Different Ways To Declare And Initialize 2-D Array in Java
Last Updated :
16 Feb, 2023
An array with more than one dimension is known as a multi-dimensional array. The most commonly used multi-dimensional arrays are 2-D and 3-D arrays. We can say that any higher dimensional array is basically an array of arrays. A very common example of a 2D Array is Chess Board. A chessboard is a grid containing 64 1×1 square boxes. You can similarly visualize a 2D array. In a 2D array, every element is associated with a row number and column number. Accessing any element of the 2D array is similar to accessing the record of an Excel File using both row number and column number. 2D arrays are useful while implementing a Tic-Tac-Toe game, Chess, or even storing the image pixels.
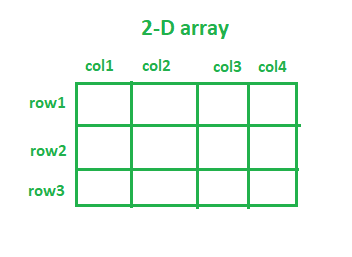
Declaring 2-D array in Java:
Any 2-dimensional array can be declared as follows:
Syntax:
data_type array_name[][]; (OR) data_type[][] array_name;
- data_type: Since Java is a statically-typed language (i.e. it expects its variables to be declared before they can be assigned values). So, specifying the datatype decides the type of elements it will accept. e.g. to store integer values only, the data type will be declared as int.
- array_name: It is the name that is given to the 2-D array. e.g. subjects, students, fruits, department, etc.
Note: We can write [ ][ ] after data_type or we can write [ ][ ] after array_name while declaring the 2D array.
Java
import java.io.*;
class GFG {
public static void main(String[] args)
{
int [][] integer2DArray;
String[][] string2DArray;
double [][] double2DArray;
boolean [][] boolean2DArray;
float [][] float2DArray;
double [][] double2DArray;
}
}
|
Different approaches for Initialization of 2-D array in Java:
data_type[][] array_Name = new data_type[no_of_rows][no_of_columns];
The total elements in any 2D array will be equal to (no_of_rows) * (no_of_columns).
- no_of_rows: The number of rows in an array. e.g. no_of_rows = 3, then the array will have three rows.
- no_of_columns: The number of columns in an array. e.g. no_of_columns = 4, then the array will have four columns.
The above syntax of array initialization will assign default values to all array elements according to the data type specified.
Below is the implementation of various approaches for initializing 2D arrays:
Approach 1:
Java
import java.io.*;
class GFG {
public static void main(String[] args)
{
int [][] integer2DArray = new int [ 5 ][ 3 ];
System.out.println(
"Default value of int array element: "
+ integer2DArray[ 0 ][ 0 ]);
String[][] string2DArray = new String[ 4 ][ 4 ];
System.out.println(
"Default value of String array element: "
+ string2DArray[ 0 ][ 0 ]);
boolean [][] boolean2DArray = new boolean [ 4 ][ 4 ];
System.out.println(
"Default value of boolean array element: "
+ boolean2DArray[ 0 ][ 0 ]);
char [][] char2DArray = new char [ 10 ][ 10 ];
System.out.println(
"Default value of char array element: "
+ char2DArray[ 0 ][ 0 ]);
int [][] arr;
arr = new int [ 5 ][ 3 ];
System.out.println( "arr[0][0]: " + arr[ 0 ][ 0 ]);
}
}
|
Note: When you initialize a 2D array, you must always specify the first dimension(no. of rows), but providing the second dimension(no. of columns) may be omitted.
In the code snippet below, we have not specified the number of columns. However, the Java compiler is smart enough to manipulate the size by checking the number of elements inside the columns.
Java
import java.io.*;
class GFG {
public static void main(String[] args)
{
int [][] arr = new int [][ 3 ];
int [][] arr = new int [ 2 ][];
}
}
|
You can access any element of a 2D array using row numbers and column numbers.
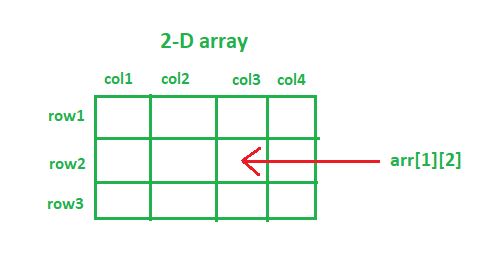
Approach 2:
In the code snippet below, we have not specified the number of rows and columns. However, the Java compiler is smart enough to manipulate the size by checking the number of elements inside the rows and columns.
