Object Class in Java
Last Updated :
06 Mar, 2024
Object class is present in java.lang package. Every class in Java is directly or indirectly derived from the Object class. If a class does not extend any other class then it is a direct child class of Object and if extends another class then it is indirectly derived. Therefore the Object class methods are available to all Java classes. Hence Object class acts as a root of the inheritance hierarchy in any Java Program.
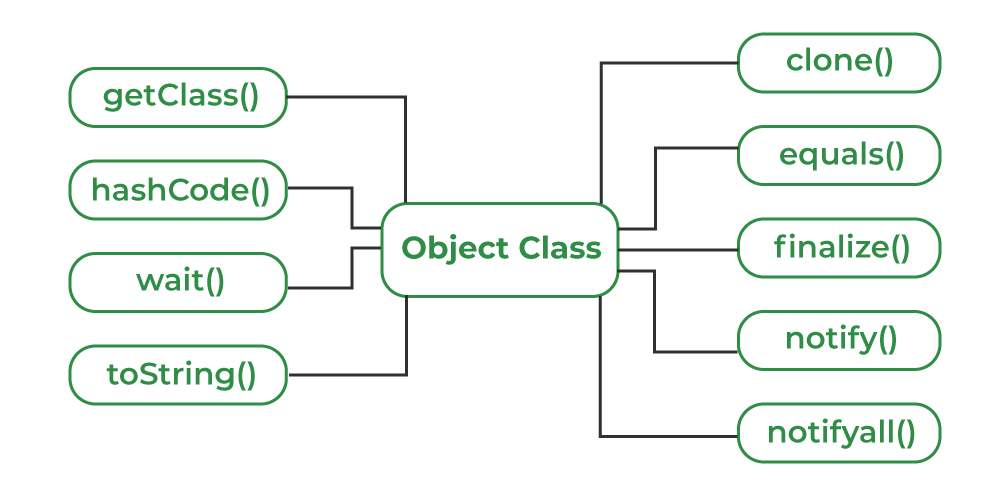
Using Object Class Methods
The Object class provides multiple methods which are as follows:
- toString() method
- hashCode() method
- equals(Object obj) method
- finalize() method
- getClass() method
- clone() method
- wait(), notify() notifyAll() methods
1. toString() method
The toString() provides a String representation of an object and is used to convert an object to a String. The default toString() method for class Object returns a string consisting of the name of the class of which the object is an instance, the at-sign character `@’, and the unsigned hexadecimal representation of the hash code of the object. In other words, it is defined as:
// Default behavior of toString() is to print class name, then
// @, then unsigned hexadecimal representation of the hash code
// of the object
public String toString()
{
return getClass().getName() + "@" + Integer.toHexString(hashCode());
}
It is always recommended to override the toString() method to get our own String representation of Object. For more on override of toString() method refer – Overriding toString() in JavaÂ
Note: Whenever we try to print any Object reference, then internally toString() method is called.
Student s = new Student();
// Below two statements are equivalent
System.out.println(s);
System.out.println(s.toString());
2. hashCode() method
For every object, JVM generates a unique number which is a hashcode. It returns distinct integers for distinct objects. A common misconception about this method is that the hashCode() method returns the address of the object, which is not correct. It converts the internal address of the object to an integer by using an algorithm. The hashCode() method is native because in Java it is impossible to find the address of an object, so it uses native languages like C/C++ to find the address of the object.
