How to Change Colors for a Button in React-Bootstrap?
Last Updated :
01 Nov, 2023
In this tutorial, we will learn how to change the colors of buttons in React-Bootstrap. The Button component in React-Bootstrap is a simple button with CSS and the effects of Boostrap. We can apply different colors based on the different situations like Error, Success, etc.
Steps to create the application:
- Create a new react project:
npx create-react-app change-button-color
- Navigate to the project and install react-bootstrap:
cd change-button-color
npm install react-bootstrap bootstrap
- Add the bootstrap stylesheet CSS to the in the index.js file:
import "bootstrap/dist/css/bootstrap.min.css";
Project Structure:
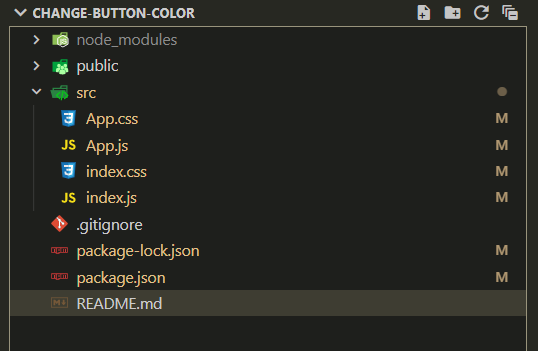
Step to Run Application:
Run the application using the following command from the root directory of the project:
npm start
Example 1: Using theme colors, modify the button color
App.js
Javascript
import { Button } from "react-bootstrap" ;
import "./App.css" ;
function App() {
return (
<div className= "App" >
