Welcome to the Competitive Programming Handbook or CP Handbook by GeeksforGeeks! This Competitive Programming Handbook is a go-to resource for individuals aiming to enhance their problem-solving skills and excel in coding competitions. This CP handbook provides a comprehensive guide, covering fundamental concepts, advanced algorithms, and proven strategies to succeed in the field of competitive programming.
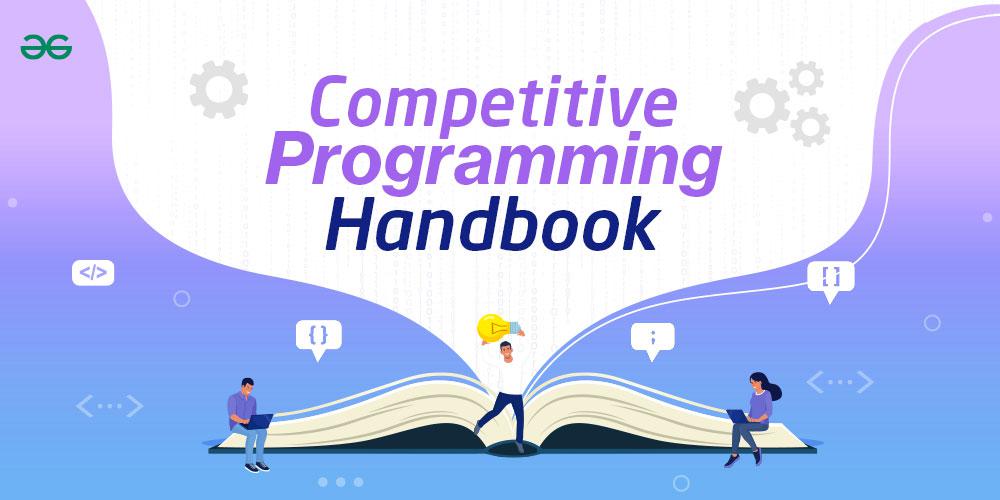
Competitive Programming (CP) Handbook
Whether you’re a novice or an expert coder, this competitive programming handbook offers valuable insights and practical applications to sharpen your skills and navigate the challenges of coding competitions effectively.
Competitive Programming Handbook || Chapter 1 | Number Theory
Number Theory is important for competitive programming because it provides essential mathematical tools to efficiently solve problems related to integers, primes, divisibility, and modular arithmetic. It enables programmers to optimize algorithms, handle mathematical operations with integers, and design efficient solutions for a wide range of computational problems.
Following is a list of important topics relevant to Number Theory:
Competitive Programming Handbook || Chapter 2 | Bit Manipulation
Bit Manipulation is crucial in competitive programming because it allows for efficient handling of binary representations of numbers. It enables compact storage, faster operations, and advanced techniques, optimizing code for performance, and reducing time and space complexity.
Following is a list of important topics relevant to Bit Manipulation:
Competitive Programming Handbook || Chapter 3 | Combinatorics
Combinatorics is crucial for competitive programming because it deals with counting and arranging elements, providing essential techniques for solving problems involving permutations, combinations, and counting principles. It enables efficient algorithm design and optimization, essential for solving complex problems within the time constraints of competitive programming.
Following is a list of important topics relevant to Combinatorics:
Competitive Programming Handbook || Chapter 4 | Advance Mathematics
Advance Mathematics is the key to mastering competitive programming. Whether it’s using smart number tricks or strategic problem-solving techniques, these advanced concepts give you the tools to tackle challenges with precision.
Following is a list of important topics relevant to Advance Mathematics:
Competitive Programming Handbook || Chapter 5 | Greedy Algorithms
Greedy algorithms are crucial in competitive programming because they provide efficient and often simple solutions by making locally optimal choices at each stage. Their intuitive nature allows for quick implementation, making them valuable for solving a wide range of optimization problems under time constraints.
Following is a list of important topics relevant to Greedy Algorithms:
Competitive Programming Handbook || Chapter 6 | Searching Techniques
Searching is crucial in competitive programming because it allows efficient retrieval of information from a dataset. It enables quick identification of elements, reducing time complexity and improving overall algorithm performance. Efficient search algorithms are essential for solving problems within the time constraints of competitive programming competitions.
Following is a list of important topics relevant to Searching Techniques:
Competitive Programming Handbook || Chapter 7 | Must know Data Structures
Data structures are essential for competitive programming because they enable efficient organization and manipulation of data, optimizing algorithms and solutions for speed and memory usage.
Following is a list of important topics relevant to Data Structures:
Competitive Programming Handbook || Chapter 8 | Pre-Computation
Pre-computation is crucial in competitive programming for optimizing time complexity. It involves calculating and storing results in advance, allowing faster retrieval during program execution. This is very useful for solving complex problems efficiently within the time constraints of competitive programming competitions.
