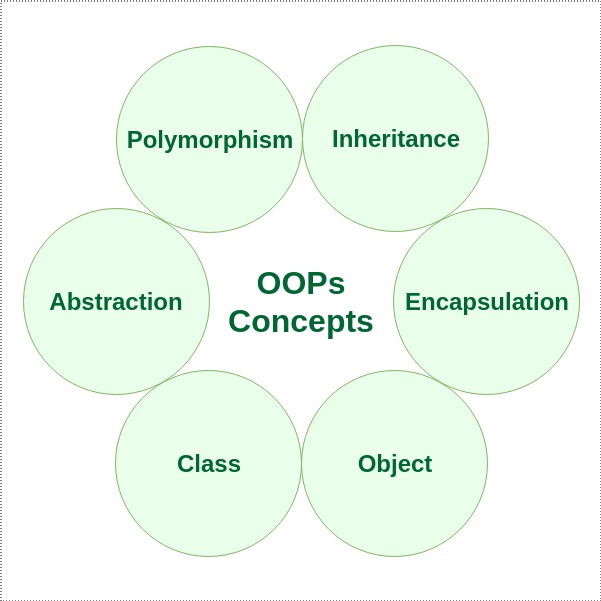
A class is a user-defined blueprint or prototype from which objects are created. It represents the set of properties or methods that are common to all objects of one type. Using classes, you can create multiple objects with the same behavior instead of writing their code multiple times. This includes classes for objects occurring more than once in your code. In general, class declarations can include these components in order:
- Modifiers: A class can be public or have default access (Refer to this for details).
- Class name: The class name should begin with the initial letter capitalized by convention.
- Superclass (if any): The name of the class’s parent (superclass), if any, preceded by the keyword extends. A class can only extend (subclass) one parent.
- Interfaces (if any): A comma-separated list of interfaces implemented by the class, if any, preceded by the keyword implements. A class can implement more than one interface.
- Body: The class body is surrounded by braces, { }.
An object is a basic unit of Object-Oriented Programming that represents real-life entities. A typical Java program creates many objects, which as you know, interact by invoking methods. The objects are what perform your code, they are the part of your code visible to the viewer/user. An object mainly consists of:
- State: It is represented by the attributes of an object. It also reflects the properties of an object.
- Behavior: It is represented by the methods of an object. It also reflects the response of an object to other objects.
- Identity: It is a unique name given to an object that enables it to interact with other objects.
- Method: A method is a collection of statements that perform some specific task and return the result to the caller. A method can perform some specific task without returning anything. Methods allow us to reuse the code without retyping it, which is why they are considered time savers. In Java, every method must be part of some class, which is different from languages like C, C++, and Python.
class and objects one simple java program :
Java
public class GFG {
static String Employee_name;
static float Employee_salary;
static void set(String n, float p) {
Employee_name = n;
Employee_salary = p;
}
static void get() {
System.out.println( "Employee name is: " +Employee_name );
System.out.println( "Employee CTC is: " + Employee_salary);
}
public static void main(String args[]) {
GFG.set( "Rathod Avinash" , 10000 .0f);
GFG.get();
}
}
|
Output
Employee name is: Rathod Avinash
Employee CTC is: 10000.0
Let us now discuss the 4 pillars of OOPs:
Data Abstraction is the property by virtue of which only the essential details are displayed to the user. The trivial or non-essential units are not displayed to the user. Ex: A car is viewed as a car rather than its individual components.
Data Abstraction may also be defined as the process of identifying only the required characteristics of an object, ignoring the irrelevant details. The properties and behaviors of an object differentiate it from other objects of similar type and also help in classifying/grouping the object.
Consider a real-life example of a man driving a car. The man only knows that pressing the accelerators will increase the car speed or applying brakes will stop the car, but he does not know how on pressing the accelerator, the speed is actually increasing. He does not know about the inner mechanism of the car or the implementation of the accelerators, brakes etc. in the car. This is what abstraction is.
In Java, abstraction is achieved by interfaces and abstract classes. We can achieve 100% abstraction using interfaces.
The abstract method contains only method declaration but not implementation.
Demonstration of Abstract class
Java
abstract class GFG{
abstract void add();
abstract void mul();
abstract void div();
}
|
It is defined as the wrapping up of data under a single unit. It is the mechanism that binds together the code and the data it manipulates. Another way to think about encapsulation is that it is a protective shield that prevents the data from being accessed by the code outside this shield.
- Technically, in encapsulation, the variables or the data in a class is hidden from any other class and can be accessed only through any member function of the class in which they are declared.
- In encapsulation, the data in a class is hidden from other classes, which is similar to what data-hiding does. So, the terms “encapsulation” and “data-hiding” are used interchangeably.
- Encapsulation can be achieved by declaring all the variables in a class as private and writing public methods in the class to set and get the values of the variables.
Demonstration of Encapsulation:
Java
class Employee {
private int empid;
private String ename;
}
|
Inheritance is an important pillar of OOP (Object Oriented Programming). It is the mechanism in Java by which one class is allowed to inherit the features (fields and methods) of another class. We are achieving inheritance by using extends keyword. Inheritance is also known as “is-a” relationship.
Let us discuss some frequently used important terminologies:
- Superclass: The class whose features are inherited is known as superclass (also known as base or parent class).
- Subclass: The class that inherits the other class is known as subclass (also known as derived or extended or child class). The subclass can add its own fields and methods in addition to the superclass fields and methods.
- Reusability: Inheritance supports the concept of “reusability”, i.e. when we want to create a new class and there is already a class that includes some of the code that we want, we can derive our new class from the existing class. By doing this, we are reusing the fields and methods of the existing class.
Demonstration of Inheritance :
Java
class A{
void method1(){}
void method2(){}
}
class B extends A{
void method3(){}
void method4(){}
}
|
It refers to the ability of object-oriented programming languages to differentiate between entities with the same name efficiently. This is done by Java with the help of the signature and declaration of these entities. The ability to appear in many forms is called polymorphism.
E.g.
Java
sleep( 1000 )
sleep( 1000 , 2000 )
|
Note: Polymorphism in Java is mainly of 2 types:
- Overloading
- Overriding
Example
Java
public class Sum {
public int sum( int x, int y)
{
return (x + y);
}
public int sum( int x, int y, int z)
{
return (x + y + z);
}
public double sum( double x, double y)
{
return (x + y);
}
public static void main(String args[])
{
Sum s = new Sum();
System.out.println(s.sum( 10 , 20 ));
System.out.println(s.sum( 10 , 20 , 30 ));
System.out.println(s.sum( 10.5 , 20.5 ));
}
}
|
Share your thoughts in the comments
Please Login to comment...