Use of hashCode() method
It returns a hash value that is used to search objects in a collection. JVM(Java Virtual Machine) uses the hashcode method while saving objects into hashing-related data structures like HashSet, HashMap, Hashtable, etc. The main advantage of saving objects based on hash code is that searching becomes easy.Â
Note: Override of hashCode() method needs to be done such that for every object we generate a unique number. For example, for a Student class, we can return the roll no. of a student from the hashCode() method as it is unique.Â
Java
public class Student {
static int last_roll = 100 ;
int roll_no;
Student()
{
roll_no = last_roll;
last_roll++;
}
@Override public int hashCode() { return roll_no; }
public static void main(String args[])
{
Student s = new Student();
System.out.println(s);
System.out.println(s.toString());
}
}
|
Output :
Student@64
Student@64
Note that 4*160 + 6*161 = 100Â
3. equals(Object obj) method
It compares the given object to “this” object (the object on which the method is called). It gives a generic way to compare objects for equality. It is recommended to override the equals(Object obj) method to get our own equality condition on Objects. For more on the override of equals(Object obj) method refer – Overriding equals method in Java
Note: It is generally necessary to override the hashCode() method whenever this method is overridden, so as to maintain the general contract for the hashCode method, which states that equal objects must have equal hash codes.Â
4. getClass() method
It returns the class object of “this” object and is used to get the actual runtime class of the object. It can also be used to get metadata of this class. The returned Class object is the object that is locked by static synchronized methods of the represented class. As it is final so we don’t override it.
Java
public class Test {
public static void main(String[] args)
{
Object obj = new String( "GeeksForGeeks" );
Class c = obj.getClass();
System.out.println( "Class of Object obj is : "
+ c.getName());
}
}
|
Output:Â
Class of Object obj is : java.lang.String
Note: After loading a .class file, JVM will create an object of the type java.lang.Class in the Heap area. We can use this class object to get Class level information. It is widely used in ReflectionÂ
5. finalize() method
This method is called just before an object is garbage collected. It is called the Garbage Collector on an object when the garbage collector determines that there are no more references to the object. We should override finalize() method to dispose of system resources, perform clean-up activities and minimize memory leaks. For example, before destroying the Servlet objects web container, always called finalize method to perform clean-up activities of the session.Â
Note: The finalize method is called just once on an object even though that object is eligible for garbage collection multiple times.Â
Java
public class Test {
public static void main(String[] args)
{
Test t = new Test();
System.out.println(t.hashCode());
t = null ;
System.gc();
System.out.println( "end" );
}
@Override protected void finalize()
{
System.out.println( "finalize method called" );
}
}
|
Output:Â
1510467688
finalize method called
end
6. clone() methodÂ
It returns a new object that is exactly the same as this object. For clone() method refer Clone().
The remaining three methods wait(), notify() notifyAll() are related to Concurrency. Refer to Inter-thread Communication in Java for details.
Example of using all the Object class methods in Java
Java
import java.io.*;
public class Book implements Cloneable {
private String title;
private String author;
private int year;
public Book(String title, String author, int year)
{
this .title = title;
this .author = author;
this .year = year;
}
@Override public String toString()
{
return title + " by " + author + " (" + year + ")" ;
}
@Override public boolean equals(Object obj)
{
if (obj == null || !(obj instanceof Book)) {
return false ;
}
Book other = (Book)obj;
return this .title.equals(other.getTitle())
&& this .author.equals(other.getAuthor())
&& this .year == other.getYear();
}
@Override public int hashCode()
{
int result = 17 ;
result = 31 * result + title.hashCode();
result = 31 * result + author.hashCode();
result = 31 * result + year;
return result;
}
@Override public Book clone()
{
try {
return (Book) super .clone();
}
catch (CloneNotSupportedException e) {
throw new AssertionError();
}
}
@Override protected void finalize() throws Throwable
{
System.out.println( "Finalizing " + this );
}
public String getTitle() { return title; }
public String getAuthor() { return author; }
public int getYear() { return year; }
public static void main(String[] args)
{
Book book1 = new Book(
"The Hitchhiker's Guide to the Galaxy" ,
"Douglas Adams" , 1979 );
System.out.println(book1);
Book book2 = book1.clone();
System.out.println(book2);
System.out.println( "book1 equals book2: "
+ book1.equals(book2));
System.out.println( "book1 hash code: "
+ book1.hashCode());
System.out.println( "book2 hash code: "
+ book2.hashCode());
book1 = null ;
System.gc();
}
}
|
Output
The Hitchhiker's Guide to the Galaxy by Douglas Adams (1979)
The Hitchhiker's Guide to the Galaxy by Douglas Adams (1979)
book1 equals book2: true
book1 hash code: 1840214527
book2 hash code: 1840214527
Share your thoughts in the comments
Please Login to comment...