Java and Multiple Inheritance
Last Updated :
16 Nov, 2022
Multiple Inheritance is a feature of an object-oriented concept, where a class can inherit properties of more than one parent class. The problem occurs when there exist methods with the same signature in both the superclasses and subclass. On calling the method, the compiler cannot determine which class method to be called and even on calling which class method gets the priority.
Note: Java doesn’t support Multiple Inheritance
Example 1:
Java
import java.io.*;
class Parent1 {
void fun() {
System.out.println( "Parent1" );
}
}
class Parent2 {
void fun() {
System.out.println( "Parent2" );
}
}
class Test extends Parent1, Parent2 {
public static void main(String args[]) {
Test t = new Test();
t.fun();
}
}
|
Output: Compilation error is thrown
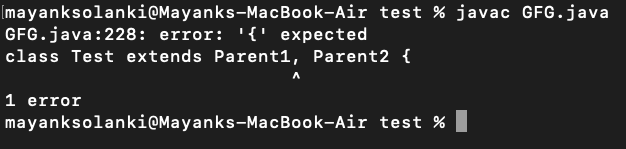
Conclusion: As depicted from code above, on calling the method fun() using Test object will cause complications such as whether to call Parent1’s fun() or Parent2’s fun() method.
Example 2:
GrandParent
/ \
/ \
Parent1 Parent2
\ /
\ /
Test
The code is as follows
Java
import java.io.*;
class GrandParent {
void fun() {
System.out.println( "Grandparent" );
}
}
class Parent1 extends GrandParent {
void fun() {
System.out.println( "Parent1" );
}
}
class Parent2 extends GrandParent {
void fun() {
System.out.println( "Parent2" );
}
}
class Test extends Parent1, Parent2 {
public static void main(String args[]) {
Test t = new Test();
t.fun();
}
}
|
Output:
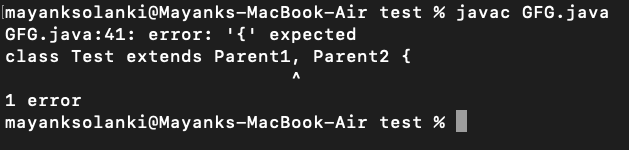
Again it throws compiler error when run fun() method as multiple inheritances cause a diamond problem when allowed in other languages like C++. From the code, we see that: On calling the method fun() using Test object will cause complications such as whether to call Parent1’s fun() or Parent2’s fun() method. Therefore, in order to avoid such complications, Java does not support multiple inheritances of classes.
Multiple inheritance is not supported by Java using classes, handling the complexity that causes due to multiple inheritances is very complex. It creates problems during various operations like casting, constructor chaining, etc, and the above all reason is that there are very few scenarios on which we actually need multiple inheritances, so better to omit it for keeping things simple and straightforward.
How are the above problems handled for Default Methods and Interfaces?
Java 8 supports default methods where interfaces can provide a default implementation of methods. And a class can implement two or more interfaces. In case both the implemented interfaces contain default methods with the same method signature, the implementing class should explicitly specify which default method is to be used in some method excluding the main() of implementing class using super keyword, or it should override the default method in the implementing class, or it should specify which default method is to be used in the default overridden method of the implementing class.
Example 3:
Java
interface PI1 {
default void show()
{
System.out.println( "Default PI1" );
}
}
interface PI2 {
default void show()
{
System.out.println( "Default PI2" );
}
}
class TestClass implements PI1, PI2 {
@Override
public void show()
{
PI1. super .show();
PI2. super .show();
}
public void showOfPI1() {
PI1. super .show();
}
public void showOfPI2() {
PI2. super .show();
}
public static void main(String args[])
{
TestClass d = new TestClass();
d.show();
System.out.println( "Now Executing showOfPI1() showOfPI2()" );
d.showOfPI1();
d.showOfPI2();
}
}
|
Output
Default PI1
Default PI2
Now Executing showOfPI1() showOfPI2()
Default PI1
Default PI2
Note: If we remove the implementation of default method from “TestClass”, we get a compiler error. If there is a diamond through interfaces, then there is no issue if none of the middle interfaces provide implementation of root interface. If they provide implementation, then implementation can be accessed as above using super keyword.
Example 4:
Java
interface GPI {
default void show()
{
System.out.println( "Default GPI" );
}
}
interface PI1 extends GPI {
}
interface PI2 extends GPI {
}
class TestClass implements PI1, PI2 {
public static void main(String args[])
{
TestClass d = new TestClass();
d.show();
}
}
|
Share your thoughts in the comments
Please Login to comment...