Java, Inheritance is an important pillar of OOP(Object-Oriented Programming). It is the mechanism in Java by which one class is allowed to inherit the features(fields and methods) of another class. In Java, Inheritance means creating new classes based on existing ones. A class that inherits from another class can reuse the methods and fields of that class. In addition, you can add new fields and methods to your current class as well.
Why Do We Need Java Inheritance?
- Code Reusability: The code written in the Superclass is common to all subclasses. Child classes can directly use the parent class code.
- Method Overriding: Method Overriding is achievable only through Inheritance. It is one of the ways by which Java achieves Run Time Polymorphism.
- Abstraction: The concept of abstract where we do not have to provide all details is achieved through inheritance. Abstraction only shows the functionality to the user.
Important Terminologies Used in Java Inheritance
- Class: Class is a set of objects which shares common characteristics/ behavior and common properties/ attributes. Class is not a real-world entity. It is just a template or blueprint or prototype from which objects are created.
- Super Class/Parent Class: The class whose features are inherited is known as a superclass(or a base class or a parent class).
- Sub Class/Child Class: The class that inherits the other class is known as a subclass(or a derived class, extended class, or child class). The subclass can add its own fields and methods in addition to the superclass fields and methods.
- Reusability: Inheritance supports the concept of “reusability”, i.e. when we want to create a new class and there is already a class that includes some of the code that we want, we can derive our new class from the existing class. By doing this, we are reusing the fields and methods of the existing class.
How to Use Inheritance in Java?
The extends keyword is used for inheritance in Java. Using the extends keyword indicates you are derived from an existing class. In other words, “extends” refers to increased functionality.
Syntax :
class DerivedClass extends BaseClass
{
//methods and fields
}
Inheritance in Java Example
Example: In the below example of inheritance, class Bicycle is a base class, class MountainBike is a derived class that extends the Bicycle class and class Test is a driver class to run the program.
Java
class Bicycle {
public int gear;
public int speed;
public Bicycle( int gear, int speed)
{
this .gear = gear;
this .speed = speed;
}
public void applyBrake( int decrement)
{
speed -= decrement;
}
public void speedUp( int increment)
{
speed += increment;
}
public String toString()
{
return ( "No of gears are " + gear + "\n"
+ "speed of bicycle is " + speed);
}
}
class MountainBike extends Bicycle {
public int seatHeight;
public MountainBike( int gear, int speed,
int startHeight)
{
super (gear, speed);
seatHeight = startHeight;
}
public void setHeight( int newValue)
{
seatHeight = newValue;
}
@Override public String toString()
{
return ( super .toString() + "\nseat height is "
+ seatHeight);
}
}
public class Test {
public static void main(String args[])
{
MountainBike mb = new MountainBike( 3 , 100 , 25 );
System.out.println(mb.toString());
}
}
|
Output
No of gears are 3
speed of bicycle is 100
seat height is 25
In the above program, when an object of MountainBike class is created, a copy of all methods and fields of the superclass acquires memory in this object. That is why by using the object of the subclass we can also access the members of a superclass.
Please note that during inheritance only the object of the subclass is created, not the superclass. For more, refer to Java Object Creation of Inherited Class.
Example 2: In the below example of inheritance, class Employee is a base class, class Engineer is a derived class that extends the Employee class and class Test is a driver class to run the program.
