Checked vs Unchecked Exceptions in Java
Last Updated :
07 May, 2023
In Java, Exception is an unwanted or unexpected event, which occurs during the execution of a program, i.e. at run time, that disrupts the normal flow of the program’s instructions.
In Java, there are two types of exceptions:
- Checked exceptions
- Unchecked exceptions
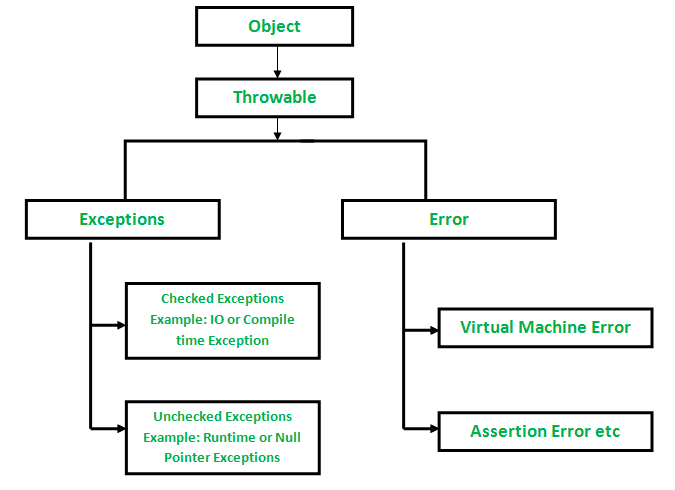
Checked Exceptions in Java
These are the exceptions that are checked at compile time. If some code within a method throws a checked exception, then the method must either handle the exception or it must specify the exception using the throws keyword. In checked exceptions, there are two types: fully checked and partially checked exceptions. A fully checked exception is a checked exception where all its child classes are also checked, like IOException, and InterruptedException. A partially checked exception is a checked exception where some of its child classes are unchecked, like an Exception.
For example, consider the following Java program that opens the file at location “C:\test\a.txt” and prints the first three lines of it. The program doesn’t compile, because the function main() uses FileReader(), and FileReader() throws a checked exception FileNotFoundException. It also uses readLine() and close() methods, and these methods also throw checked exception IOException
Example:
Java
import java.io.*;
class GFG {
public static void main(String[] args)
{
FileReader file = new FileReader( "C:\\test\\a.txt" );
BufferedReader fileInput = new BufferedReader(file);
for ( int counter = 0 ; counter < 3 ; counter++)
System.out.println(fileInput.readLine());
fileInput.close();
}
}
|
Output:
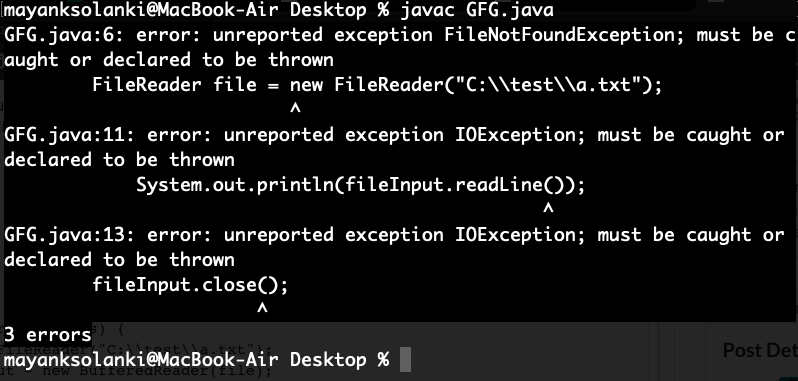
To fix the above program, we either need to specify a list of exceptions using throws, or we need to use a try-catch block. We have used throws in the below program. Since FileNotFoundException is a subclass of IOException, we can just specify IOException in the throws list and make the above program compiler-error-free.
Example:
Java
import java.io.*;
class GFG {
public static void main(String[] args)
throws IOException
{
FileReader file = new FileReader( "C:\\test\\a.txt" );
BufferedReader fileInput = new BufferedReader(file);
for ( int counter = 0 ; counter < 3 ; counter++)
System.out.println(fileInput.readLine());
fileInput.close();
}
}
|
Output:
First three lines of file "C:\test\a.txt"
Unchecked Exceptions in Java
These are the exceptions that are not checked at compile time. In C++, all exceptions are unchecked, so it is not forced by the compiler’s to either handle or specify the exception. It is up to the programmers to be civilized and specify or catch the exceptions. In Java, exceptions under Error and RuntimeException classes are unchecked exceptions, everything else under throwable is checked.
Consider the following Java program. It compiles fine, but it throws ArithmeticException when run. The compiler allows it to compile because ArithmeticException is an unchecked exception.
Example:
Java
class GFG {
public static void main(String args[])
{
int x = 0 ;
int y = 10 ;
int z = y / x;
}
}
|
Output
Exception in thread "main" java.lang.ArithmeticException: / by zero
at Main.main(Main.java:5)
Java Result: 1
In short unchecked exceptions are runtime exceptions that are not required to be caught or declared in a throws clause. These exceptions are usually caused by programming errors, such as attempting to access an index out of bounds in an array or attempting to divide by zero.
Unchecked exceptions include all subclasses of the RuntimeException class, as well as the Error class and its subclasses.
Here are some examples of unchecked exceptions in Java:
1. ArrayIndexOutOfBoundsException: This exception is thrown when you attempt to access an array index that is out of bounds.
2. NullPointerException: This exception is thrown when you attempt to access a null object reference.
3. ArithmeticException: This exception is thrown when you attempt to divide by zero or perform an invalid arithmetic operation.
Share your thoughts in the comments
Please Login to comment...