<h1 className= "text-center" >
Welcome to GeeksforGeeks
</h1>
<h3 className= "text-center mt-5" >
How to change colors for a
button in React-Bootstrap
</h3>
<div className= "container mt-5 d-flex
justify-content-center" >
<Button
onClick={() => {
alert( "Welcome to GeeksforGeeks
Primary Button" );
}}
className= "m-4"
variant= "primary" >
Primary
</Button>
<Button
onClick={() => {
alert( "Welcome to GeeksforGeeks
Success Button" );
}}
className= "m-4"
variant= "success" >
Success
</Button>
<Button
onClick={() => {
alert( "Welcome to GeeksforGeeks
Warning Button" );
}}
className= "m-4"
variant= "warning" >
Warning
</Button>
<Button
onClick={() => {
alert( "Welcome to GeeksforGeeks
Danger Button" );
}}
className= "m-4"
variant= "danger" >
Danger
</Button>
</div>
</div>
);
}
export default App;
|
Output:
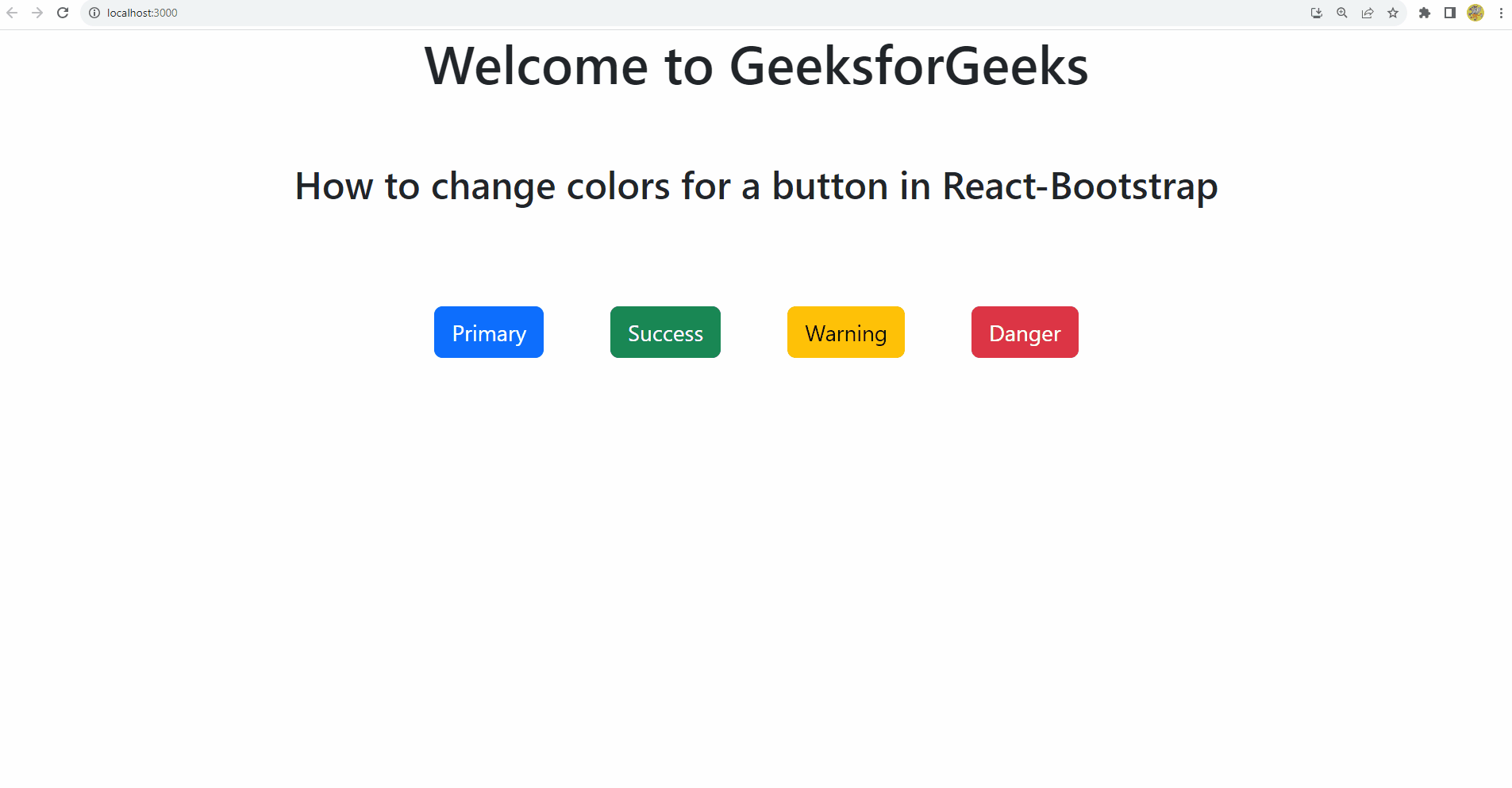
Example 2: Using style properties to modify button color
Javascript
import { Button } from "react-bootstrap" ;
import "./App.css" ;
function App() {
return (
<div className= "App" >
<h1 className= "text-center" >
