Encapsulation in Java
Last Updated :
01 Nov, 2023
Encapsulation in Java is a fundamental concept in object-oriented programming (OOP) that refers to the bundling of data and methods that operate on that data within a single unit, which is called a class in Java. Java Encapsulation is a way of hiding the implementation details of a class from outside access and only exposing a public interface that can be used to interact with the class.
In Java, encapsulation is achieved by declaring the instance variables of a class as private, which means they can only be accessed within the class. To allow outside access to the instance variables, public methods called getters and setters are defined, which are used to retrieve and modify the values of the instance variables, respectively. By using getters and setters, the class can enforce its own data validation rules and ensure that its internal state remains consistent.
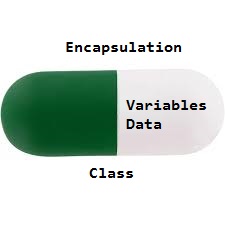
Implementation of Java Encapsulation
Below is the example with Java Encapsulation:
Java
class Person {
private String name;
private int age;
public String getName() { return name; }
public void setName(String name) { this .name = name; }
public int getAge() { return age; }
public void setAge( int age) { this .age = age; }
}
public class Main {
public static void main(String[] args)
{
Person person = new Person();
person.setName( "John" );
person.setAge( 30 );
System.out.println( "Name: " + person.getName());
System.out.println( "Age: " + person.getAge());
}
}
|
Output
Name: John
Age: 30
Encapsulation is defined as the wrapping up of data under a single unit. It is the mechanism that binds together code and the data it manipulates. Another way to think about encapsulation is, that it is a protective shield that prevents the data from being accessed by the code outside this shield.
- Technically in encapsulation, the variables or data of a class is hidden from any other class and can be accessed only through any member function of its own class in which it is declared.
- As in encapsulation, the data in a class is hidden from other classes using the data hiding concept which is achieved by making the members or methods of a class private, and the class is exposed to the end-user or the world without providing any details behind implementation using the abstraction concept, so it is also known as a combination of data-hiding and abstraction.
- Encapsulation can be achieved by Declaring all the variables in the class as private and writing public methods in the class to set and get the values of variables.
- It is more defined with the setter and getter method.
Advantages of Encapsulation
- Data Hiding: it is a way of restricting the access of our data members by hiding the implementation details. Encapsulation also provides a way for data hiding. The user will have no idea about the inner implementation of the class. It will not be visible to the user how the class is storing values in the variables. The user will only know that we are passing the values to a setter method and variables are getting initialized with that value.
- Increased Flexibility: We can make the variables of the class read-only or write-only depending on our requirements. If we wish to make the variables read-only then we have to omit the setter methods like setName(), setAge(), etc. from the above program or if we wish to make the variables write-only then we have to omit the get methods like getName(), getAge(), etc. from the above program
- Reusability: Encapsulation also improves the re-usability and is easy to change with new requirements.
- Testing code is easy: Encapsulated code is easy to test for unit testing.
- Freedom to programmer in implementing the details of the system: This is one of the major advantage of encapsulation that it gives the programmer freedom in implementing the details of a system. The only constraint on the programmer is to maintain the abstract interface that outsiders see.
For example: The Programmer of the edit menu code in a text-editor GUI might at first, implement the cut and paste operations by copying actual screen images in and out of an external buffer. Later, he/she may be dissatisfied with this implementation, since it does not allow compact storage of the selection, and it does not distinguish text and graphic objects. If the programmer has designed the cut-and-paste interface with encapsulation in mind, switching the underlying implementation to one that stores text as text and graphic objects in an appropriate compact format should not cause any problems to functions that need to interface with this GUI. Thus encapsulation yields adaptability, for it allows the implementation details of parts of a program to change without adversely affecting other parts.
Disadvantages of Encapsulation in Java
- Can lead to increased complexity, especially if not used properly.
- Can make it more difficult to understand how the system works.
- May limit the flexibility of the implementation.
Examples Showing Data Encapulation in Java
Example 1:
Below is the implementation of the above topic:
Java
class Area {
int length;
int breadth;
Area( int length, int breadth)
{
this .length = length;
this .breadth = breadth;
}
public void getArea()
{
int area = length * breadth;
System.out.println( "Area: " + area);
}
}
class Main {
public static void main(String[] args)
{
Area rectangle = new Area( 2 , 16 );
rectangle.getArea();
}
}
|
Example 2:
The program to access variables of the class EncapsulateDemo is shown below:
Java
class Encapsulate {
private String geekName;
private int geekRoll;
private int geekAge;
public int getAge() { return geekAge; }
public String getName() { return geekName; }
public int getRoll() { return geekRoll; }
public void setAge( int newAge) { geekAge = newAge; }
public void setName(String newName)
{
geekName = newName;
}
public void setRoll( int newRoll) { geekRoll = newRoll; }
}
public class TestEncapsulation {
public static void main(String[] args)
{
Encapsulate obj = new Encapsulate();
obj.setName( "Harsh" );
obj.setAge( 19 );
obj.setRoll( 51 );
System.out.println( "Geek's name: " + obj.getName());
System.out.println( "Geek's age: " + obj.getAge());
System.out.println( "Geek's roll: " + obj.getRoll());
}
}
|
Output
Geek's name: Harsh
Geek's age: 19
Geek's roll: 51
Example 3:
In the above program, the class Encapsulate is encapsulated as the variables are declared private. The get methods like getAge(), getName(), and getRoll() are set as public, these methods are used to access these variables. The setter methods like setName(), setAge(), setRoll() are also declared as public and are used to set the values of the variables.
Below is the implementation of the defined example:
Java
class Name {
private int age;
public int getAge() { return age; }
public void setAge( int age) { this .age = age; }
}
class GFG {
public static void main(String[] args)
{
Name n1 = new Name();
n1.setAge( 19 );
System.out.println( "The age of the person is: "
+ n1.getAge());
}
}
|
Output
The age of the person is: 19
Example 4:
Below is the implementation of the Java Encapsulation:
Java
class Account {
private long acc_no;
private String name, email;
private float amount;
public long getAcc_no() { return acc_no; }
public void setAcc_no( long acc_no)
{
this .acc_no = acc_no;
}
public String getName() { return name; }
public void setName(String name) { this .name = name; }
public String getEmail() { return email; }
public void setEmail(String email)
{
this .email = email;
}
public float getAmount() { return amount; }
public void setAmount( float amount)
{
this .amount = amount;
}
}
public class GFG {
public static void main(String[] args)
{
Account acc = new Account();
acc.setAcc_no(90482098491L);
acc.setName( "ABC" );
acc.setEmail( "abc@gmail.com" );
acc.setAmount(100000f);
System.out.println(
acc.getAcc_no() + " " + acc.getName() + " "
+ acc.getEmail() + " " + acc.getAmount());
}
}
|
Output
90482098491 ABC abc@gmail.com 100000.0
Share your thoughts in the comments
Please Login to comment...