Following is a list of important topics relevant to Pre-Computation:
Competitive Programming Handbook || Chapter 9 | Graph Algorithms
Graphs are important in competitive programming because they model relationships between entities. Algorithms on graphs, like Dijkstra’s and Kruskal’s, solve various problems efficiently, such as finding shortest paths, minimum spanning trees and a lot more important graph algorithms are there. They provide powerful tools for solving a wide range of problems efficiently.
Following is a list of important topics relevant to Graph Algorithms:
Competitive Programming Handbook || Chapter 10 | Tree Algorithms
Trees are important in competitive programming because they provide efficient data structures for various algorithms and operations. Their hierarchical structure allows for quick and effective traversal, making them useful for tasks such as searching, sorting, and dynamic programming. Trees are fundamental in algorithmic problem-solving and enable optimized solutions for a wide range of programming challenges.
Following is a list of important topics relevant to Tree Algorithms:
Competitive Programming Handbook || Chapter 11 | Dynamic Programming
Dynamic Programming is crucial in competitive programming because it optimizes solutions by breaking down problems into smaller overlapping subproblems. It stores the results of subproblems to avoid redundant computations, leading to more efficient algorithms and faster execution times.
Following is a list of important topics relevant to Dynamic Programming:
Competitive Programming Handbook || Chapter 12 | Range Queries
Range Queries are important for competitive programming because they involve efficiently retrieving and manipulating elements within a specified range in a data structure. This is crucial for optimizing algorithms and achieving better time complexity, which is essential in competitive programming where efficiency is important.
Following is a list of important topics relevant to Range Queries:
Competitive Programming Handbook || Chapter 13 | String Algorithms
String algorithms are crucial in competitive programming because they enable efficient manipulation and analysis of textual data. Rapidly processing and manipulating strings is often required in problem-solving scenarios, making a solid understanding of string algorithms essential for optimizing code and achieving competitive programming success.
Following is a list of important topics relevant to String Algorithms:
Competitive Programming Handbook || Chapter 14 | Game Theory
Game Theory is important in competitive programming because it provides a strategic framework for analyzing and solving problems involving strategic interactions and decision-making. It helps programmers to model and optimize scenarios where multiple participants make choices to achieve the best outcome, enhancing problem-solving skills in competitive environments.
Following is a list of important topics relevant to Game Theory:
Competitive Programming Handbook || Chapter 15 | Advanced Topics
Advanced topics in competitive programming, such as advanced data structures, algorithms, and optimization techniques, are crucial because they enable programmers to solve complex problems efficiently within strict time and memory constraints, giving them a competitive edge in coding competitions.
Following is a list of important topics relevant to Advanced Topics:
How to get started with Competitive Programming?
Getting started with competitive programming involves a systematic approach to build your skills and tackle coding challenges efficiently. Here’s a step-by-step guide to help you begin:
- Learn a Programming Language:
- Choose a programming language you’re comfortable with or interested in (e.g., Python, C++, Java).
- Understand the basics of syntax, data types, control structures, and functions.
- Understand Basic Data Structures and Algorithms:
- Focus on fundamental data structures (arrays, linked lists, stacks, queues) and algorithms (sorting, searching).
- Learn about time and space complexity analysis.
- Explore Advanced Data Structures and Algorithms:
- Dive into more complex data structures (trees, graphs, hash tables) and algorithms (dynamic programming, greedy algorithms).
- Understand their applications and when to use them.
- Practice Regularly:
- Solve problems on online coding platforms such as GeeksforGeeks, Codeforces, HackerRank, or LeetCode.
- Start with easy problems and gradually move to medium and hard difficulty levels.
- Can also practice CSES Problem Set to help for learning algorithmic programming.
- Participate in Contests:
- Join online coding contests on platforms like Codeforces, AtCoder, or HackerRank.
- Set aside specific times for practice contests to simulate real competition conditions.
- Analyze and Optimize Your Solutions:
- After solving a problem, analyze your solution for efficiency and correctness.
- Explore different approaches and optimize your code.
- Read Editorials and Discuss Solutions:
- Review editorial solutions and other participants’ code after contests.
- Engage in online forums or communities to discuss different problem-solving approaches.
- Study Advanced Topics:
- Explore advanced topics like graph theory, number theory, and combinatorics.
- Understand specialized algorithms and techniques used in competitive programming.
- Learn Time Management:
- Practice solving problems within time limits.
- Develop a sense of when to switch approaches or move on to the next problem during a contest.
- Stay Consistent and Persevere:
- Regular, consistent practice is key to improvement.