Java
import java.io.*;
class Employee {
int salary = 60000 ;
}
class Engineer extends Employee {
int benefits = 10000 ;
}
class Gfg {
public static void main(String args[])
{
Engineer E1 = new Engineer();
System.out.println( "Salary : " + E1.salary
+ "\nBenefits : " + E1.benefits);
}
}
|
Output
Salary : 60000
Benefits : 10000
Illustrative image of the program:
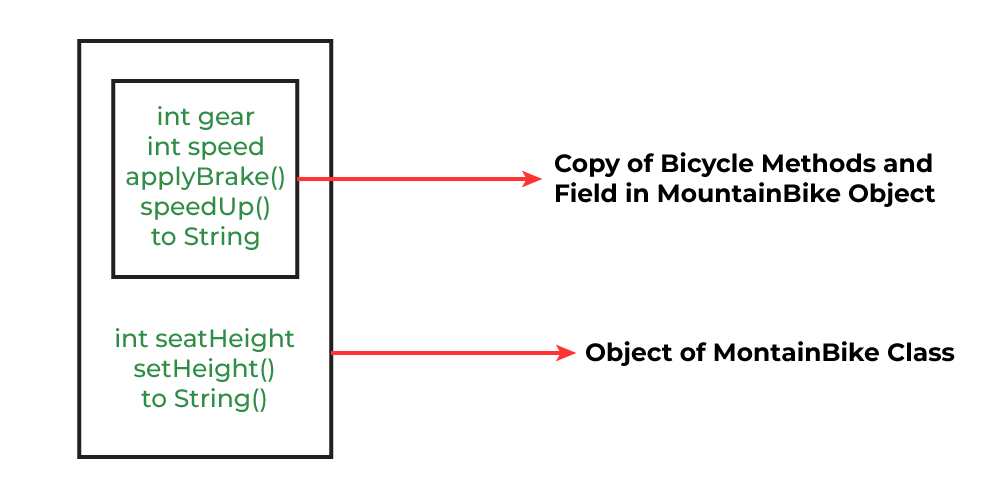
In practice, inheritance, and polymorphism are used together in Java to achieve fast performance and readability of code.
Java Inheritance Types
Below are the different types of inheritance which are supported by Java.
- Single Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
- Multiple Inheritance
- Hybrid Inheritance
1. Single Inheritance
In single inheritance, subclasses inherit the features of one superclass. In the image below, class A serves as a base class for the derived class B.
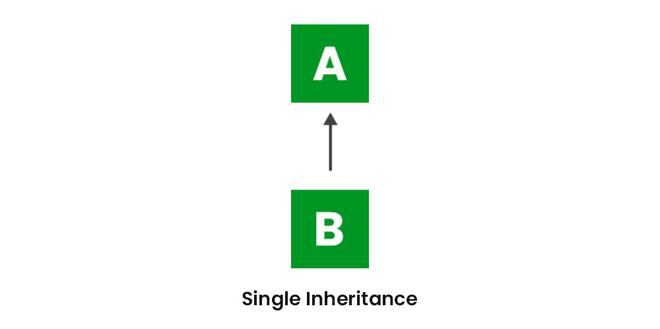
Single inheritance
Java
import java.io.*;
import java.lang.*;
import java.util.*;
class One {
public void print_geek()
{
System.out.println( "Geeks" );
}
}
class Two extends One {
public void print_for() { System.out.println( "for" ); }
}
public class Main {
public static void main(String[] args)
{
Two g = new Two();
g.print_geek();
g.print_for();
g.print_geek();
}
}
|
2. Multilevel Inheritance
In Multilevel Inheritance, a derived class will be inheriting a base class, and as well as the derived class also acts as the base class for other classes. In the below image, class A serves as a base class for the derived class B, which in turn serves as a base class for the derived class C. In Java, a class cannot directly access the grandparent’s members.
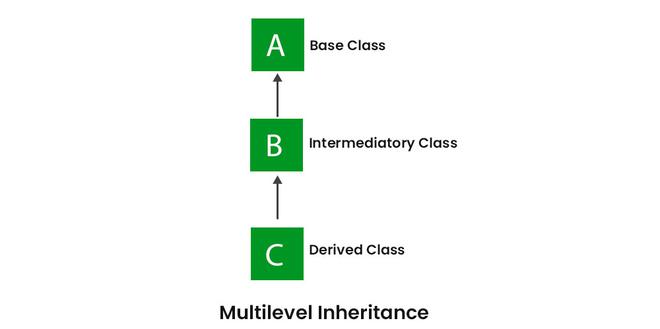
Multilevel Inheritance
Java
import java.io.*;
import java.lang.*;
import java.util.*;
class One {
public void print_geek()
{
System.out.println( "Geeks" );
}
}
class Two extends One {
public void print_for() { System.out.println( "for" ); }
}
class Three extends Two {
public void print_geek()
{
System.out.println( "Geeks" );
}
}
public class Main {
public static void main(String[] args)
{
Three g = new Three();
g.print_geek();
g.print_for();
g.print_geek();
}
}
|
3. Hierarchical Inheritance
In Hierarchical Inheritance, one class serves as a superclass (base class) for more than one subclass. In the below image, class A serves as a base class for the derived classes B, C, and D.
