Interactive Problems in Competitive Programming
Last Updated :
28 Dec, 2023
Interactive Problems are those problems in which our solution or code interacts with the judge in real time. When we develop a solution for an Interactive Problem then the input data given to our solution may not be predetermined but is built for that problem specifically. The solution performs a series of exchange of data with the judge and at the end of the conversation the judge decides whether our solution was correct or not.
Â
Guessing the Number (An Interactive Problem)
In this problem the user has to guess the number during a communication with the judge. The user is provided with the upper and lower bound and he/she can ask the judge whether a number is the number to be guessed. The judge replies with -1 if the number is smaller than the number to be guessed or 1 if number is greater than the number to be guessed or 0 if it is equal to the number to be guessed.
Â
Approach 1 : Linear GuessingÂ
Â
The user can query the judge for all the numbers between lower limit and upper limit to find the solution.Â
Â
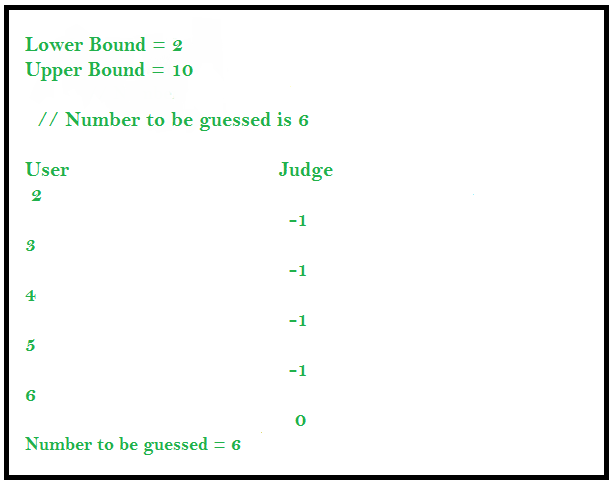
Â
C++
#include <bits/stdc++.h>
using namespace std;
int main()
{
int lower_bound = 2;
int upper_bound = 10;
for ( int i = lower_bound; i <= upper_bound; i++) {
cout << i << endl;
int response;
cin >> response;
if (response == 0) {
cout << "Number guessed is :" << i;
break ;
}
}
return 0;
}
|
Java
import java.util.*;
class GFG {
public static void main(String[] args)
{
Scanner sc1 = new Scanner(System.in);
int lower_bound = 2 ;
int upper_bound = 10 ;
for ( int i = lower_bound; i <= upper_bound; i++) {
System.out.println(i);
int response = sc1.nextInt();
if (response == 0 ) {
System.out.println( "Number guessed is :" + i);
break ;
}
}
}
}
|
Python3
if __name__ = = '__main__' :
lower_bound = 2 ;
upper_bound = 10 ;
for i in range (lower_bound, upper_bound + 1 ):
print (i)
response = int ( input ())
if (response = = 0 ):
print ( "Number guessed is :" , i, end = '')
break ;
|
C#
using System;
class GFG
{
public static void Main( string [] args)
{
int lower_bound = 2;
int upper_bound = 10;
for ( int i = lower_bound; i <= upper_bound; i++)
{
Console.WriteLine(i);
int response = int .Parse(Console.ReadLine());
if (response == 0) {
Console.WriteLine( "Number guessed is :" + i);
break ;
}
}
}
}
|
Javascript
let lowerBound = 2;
let upperBound = 10;
for (let i = lowerBound; i <= upperBound; i++) {
console.log(i);
let response = prompt( "Enter 0 if the number is guessed correctly:" );
response = parseInt(response);
if (response === 0) {
console.log( "Number guessed is: " + i);
break ;
}
}
|
Output
2
Number guessed is :2
Time Complexity: O(n)
Â
Approach 2 : Applying Binary SearchÂ
Â
We can also apply binary search interactively to find the solution. This solution is efficient as compared to the previous approach.
