How to Convert JSON Array Object to Java Object?
Last Updated :
06 Feb, 2024
JSON arrays are useful for storing multiple values together that are related or part of the same set. For example, storing a list of items, user profiles, product catalog, etc. JSON arrays allow ordered access to the values using indices like in regular arrays in other languages (0 indexed).
Conversion Methods:
- Using a JSON parsing library: Jackson & GSON
- Iterating and parsing manually.
Steps to Convert JSON Array Object to Java Object
Below are the steps and implementation to convert JSON Array object to Java object using Jackson library.
Step 1: Create a Maven Project
Open any preferred IDE and create a new Maven project. Here we will be using IntelliJ IDEA, we can do this by selecting File -> New -> Project.. -> Maven and following the wizard.
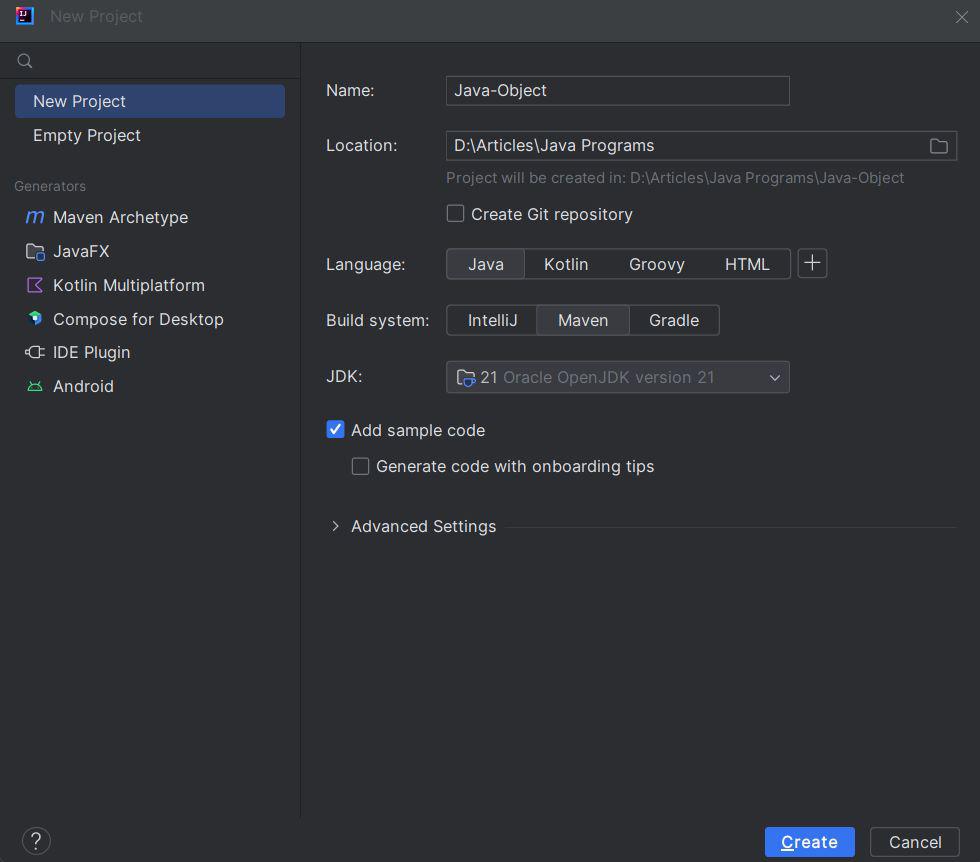
Step 2: Add Jackson Dependency to POM.xml
Now, we will add Jackson dependency to the pom.xml file.
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
< modelVersion >4.0.0</ modelVersion >
< groupId >org.example</ groupId >
< artifactId >Java-Object</ artifactId >
< version >1.0-SNAPSHOT</ version >
< dependencies >
< dependency >
< groupId >com.fasterxml.jackson.core</ groupId >
< artifactId >jackson-databind</ artifactId >
< version >2.13.0</ version >
</ dependency >
</ dependencies >
< properties >
< maven.compiler.source >21</ maven.compiler.source >
< maven.compiler.target >21</ maven.compiler.target >
< project.build.sourceEncoding >UTF-8</ project.build.sourceEncoding >
</ properties >
</ project >
|
Step 3: Create the Java POJO Class
The User class defines a POJO to map JSON data with name and age properties. Getters and setters are provided for Jackson to populate objects.
User.java
Java
package org.example;
public class User
{
private String firstName;
private String lastName;
private String email;
public User() {}
public User(String firstName, String lastName,
String email)
{
this .firstName = firstName;
this .lastName = lastName;
this .email = email;
}
public String getFirstName()
{
return firstName;
}
public void setFirstName(String firstName)
{
this .firstName = firstName;
}
public String getLastName()
{
return lastName;
}
public void setLastName(String lastName)
{
this .lastName = lastName;
}
public String getEmail()
{
return email;
}
public void setEmail(String email)
{
this .email = email;
}
}
|
Step 4: In the Main class add logic of Conversion JSON Array Object to Java Object
Now, we will add the logic to convert JSON Array Object to Java Object in the main class.
Java
package org.example;
import com.fasterxml.jackson.databind.ObjectMapper;
public class Main {
public static void main(String[] args)
{
try {
String jsonString = "[{\"firstName\":\"User1\",\"lastName\":\"XYZ\",\"email\":\"user1@example.com\"},"
+ "{\"firstName\":\"User2\",\"lastName\":\"PQR\",\"email\":\"user2@example.com\"}]" ;
ObjectMapper objectMapper = new ObjectMapper();
User[] users = objectMapper.readValue(jsonString, User[]. class );
for (User user : users) {
System.out.println(
"First Name: " + user.getFirstName()
+ ", Last Name: " + user.getLastName()
+ ", Email: " + user.getEmail());
}
}
catch (Exception e) {
e.printStackTrace();
}
}
}
|
Output:
First Name: User1, Last Name: XYZ, Email: user1@example.com
First Name: User2, Last Name: PQR, Email: user2@example.com
Explanation of the Code:
- In the above code, we have a JSON string
jsonString
representing an array of user objects.
- We have created an
ObjectMapper
instance from the Jackson library, which helps with JSON processing.
- We have used the
readValue()
method of the ObjectMapper
to convert the JSON string into an array of User
objects. We specify the type of the target array using User class
.
- Then we iterate over the array of
User
objects and print the details of each user.
Share your thoughts in the comments
Please Login to comment...