Abstraction in Java
Last Updated :
15 Dec, 2023
Abstraction in Java is the process in which we only show essential details/functionality to the user. The non-essential implementation details are not displayed to the user.
In this article, we will learn about abstraction and what abstract means.
Simple Example to understand Abstraction:
Television remote control is an excellent example of abstraction. It simplifies the interaction with a TV by hiding the complexity behind simple buttons and symbols, making it easy without needing to understand the technical details of how the TV functions.
What is Abstraction in Java?
In Java, abstraction is achieved by interfaces and abstract classes. We can achieve 100% abstraction using interfaces.
Data Abstraction may also be defined as the process of identifying only the required characteristics of an object ignoring the irrelevant details. The properties and behaviours of an object differentiate it from other objects of similar type and also help in classifying/grouping the objects.
Abstraction Real-Life Example:
Consider a real-life example of a man driving a car. The man only knows that pressing the accelerators will increase the speed of a car or applying brakes will stop the car, but he does not know how on pressing the accelerator the speed is actually increasing, he does not know about the inner mechanism of the car or the implementation of the accelerator, brakes, etc in the car. This is what abstraction is.
Java Abstract classes and Java Abstract methods
- An abstract class is a class that is declared with an abstract keyword.
- An abstract method is a method that is declared without implementation.
- An abstract class may or may not have all abstract methods. Some of them can be concrete methods
- A method-defined abstract must always be redefined in the subclass, thus making overriding compulsory or making the subclass itself abstract.
- Any class that contains one or more abstract methods must also be declared with an abstract keyword.
- There can be no object of an abstract class. That is, an abstract class can not be directly instantiated with the new operator.
- An abstract class can have parameterized constructors and the default constructor is always present in an abstract class.
Algorithm to implement abstraction in Java
- Determine the classes or interfaces that will be part of the abstraction.
- Create an abstract class or interface that defines the common behaviours and properties of these classes.
- Define abstract methods within the abstract class or interface that do not have any implementation details.
- Implement concrete classes that extend the abstract class or implement the interface.
- Override the abstract methods in the concrete classes to provide their specific implementations.
- Use the concrete classes to implement the program logic.
When to use abstract classes and abstract methods?
There are situations in which we will want to define a superclass that declares the structure of a given abstraction without providing a complete implementation of every method. Sometimes we will want to create a superclass that only defines a generalization form that will be shared by all of its subclasses, leaving it to each subclass to fill in the details.
Consider a classic “shape” example, perhaps used in a computer-aided design system or game simulation. The base type is “shape” and each shape has a color, size, and so on. From this, specific types of shapes are derived(inherited)-circle, square, triangle, and so on — each of which may have additional characteristics and behaviours. For example, certain shapes can be flipped. Some behaviours may be different, such as when you want to calculate the area of a shape. The type hierarchy embodies both the similarities and differences between the shapes.
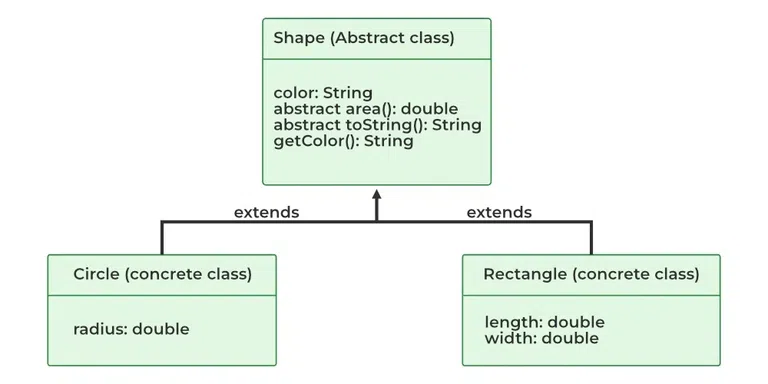
Abstract Class in Java
Java Abstraction Example
Example 1:
Java
abstract class Shape {
String color;
abstract double area();
public abstract String toString();
public Shape(String color)
{
System.out.println( "Shape constructor called" );
this .color = color;
}
public String getColor() { return color; }
}
class Circle extends Shape {
double radius;
public Circle(String color, double radius)
{
super (color);
System.out.println( "Circle constructor called" );
this .radius = radius;
}
@Override double area()
{
return Math.PI * Math.pow(radius, 2 );
}
@Override public String toString()
{
return "Circle color is " + super .getColor()
+ "and area is : " + area();
}
}
class Rectangle extends Shape {
double length;
double width;
public Rectangle(String color, double length,
double width)
{
super (color);
System.out.println( "Rectangle constructor called" );
this .length = length;
this .width = width;
}
@Override double area() { return length * width; }
@Override public String toString()
{
return "Rectangle color is " + super .getColor()
+ "and area is : " + area();
}
}
public class Test {
public static void main(String[] args)
{
Shape s1 = new Circle( "Red" , 2.2 );
Shape s2 = new Rectangle( "Yellow" , 2 , 4 );
System.out.println(s1.toString());
System.out.println(s2.toString());
}
}
|
Output
Shape constructor called
Circle constructor called
Shape constructor called
Rectangle constructor called
Circle color is Redand area is : 15.205308443374602
Rectangle color is Yellowand area is : 8.0
Example 2:
Java
abstract class Animal {
private String name;
public Animal(String name) { this .name = name; }
public abstract void makeSound();
public String getName() { return name; }
}
class Dog extends Animal {
public Dog(String name) { super (name); }
public void makeSound()
{
System.out.println(getName() + " barks" );
}
}
class Cat extends Animal {
public Cat(String name) { super (name); }
public void makeSound()
{
System.out.println(getName() + " meows" );
}
}
public class AbstractionExample {
public static void main(String[] args)
{
Animal myDog = new Dog( "Buddy" );
Animal myCat = new Cat( "Fluffy" );
myDog.makeSound();
myCat.makeSound();
}
}
|
Output
Buddy barks
Fluffy meows
Explanation of the above Java program:
This code defines an Animal abstract class with an abstract method makeSound(). The Dog and Cat classes extend Animal and implement the makeSound() method. The main() method creates instances of Dog and Cat and calls the makeSound() method on them.