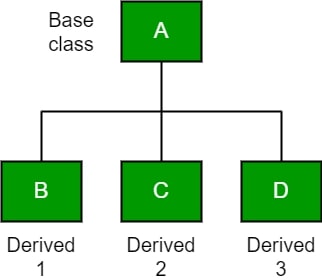
Java
class A {
public void print_A() { System.out.println( "Class A" ); }
}
class B extends A {
public void print_B() { System.out.println( "Class B" ); }
}
class C extends A {
public void print_C() { System.out.println( "Class C" ); }
}
class D extends A {
public void print_D() { System.out.println( "Class D" ); }
}
public class Test {
public static void main(String[] args)
{
B obj_B = new B();
obj_B.print_A();
obj_B.print_B();
C obj_C = new C();
obj_C.print_A();
obj_C.print_C();
D obj_D = new D();
obj_D.print_A();
obj_D.print_D();
}
}
|
Output
Class A
Class B
Class A
Class C
Class A
Class D
4. Multiple Inheritance (Through Interfaces)
In Multiple inheritances, one class can have more than one superclass and inherit features from all parent classes. Please note that Java does not support multiple inheritances with classes. In Java, we can achieve multiple inheritances only through Interfaces. In the image below, Class C is derived from interfaces A and B.
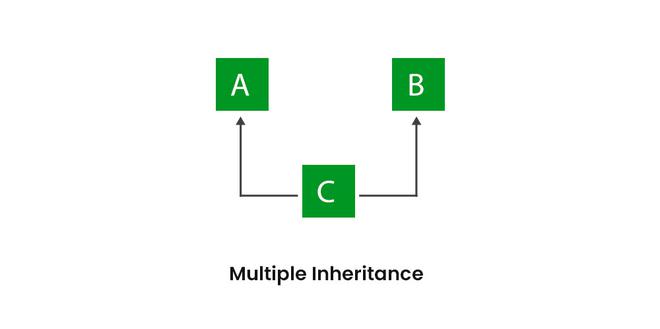
Multiple Inheritance
Java
import java.io.*;
import java.lang.*;
import java.util.*;
interface One {
public void print_geek();
}
interface Two {
public void print_for();
}
interface Three extends One, Two {
public void print_geek();
}
class Child implements Three {
@Override public void print_geek()
{
System.out.println( "Geeks" );
}
public void print_for() { System.out.println( "for" ); }
}
public class Main {
public static void main(String[] args)
{
Child c = new Child();
c.print_geek();
c.print_for();
c.print_geek();
}
}
|
5. Hybrid Inheritance
It is a mix of two or more of the above types of inheritance. Since Java doesn’t support multiple inheritances with classes, hybrid inheritance involving multiple inheritance is also not possible with classes. In Java, we can achieve hybrid inheritance only through Interfaces if we want to involve multiple inheritance to implement Hybrid inheritance.
However, it is important to note that Hybrid inheritance does not necessarily require the use of Multiple Inheritance exclusively. It can be achieved through a combination of Multilevel Inheritance and Hierarchical Inheritance with classes, Hierarchical and Single Inheritance with classes. Therefore, it is indeed possible to implement Hybrid inheritance using classes alone, without relying on multiple inheritance type.
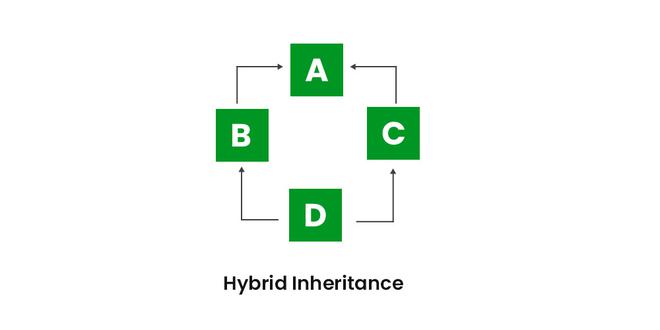
Hybrid Inheritance
Java IS-A type of Relationship
IS-A is a way of saying: This object is a type of that object. Let us see how the extends keyword is used to achieve inheritance.
