How are Java objects stored in memory?
Last Updated :
28 Dec, 2022
In Java, all objects are dynamically allocated on Heap. This is different from C++ where objects can be allocated memory either on Stack or on Heap. In JAVA , when we allocate the object using new(), the object is allocated on Heap, otherwise on Stack if not global or static.
In Java, when we only declare a variable of a class type, only a reference is created (memory is not allocated for the object). To allocate memory to an object, we must use new(). So the object is always allocated memory on the heap (See this for more details).
There are two ways to create an object of string in java:
- By string literal
- By new keyword
i) By string literal:
This is done using double-quotes.
For example:
String str1="GFG";
String str2="GFG";
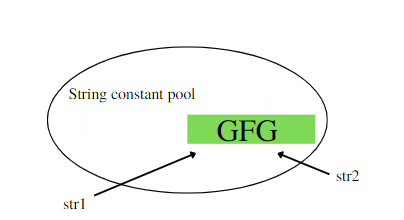
By String Literal
Every time when a string literal is created, JVM will check whether that string already exists in the string constant pool or not. If the string already exists in the string literal pool then a reference to the pooled instance is returned. If the string does not exist, then a new string instance is created in the pool. Hence, only one object will get created.
Here, the JVM is not bonded to create a new memory.
ii) By new keyword:
This is done using a new keyword.
For example:
String str1=new String("GFG");
String str2=new String("GFG");
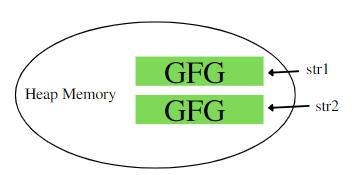
By new Keyword
Both str1 and str2 are objects of String.
Every time when a string object is created, JVM will create it in a heap memory. In this case, the JVM will not check whether the string already exists or not. If a string already exist , then also for every string object the memory will get created separately.
Here, the JVM is bond to create a new memory. For example, the following program fails in the compilation. Compiler gives error “Error here because t is not initialized”.
java
class Test {
void show()
{
System.out.println( "Test::show() called" );
}
}
public class Main {
public static void main(String[] args)
{
Test t;
t.show();
}
}
|
Output:
Allocating memory using new() makes the above program work.
java
class Test {
void show()
{
System.out.println( "Test::show() called" );
}
}
public class Main {
public static void main(String[] args)
{
Test t = new Test();
t.show();
}
}
|
Output
Test::show() called
Share your thoughts in the comments
Please Login to comment...