This demonstrates the abstraction concept in Java, where we define a template for a class (in this case Animal), but leave the implementation of certain methods to be defined by subclasses (in this case makeSound()).
Interface
Interfaces are another method of implementing abstraction in Java. The key difference is that, by using interfaces, we can achieve 100% abstraction in Java classes. In Java or any other language, interfaces include both methods and variables but lack a method body. Apart from abstraction, interfaces can also be used to implement interfaces in Java.
Implementation: To implement an interface we use the keyword “implements” with class.
Java
interface Shape {
double calculateArea();
}
class Circle implements Shape {
private double radius;
public Circle( double radius) { this .radius = radius; }
public double calculateArea()
{
return Math.PI * radius * radius;
}
}
class Rectangle implements Shape {
private double length;
private double width;
public Rectangle( double length, double width)
{
this .length = length;
this .width = width;
}
public double calculateArea() { return length * width; }
}
public class Main {
public static void main(String[] args)
{
Circle myCircle = new Circle( 5.0 );
Rectangle myRectangle = new Rectangle( 4.0 , 6.0 );
System.out.println( "Area of Circle: "
+ myCircle.calculateArea());
System.out.println( "Area of Rectangle: "
+ myRectangle.calculateArea());
}
}
|
Output
Area of Circle: 78.53981633974483
Area of Rectangle: 24.0
Advantages of Abstraction
Here are some advantages of abstraction:
- It reduces the complexity of viewing things.
- Avoids code duplication and increases reusability.
- Helps to increase the security of an application or program as only essential details are provided to the user.
- It improves the maintainability of the application.
- It improves the modularity of the application.
- The enhancement will become very easy because without affecting end-users we can able to perform any type of changes in our internal system.
- Improves code reusability and maintainability.
- Hides implementation details and exposes only relevant information.
- Provides a clear and simple interface to the user.
- Increases security by preventing access to internal class details.
- Supports modularity, as complex systems can be divided into smaller and more manageable parts.
- Abstraction provides a way to hide the complexity of implementation details from the user, making it easier to understand and use.
- Abstraction allows for flexibility in the implementation of a program, as changes to the underlying implementation details can be made without affecting the user-facing interface.
- Abstraction enables modularity and separation of concerns, making code more maintainable and easier to debug.
Disadvantages of Abstraction in Java
Here are the main disadvantages of abstraction in Java:
- Abstraction can make it more difficult to understand how the system works.
- It can lead to increased complexity, especially if not used properly.
- This may limit the flexibility of the implementation.
- Abstraction can add unnecessary complexity to code if not used appropriately, leading to increased development time and effort.
- Abstraction can make it harder to debug and understand code, particularly for those unfamiliar with the abstraction layers and implementation details.
- Overuse of abstraction can result in decreased performance due to the additional layers of code and indirection.
Also Read:
Abstraction in Java – FAQs
Q1. Why do we use abstract?
One key reason we use abstract concepts is to simplify complexity. Imagine trying to explain the entire universe with every single atom and star! Abstracts let us zoom out, grab the main ideas like gravity and energy, and make sense of it all without getting lost in the details.
Here are some other reasons why we use abstract in Java:
1. Abstraction: Abstract classes are used to define a generic template for other classes to follow. They define a set of rules and guidelines that their subclasses must follow. By providing an abstract class, we can ensure that the classes that extend it have a consistent structure and behavior. This makes the code more organized and easier to maintain.
2. Polymorphism: Abstract classes and methods enable polymorphism in Java. Polymorphism is the ability of an object to take on many forms. This means that a variable of an abstract type can hold objects of any concrete subclass of that abstract class. This makes the code more flexible and adaptable to different situations.
3. Frameworks and APIs: Java has numerous frameworks and APIs that use abstract classes. By using abstract classes developers can save time and effort by building on existing code and focusing on the aspects specific to their applications.
Q2. What is the Difference between Encapsulation and Data Abstraction?
Here are some key difference b/w encapsulation and abstration:
Encapsulation is data hiding(information hiding) |
Abstraction is detailed hiding(implementation hiding). |
Encapsulation groups together data and methods that act upon the data |
Data Abstraction deal with exposing the interface to the user and hiding the details of implementation |
Encapsulated classes are Java classes that follow data hiding and abstraction |
Implementation of abstraction is done using abstract classes and interface |
Encapsulation is a procedure that takes place at the implementation level |
abstraction is a design-level process |
Q3. What is a real-life example of data abstraction?
Television remote control is an excellent real-life example of abstraction. It simplifies the interaction with a TV by hiding the complexity behind simple buttons and symbols, making it easy without needing to understand the technical details of how the TV functions.
Q4. What is the Difference between Abstract Classes and Interfaces in Java?
Here are some key difference b/w Abstract Classes and Interfaces in Java:
Abstract classes support abstract and Non-abstract methods |
Interface supports have abstract methods only. |
Doesn’t support Multiple Inheritance |
Supports Multiple Inheritance |
Abstract classes can be extended by Java classes and multiple interfaces |
The interface can be extended by Java interface only. |
Abstract class members in Java can be private, protected, etc. |
Interfaces are public by default. |
Example:
public abstract class Vechicle{ public abstract void drive() }
|
Example:
public interface Animal{ void speak(); }
|
Share your thoughts in the comments
Please Login to comment...