Association, Composition and Aggregation in Java
Last Updated :
21 Mar, 2024
Association is a relation between two separate classes which is established through their Objects. Association can be one-to-one, one-to-many, many-to-one, many-to-many. In Object-Oriented programming, an Object communicates to another object to use functionality and services provided by that object. Composition and Aggregation are the two forms of association.Â
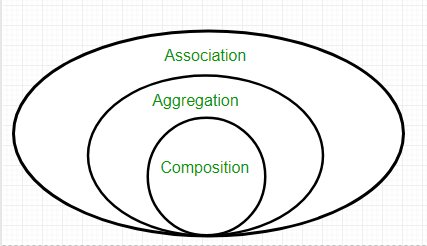
Association
To understand the concept association, refer to the below example.
Example:
Java
// Java Program to illustrate the
// Concept of Association
// Importing required classes
import java.io.*;
import java.util.*;
// Class 1
// Bank class
class Bank {
// Attributes of bank
private String bankName;
private Set<Employee> employees;
// Constructor of Bank class
public Bank(String bankName)
{
this.bankName = bankName;
}
// Method of Bank class
public String getBankName()
{
// Returning name of bank
return this.bankName;
}
public void setEmployees(Set<Employee> employees)
{
this.employees = employees;
}
public Set<Employee> getEmployees()
{
return this.employees;
}
}
// Class 2
// Employee class
class Employee {
// Attributes of employee
private String name;
// Constructor of Employee class
public Employee(String name)
{
// this keyword refers to current instance
this.name = name;
}
// Method of Employee class
public String getEmployeeName()
{
// returning the name of employee
return this.name;
}
}
// Class 3
// Association between both the
// classes in main method
class AssociationExample {
// Main driver method
public static void main(String[] args)
{
// Creating Employee objects
Employee emp1 = new Employee("Ridhi");
Employee emp2 = new Employee("Vijay");
// adding the employees to a set
Set<Employee> employees = new HashSet<>();
employees.add(emp1);
employees.add(emp2);
// Creating a Bank object
Bank bank = new Bank("ICICI");
// setting the employees for the Bank object
bank.setEmployees(employees);
// traversing and displaying the bank employees
for (Employee emp : bank.getEmployees()) {
System.out.println(emp.getEmployeeName()
+ " belongs to bank "
+ bank.getBankName());
}
}
}
OutputRidhi belongs to bank ICICI
Vijay belongs to bank ICICI
Explanation of the above Program:
In the above example, two separate classes Bank and Employee are associated through their Objects. Bank can have many employees, So, it is a one-to-many relationship.Â
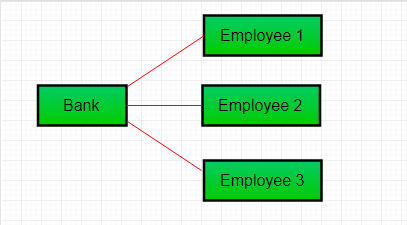
Aggregation
It is a special form of Association where:Â Â
- It represents Has-A’s relationship.
- It is a unidirectional association i.e. a one-way relationship. For example, a department can have students but vice versa is not possible and thus unidirectional in nature.
- In Aggregation, both entries can survive individually which means ending one entity will not affect the other entity.
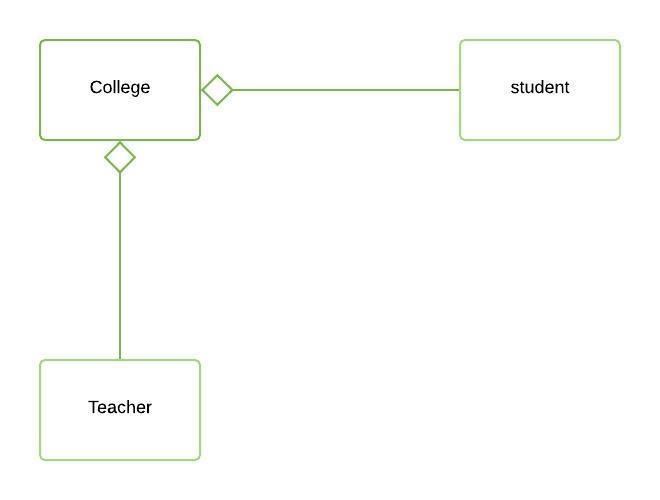
Aggregation Example:
Java
// Java program to illustrate
// Concept of Aggregation
// Importing required classes
import java.io.*;
import java.util.*;
// Class 1
// Student class
class Student {
// Attributes of Student
private String studentName;
private int studentId;
// Constructor of Student class
public Student(String studentName, int studentId)
{
this.studentName = studentName;
this.studentId = studentId;
}
public int getstudentId() {
return studentId;
}
public String getstudentName() {
return studentName;
}
}
// Class 2
// Department class
// Department class contains list of Students
class Department {
// Attributes of Department class
private String deptName;