Java
import java.io.*;
class GFG {
public static void main(String[] args)
{
String[][] subjects = {
{ "Data Structures & Algorithms" ,
"Programming & Logic" , "Software Engineering" ,
"Theory of Computation" },
{ "Thermodynamics" , "Metallurgy" ,
"Machine Drawing" ,
"Fluid Mechanics" },
{ "Signals and Systems" , "Digital Electronics" ,
"Power Electronics" }
};
System.out.println(
"Fundamental Subject in Computer Engineering: "
+ subjects[ 0 ][ 0 ]);
System.out.println(
"Fundamental Subject in Mechanical Engineering: "
+ subjects[ 1 ][ 3 ]);
System.out.println(
"Fundamental Subject in Electronics Engineering: "
+ subjects[ 2 ][ 1 ]);
}
}
|
Output
Fundamental Subject in Computer Engineering: Data Structures & Algorithms
Fundamental Subject in Mechanical Engineering: Fluid Mechanics
Fundamental Subject in Electronics Engineering: Digital Electronics
Approach 3:
Moreover, we can initialize each element of the array separately. Look at the code snippet below:
Java
import java.io.*;
import java.util.*;
class GFG {
public static void main(String[] args)
{
int [][] scores = new int [ 2 ][ 2 ];
scores[ 0 ][ 0 ] = 15 ;
scores[ 0 ][ 1 ] = 23 ;
scores[ 1 ][ 0 ] = 30 ;
scores[ 1 ][ 1 ] = 21 ;
System.out.println( "scores[0][0] = "
+ scores[ 0 ][ 0 ]);
System.out.println( "scores[0][1] = "
+ scores[ 0 ][ 1 ]);
System.out.println( "scores[1][0] = "
+ scores[ 1 ][ 0 ]);
System.out.println( "scores[1][1] = "
+ scores[ 1 ][ 1 ]);
System.out.println(
"Printing 2D array using Arrays.deepToString() method: " );
System.out.println(Arrays.deepToString(scores));
}
}
|
Output
scores[0][0] = 15
scores[0][1] = 23
scores[1][0] = 30
scores[1][1] = 21
Printing 2D array using Arrays.deepToString() method:
[[15, 23], [30, 21]]
Approach 4
Using the above approach for array initialization would be a tedious task if the size of the 2D array is too large. The efficient way is to use for loop for initializing the array elements in the case of a large 2D array.
Java
import java.io.*;
class GFG {
public static void main(String[] args)
{
int rows = 80 , columns = 5 ;
int [][] marks = new int [rows][columns];
for ( int i = 0 ; i < rows; i++) {
for ( int j = 0 ; j < columns; j++) {
marks[i][j] = i + j;
}
}
System.out.println( "First three rows are: " );
for ( int i = 0 ; i < 3 ; i++) {
for ( int j = 0 ; j < columns; j++) {
System.out.printf(marks[i][j] + " " );
}
System.out.println();
}
}
}
|
Output
First three rows are:
0 1 2 3 4
1 2 3 4 5
2 3 4 5 6
Note: We can use arr. length function to find the size of the rows (1st dimension), and arr[0].length function to find the size of the columns (2nd dimension).
Approach 5: (Jagged arrays)
There may be a certain scenario where you want every row to have a different number of columns. This type of array is called a Jagged Array.
Java
import java.io.*;
class GFG {
public static void main(String[] args)
{
int jagged[][] = new int [ 2 ][];
jagged[ 0 ] = new int [ 2 ];
jagged[ 1 ] = new int [ 4 ];
int count = 0 ;
for ( int i = 0 ; i < jagged.length; i++) {
for ( int j = 0 ; j < jagged[i].length; j++) {
jagged[i][j] = count++;
}
}
System.out.println( "The values of 2D jagged array" );
for ( int i = 0 ; i < jagged.length; i++) {
for ( int j = 0 ; j < jagged[i].length; j++)
System.out.printf(jagged[i][j] + " " );
System.out.println();
}
}
}
|
Output
The values of 2D jagged array
0 1
2 3 4 5
Program to add two 2D arrays:
Java
import java.io.*;
import java.util.*;
class GFG {
public static void main(String[] args)
{
int [][] arr1 = { { 1 , 2 , 3 }, { 4 , 5 , 6 } };
int [][] arr2 = { { 4 , 5 , 6 }, { 1 , 3 , 2 } };
int [][] sum = new int [ 2 ][ 3 ];
for ( int i = 0 ; i < arr1.length; i++) {
for ( int j = 0 ; j < arr1[ 0 ].length; j++) {
sum[i][j] = arr1[i][j] + arr2[i][j];
}
}
System.out.println( "Resultant 2D array: " );
for ( int i = 0 ; i < sum.length; i++) {
System.out.println(Arrays.toString(sum[i]));
}
}
}
|
Output
Resultant 2D array:
[5, 7, 9]
[5, 8, 8]
Share your thoughts in the comments
Please Login to comment...