- Embrace challenges and setbacks as opportunities to learn and grow.
- Read Books and Online Resources:
- Supplement your learning with books like “Competitive Programming” by Steven Halim and Felix Halim.
- Utilize online tutorials and courses available on platforms like GeeksforGeeks and Coursera.
- Participate in Coding Communities:
- Join coding communities on platforms like Discord or online forums.
- Engage with fellow programmers, ask questions, and share your knowledge.
Remember, competitive programming is a journey that requires patience and persistence. Stay curious, keep practicing, and continuously seek to improve your problem-solving skills.
Tips and Tricks for Competitive Programming Success:
1. Practice Regularly: Consistent practice is the key to improvement in competitive programming. Set aside dedicated time to solve problems and participate in coding contests.
2. Understand Fundamentals: Ensure a solid grasp of fundamental concepts in data structures and algorithms. Strong basics serve as a foundation for tackling more complex problems.
3. Analyze Mistakes: Learn from your mistakes. After solving a problem or participating in a contest, analyze your approach and identify areas for improvement.
4. Explore Varieties of Problems: Challenge yourself with a diverse range of problems. Exposure to different types of challenges will broaden your problem-solving skills.
5. Time Management: Develop effective time management skills during contests. Prioritize problems based on difficulty and potential points.
1. What is competitive programming?
Competitive programming is a mental sport that involves solving well-defined algorithmic and computational problems within a specified time limit. Participants, known as competitive programmers, aim to write efficient and correct code to solve these problems and compete in online or onsite coding competitions.
2. Why should I participate in competitive programming?
Participating in competitive programming sharpens problem-solving skills, enhances algorithmic thinking, and prepares individuals for technical interviews. It also fosters healthy competition, offers opportunities for learning, and provides a platform to showcase coding skills to potential employers.
3. Do I need advanced knowledge to start competitive programming?
No, competitive programming welcomes participants of all skill levels. Beginners can start with simple problems and gradually progress to more complex challenges. The key is consistent practice and a willingness to learn from mistakes.
4. Which programming language is best for competitive programming?
Popular languages for competitive programming include C++, Python, and Java. The choice depends on personal preference, but C++ is widely favored for its speed and extensive standard template library (STL).
5. How can I improve my coding speed in competitive programming?
Improving coding speed involves regular practice, familiarizing yourself with common algorithms and data structures, and mastering keyboard shortcuts in your preferred integrated development environment (IDE). Additionally, participating in timed practice contests can enhance your speed under pressure.
6. How can I manage my time effectively during a coding competition?
Time management is crucial in competitive programming. Practice solving problems under time constraints, learn to recognize when to pivot to another problem, and avoid spending too much time on a single challenge. Develop a strategy to maximize your score within the given timeframe.
7. Are there resources to help me prepare for competitive programming?
Numerous online platforms offer practice problems and organize coding contests. Websites like Codeforces, HackerRank, and LeetCode provide a wealth of challenges suitable for all skill levels. Additionally, there are books, tutorials, and courses, such as the “Competitive Programming” book by Steven Halim and Felix Halim, to aid in your preparation.
8. How do I overcome challenges and setbacks in competitive programming?
Setbacks are a natural part of the learning process. Analyze your mistakes, read editorial solutions, and seek feedback from the community. Embrace challenges as opportunities to learn and improve. Consistency and perseverance are key to overcoming obstacles in competitive programming.
9. Can competitive programming help in securing a job in the tech industry?
Yes, many tech companies value competitive programming skills and incorporate coding challenges into their interview processes. Success in competitive programming can enhance your problem-solving abilities, which is highly sought after in the tech industry.
10. How do I find and connect with the competitive programming community?
Joining online coding communities on platforms like Discord, Reddit, or participating in coding forums associated with competitive programming websites allows you to connect with like-minded individuals. Engaging in discussions, asking questions, and sharing your experiences can foster a sense of community and provide valuable insights.
11. How important is optimization in competitive programming?
Optimization is crucial. Efficient algorithms and data structures are often the key to solving problems within time limits.
12. What should I do when I’m stuck on a problem?
Take a break if needed. Sometimes, stepping away and returning with a fresh perspective can lead to new insights. Don’t hesitate to seek help from online communities.
13. How do I prepare for coding interviews through competitive programming?
Treat coding contests as mock interviews. Practice problem-solving under time constraints, simulate interview conditions, and focus on explaining your thought process.
Competitive programming is an exciting journey that combines analytical thinking with coding skills. Remember, there’s no substitute for consistent practice and a willingness to learn. Stay curious, embrace challenges, and enjoy the process of becoming a proficient competitive programmer. Happy coding!
Share your thoughts in the comments
Please Login to comment...