Java
public class SolarSystem {
}
public class Earth extends SolarSystem {
}
public class Mars extends SolarSystem {
}
public class Moon extends Earth {
}
|
Now, based on the above example, in Object-Oriented terms, the following are true:-
- SolarSystem is the superclass of Earth class.
- SolarSystem is the superclass of Mars class.
- Earth and Mars are subclasses of SolarSystem class.
- Moon is the subclass of both Earth and SolarSystem classes.
Java
class SolarSystem {
}
class Earth extends SolarSystem {
}
class Mars extends SolarSystem {
}
public class Moon extends Earth {
public static void main(String args[])
{
SolarSystem s = new SolarSystem();
Earth e = new Earth();
Mars m = new Mars();
System.out.println(s instanceof SolarSystem);
System.out.println(e instanceof Earth);
System.out.println(m instanceof SolarSystem);
}
}
|
What Can Be Done in a Subclass?
In sub-classes we can inherit members as is, replace them, hide them, or supplement them with new members:
- The inherited fields can be used directly, just like any other fields.
- We can declare new fields in the subclass that are not in the superclass.
- The inherited methods can be used directly as they are.
- We can write a new instance method in the subclass that has the same signature as the one in the superclass, thus overriding it (as in the example above, toString() method is overridden).
- We can write a new static method in the subclass that has the same signature as the one in the superclass, thus hiding it.
- We can declare new methods in the subclass that are not in the superclass.
- We can write a subclass constructor that invokes the constructor of the superclass, either implicitly or by using the keyword super.
Advantages Of Inheritance in Java:
- Code Reusability: Inheritance allows for code reuse and reduces the amount of code that needs to be written. The subclass can reuse the properties and methods of the superclass, reducing duplication of code.
- Abstraction: Inheritance allows for the creation of abstract classes that define a common interface for a group of related classes. This promotes abstraction and encapsulation, making the code easier to maintain and extend.
- Class Hierarchy: Inheritance allows for the creation of a class hierarchy, which can be used to model real-world objects and their relationships.
- Polymorphism: Inheritance allows for polymorphism, which is the ability of an object to take on multiple forms. Subclasses can override the methods of the superclass, which allows them to change their behavior in different ways.
Disadvantages of Inheritance in Java:
- Complexity: Inheritance can make the code more complex and harder to understand. This is especially true if the inheritance hierarchy is deep or if multiple inheritances is used.
- Tight Coupling: Inheritance creates a tight coupling between the superclass and subclass, making it difficult to make changes to the superclass without affecting the subclass.
Conclusion
Let us check some important points from the article are mentioned below:
- Default superclass: Except Object class, which has no superclass, every class has one and only one direct superclass (single inheritance). In the absence of any other explicit superclass, every class is implicitly a subclass of the Object class.
- Superclass can only be one: A superclass can have any number of subclasses. But a subclass can have only one superclass. This is because Java does not support multiple inheritances with classes. Although with interfaces, multiple inheritances are supported by Java.
- Inheriting Constructors: A subclass inherits all the members (fields, methods, and nested classes) from its superclass. Constructors are not members, so they are not inherited by subclasses, but the constructor of the superclass can be invoked from the subclass.
- Private member inheritance: A subclass does not inherit the private members of its parent class. However, if the superclass has public or protected methods(like getters and setters) for accessing its private fields, these can also be used by the subclass.
FAQs in Inheritance
1. What is Inheritance Java?
Inheritance is a concept of OOPs where one class inherits from another class that can reuse the methods and fields of the parent class.
2. What are the 4 types of inheritance in Java?
There are Single, Multiple, Multilevel, Hierarchical and Hybrid
3. What is the use of extend keyword?
Extend keyword is used for inheriting one class into another.
4. What is an example of inheritance in Java?
A real-world example of Inheritance in Java is mentioned below:
Consider a group of vehicles. You need to create classes for Bus, Car, and Truck. The methods fuelAmount(), capacity(), applyBrakes() will be the same for all three classes.
References Used:
- “Head First Java” by Kathy Sierra and Bert Bates
- “Java: A Beginner’s Guide” by Herbert Schildt
- “Java: The Complete Reference” by Herbert Schildt
- “Effective Java” by Joshua Bloch
- “Java: The Good Parts” by Jim Waldo.
Share your thoughts in the comments
Please Login to comment...