Â
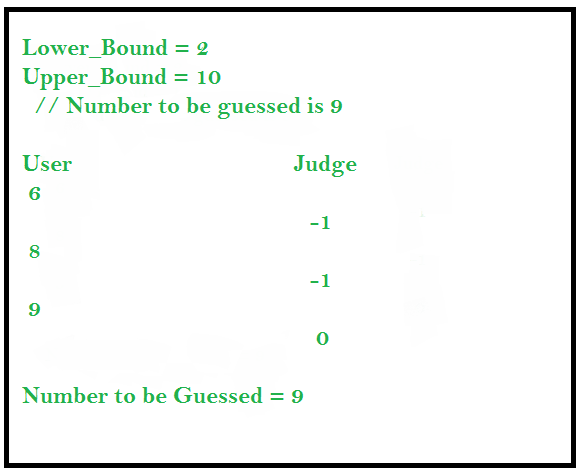
Â
C++
#include <bits/stdc++.h>
using namespace std;
int main( int argc, char ** argv)
{
int lower_bound = 2;
int upper_bound = 10;
while (lower_bound <= upper_bound)
{
int mid = (lower_bound + upper_bound) / 2;
cout << mid << endl;
int response;
cin >> response;
if (response == -1)
lower_bound = mid + 1;
else if (response == 1)
upper_bound = mid - 1;
else if (response == 0){
cout << "Number guessed is :" + to_string(mid) << endl;
break ;
}
}
return 0;
}
|
Java
import java.util.*;
class GFG {
public static void main(String[] args)
{
Scanner sc1 = new Scanner(System.in);
int lower_bound = 2 ;
int upper_bound = 10 ;
while (lower_bound <= upper_bound) {
int mid = (lower_bound + upper_bound) / 2 ;
System.out.println(mid);
int response = sc1.nextInt();
if (response == - 1 ) {
lower_bound = mid + 1 ;
}
else if (response == 1 ) {
upper_bound = mid - 1 ;
}
else if (response == 0 ) {
System.out.println( "Number guessed is :" + mid);
break ;
}
}
}
}
|
Python3
lower_bound = 2
upper_bound = 10
while (lower_bound < = upper_bound) :
mid = (lower_bound + upper_bound) / / 2
print (mid)
response = int ( input ())
if (response = = - 1 ) :
lower_bound = mid + 1
elif (response = = 1 ) :
upper_bound = mid - 1
elif (response = = 0 ) :
print ( "Number guessed is :" , mid)
break
|
C#
using System;
class GFG {
static void Main() {
int lower_bound = 2;
int upper_bound = 10;
while (lower_bound <= upper_bound) {
int mid = (lower_bound + upper_bound) / 2;
Console.WriteLine(mid);
int response = Convert.ToInt32(Console.ReadLine());
if (response == -1) {
lower_bound = mid + 1;
}
else if (response == 1) {
upper_bound = mid - 1;
}
else if (response == 0) {
Console.WriteLine( "Number guessed is :" + mid);
break ;
}
}
}
}
|
Javascript
const readline = require( 'readline' );
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
function binarySearch() {
let lowerBound = 2;
let upperBound = 10;
while (lowerBound <= upperBound) {
let mid = Math.floor((lowerBound + upperBound) / 2);
console.log(mid);
rl.question( 'Enter -1 if the number is lower, 1 if higher, or 0 if guessed correctly: ' , (response) => {
response = parseInt(response);
if (response === -1) {
lowerBound = mid + 1;
} else if (response === 1) {
upperBound = mid - 1;
} else if (response === 0) {
console.log( "Number guessed is: " + mid);
rl.close();
return ;
}
binarySearch();
});
return ;
}
}
binarySearch();
|
Output
6
Number guessed is :6
Time Complexity: O(logn)Â
Algorithm Paradigm: Divide and Conquer
Â
Share your thoughts in the comments
Please Login to comment...