private List<Student> students;
// Constructor of Department class
public Department(String deptName, List<Student> students)
{
this.deptName = deptName;
this.students = students;
}
public List<Student> getStudents() {
return students;
}
public void addStudent(Student student)
{
students.add(student);
}
}
// Class 3
// Institute class
// Institute class contains the list of Departments
class Institute {
// Attributes of Institute
private String instituteName;
private List<Department> departments;
// Constructor of Institute class
public Institute(String instituteName,
List<Department> departments)
{
// This keyword refers to current instance itself
this.instituteName = instituteName;
this.departments = departments;
}
public void addDepartment(Department department)
{
departments.add(department);
}
// Method of Institute class
// Counting total students in the institute
public int getTotalStudentsInInstitute()
{
int noOfStudents = 0;
List<Student> students = null;
for (Department dept : departments) {
students = dept.getStudents();
for (Student s : students) {
noOfStudents++;
}
}
return noOfStudents;
}
}
// Class 4
// main class
class AggregationExample {
// main driver method
public static void main(String[] args)
{
// Creating independent Student objects
Student s1 = new Student("Parul", 1);
Student s2 = new Student("Sachin", 2);
Student s3 = new Student("Priya", 1);
Student s4 = new Student("Rahul", 2);
// Creating an list of CSE Students
List<Student> cse_students = new ArrayList<Student>();
cse_students.add(s1);
cse_students.add(s2);
// Creating an initial list of EE Students
List<Student> ee_students = new ArrayList<Student>();
ee_students.add(s3);
ee_students.add(s4);
// Creating Department object with a Students list
// using Aggregation (Department "has" students)
Department CSE = new Department("CSE", cse_students);
Department EE = new Department("EE", ee_students);
// Creating an initial list of Departments
List<Department> departments = new ArrayList<Department>();
departments.add(CSE);
departments.add(EE);
// Creating an Institute object with Departments list
// using Aggregation (Institute "has" Departments)
Institute institute = new Institute("BITS", departments);
// Display message for better readability
System.out.print("Total students in institute: ");
// Calling method to get total number of students
// in the institute and printing on console
System.out.print(
institute.getTotalStudentsInInstitute());
}
}
OutputTotal students in institute: 4
Explanation of the above Program:
In this example,
- There is an Institute which has no. of departments like CSE, EE. Every department has no. of students.
- So, we make an Institute class that has a reference to Object or no. of Objects (i.e. List of Objects) of the Department class.
- That means Institute class is associated with Department class through its Object(s).
- And Department class has also a reference to Object or Objects (i.e. List of Objects) of the Student class means it is associated with the Student class through its Object(s).Â
It represents a Has-A relationship. In the above example: Student Has-A name. Student Has-A ID. Department Has-A Students as depicted from the below media.Â
Â
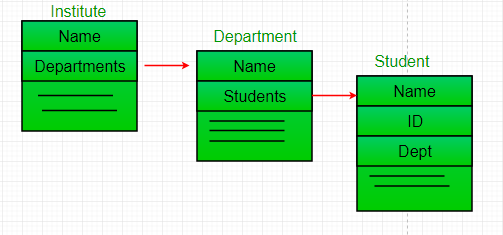
Note: Code reuse is best achieved by aggregation. Â
CompositionÂ
Composition is a restricted form of Aggregation in which two entities are highly dependent on each other. Â
- It represents part-of relationship.
- In composition, both entities are dependent on each other.
- When there is a composition between two entities, the composed object cannot exist without the other entity.