Welcome to GeeksforGeeks
</h1>
<h3 className= "text-center mt-5" >
How to change colors for a
button in React-Bootstrap
</h3>
<div className= "container mt-5 d-flex
justify-content-center" >
<Button
onClick={() => {
alert( "Welcome to GeeksforGeeks Pink Button" );
}}
className= "m-4"
style={
{ backgroundColor: "#FF1493" ,
borderColor: "#FF1493" }}>
Pink
</Button>
<Button
onClick={() => {
alert(
"Welcome to GeeksforGeeks
Light Green Button" );
}}
className= "m-4"
style={
{ backgroundColor: "#32CD32" ,
borderColor: "#32CD32" }}>
Light Green
</Button>
<Button
onClick={() => {
alert(
"Welcome to GeeksforGeeks
Deep Green Button" );
}}
className= "m-4"
style={
{ backgroundColor: "#149403" ,
borderColor: "#149403" }}>
Deep Green
</Button>
<Button
onClick={() => {
alert(
"Welcome to GeeksforGeeks
Deep Blue Button" );
}}
className= "m-4"
style={
{ backgroundColor: "#11077d" ,
borderColor: "#11077d" }}>
Deep Blue
</Button>
</div>
</div>
);
}
export default App;
|
Output:
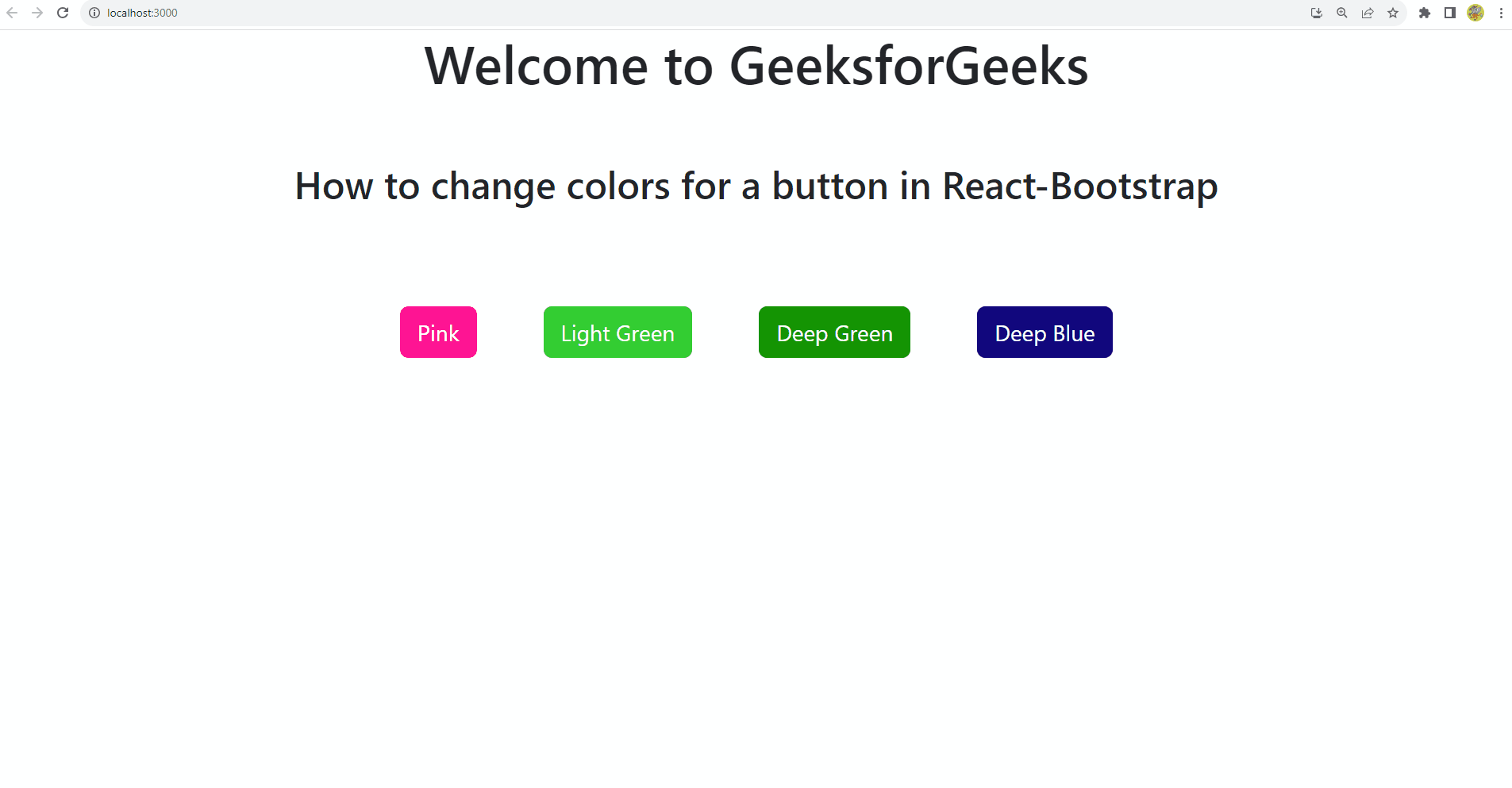
Share your thoughts in the comments
Please Login to comment...