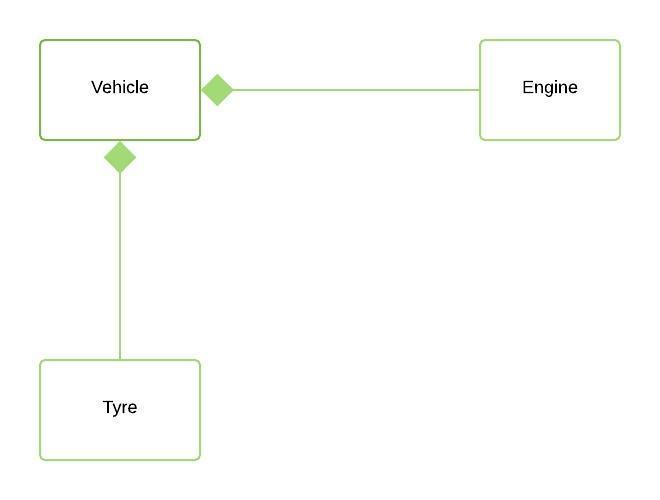
Composition Example:
Java
// Java program to illustrate
// Concept of Composition
// Importing required classes
import java.io.*;
import java.util.*;
// Class 1
// Department class
class Department {
// Attributes of Department
public String departmentName;
// Constructor of Department class
public Department(String departmentName)
{
this.departmentName = departmentName;
}
public String getDepartmentName()
{
return departmentName;
}
}
// Class 2
// Company class
class Company {
// Reference to refer to list of books
private String companyName;
private List<Department> departments;
// Constructor of Company class
public Company(String companyName)
{
this.companyName = companyName;
this.departments = new ArrayList<Department>();
}
// Method
// to add new Department to the Company
public void addDepartment(Department department)
{
departments.add(department);
}
public List<Department> getDepartments()
{
return new ArrayList<>(departments);
}
// Method
// to get total number of Departments in the Company
public int getTotalDepartments()
{
return departments.size();
}
}
// Class 3
// Main class
class CompositonExample {
// Main driver method
public static void main(String[] args)
{
// Creating a Company object
Company techCompany = new Company("Tech Corp");
techCompany.addDepartment(new Department("Engineering"));
techCompany.addDepartment(new Department("Operations"));
techCompany.addDepartment(new Department("Human Resources"));
techCompany.addDepartment(new Department("Finance"));
int d = techCompany.getTotalDepartments();
System.out.println("Total Departments: " + d);
System.out.println("Department names: ");
for (Department dept : techCompany.getDepartments()) {
System.out.println("- " + dept.getDepartmentName());
}
}
}
OutputTotal Departments: 4
Department names:
- Engineering
- Operations
- Human Resources
- Finance
Explanation of the above Program:
In the above example,
- A company can have no. of departments.
- All the departments are part-of the Company.
- So, if the Company gets destroyed then all the Departments within that particular Company will be destroyed, i.e. Departments can not exist independently without the Company.
- That’s why it is composition. Department is Part-of Company.
Aggregation vs CompositionÂ
- Dependency: Aggregation implies a relationship where the child can exist independently of the parent. For example, Bank and Employee, delete the Bank and the Employee still exist. whereas Composition implies a relationship where the child cannot exist independent of the parent. Example: Human and heart, heart don’t exist separate to a Human
- Type of Relationship: Aggregation represents “has-a” relationship whereas, Composition represents “part-of” relationship.
- Type of association: Composition is a strong Association whereas, Aggregation is a weak Association.
Example for Composition:
Java
// Java Program to Illustrate
// Composition
// Importing I/O classes
import java.io.*;
// Class 1
// Engine class which will be used by car.
class Engine {
// Method to start the engine
public void work()
{
// Print statement whenever this method is called
System.out.println(
"Engine of car has been started ");
}
}
// Class 2
// Car class
class Car {
// For a car to move,
// it needs to have an engine.
private final Engine engine;
// Constructor of this class
public Car()
{
this.engine = new Engine(); // Composition
}
// Method
// Car start moving by starting engine
public void move()
{
engine.work();
System.out.println("Car is moving");
}
}
// Class 3
// Main class
class CompositionCarExample {
// Main driver method
public static void main(String[] args)
{
// Creating a car object,
// which will also initializes the engine class object
Car car = new Car();
// Making car to move by calling
// move() method inside main()
car.move();
}
}
OutputEngine of car has been started
Car is moving
Explanation of the above Program:
In the above case of composition, the Car owns the Engine object (i.e. Engine cannot exist without the Car object).
Example for Aggregation:
Java
// Java Program to illustrate
// Aggregation
import java.io.*;
// Class 1
// Engine class
class Engine {
// attributes of Engine
private String engineType;
// Constructor
public Engine(String engineType) {
this.engineType = engineType;
}
public String getEngineType() {
return engineType;
}
// Method to start the engine
public void work()
{
// Print statement whenever this method is called
System.out.println("Engine of car has been started");
}
}
// Class 2
// Car class
class Car {
// For a car to move,
// it needs to have an engine.
private Engine engine;
// Constructor
public Car(Engine engine)
{
this.engine = engine; // Aggregation
}
// Method
// Car start moving by starting engine
public void move()
{
if (engine != null) {
engine.work();
System.out.println("Car is moving");
}
else {
System.out.println("Car cannot start without engine");
}
}
}
// Class 3
// Main class
class AggregationCarExample {
public static void main (String[] args) {
// Creating an independent Engine Object
Engine engine = new Engine("V10");
// Creating a Car object, by passing the engine
// such that, Car "has" Engine
Car car = new Car(engine);
car.move();
System.out.println("Engine Type: " + engine.getEngineType());
}
}
OutputEngine of car has been started
Car is moving
Engine Type: V10
Explanation of the above Program:
In the above case of aggregation, the the Engine object is independent of the Car object (i.e. Engine can exist without the Car object).
Share your thoughts in the comments
Please Login to comment...