How to get started with Competitive Programming in JavaScript
Last Updated :
24 Aug, 2023
Competitive programming is a popular activity among programmers looking to enhance their problem-solving skills and algorithmic thinking. JavaScript is a wonderful language for competitive programming because of how simple it is to use, how powerful its libraries are, and how quickly it can prototype ideas.
JavaScript is a great option for programming in a competitive environment because:
- First off, its easy-to-understand and concise grammar enables quicker development and simpler debugging.
- The huge standard library of JavaScript offers a wide range of modules and functions that can be used to effectively address programming difficulties.
- JavaScript also offers dynamic typing, intelligent memory management, and high-level data structures, which speed up development and simplify coding.
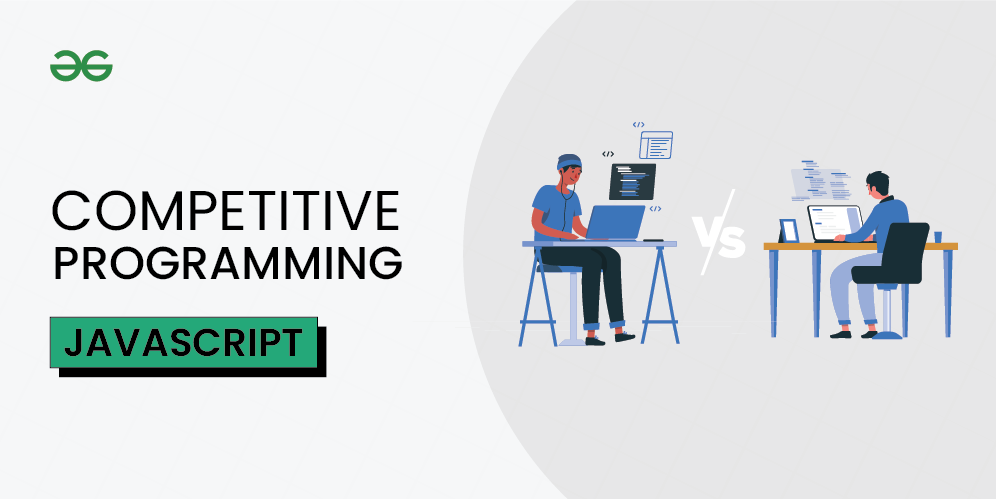
Table of Contents:
1. How to start Competitive Programming using JavaScript:
- Learn the Javascript language
- JavaScript variables and data types:
- Increase difficulty level
- Solving an easy-level problem:
- Learn Data Structures and Algorithms
- Implementing a simple linked list:
- Optimize code step by step
- Brute-force approach to find the maximum element in an array:
- Take part in contests
- Solving a simple problem on GeeksForGeeks:
- Look for Better solutions
- Comparing two solutions to find the maximum element in an array:
- Practice Time Management
2. Tips for improving in Competitive Programming: 3. Pros of Using JavaScript for CP: 4. Cons of Using JavaScript for CP: 5. Conclusion:
|
How to start Competitive Programming using JavaScript:
1. Learn the Javascript language
We should learn the basics of the JavaScript language which contain syntax, datatypes, string, etc;
JavaScript variables and data types:
Javascript
let name = "John" ;
const age = 30;
var isMarried = false ;
let fruits = [ "apple" , "banana" , "orange" ];
let person = { name: "Alice" , age: 25 };
console.log(fruits,person)
|
Output
[ 'apple', 'banana', 'orange' ] { name: 'Alice', age: 25 }
2. Increase difficulty level
After learning the basics of JavaScript, start with solving easy-level problems and then increase your difficulty by solving medium and hard problems. You can also practice on the GeeksForGeeks portal.
Solving an easy-level problem:
Javascript
function findSum(a, b) {
return a + b;
}
console.log(findSum(3,4))
|
3. Learn Data Structures and Algorithms
Learn data structures and algorithms like arrays, linked lists, stacks, queues, trees, graphs, sorting, searching, and dynamic programming.
Implementing a simple linked list:
Javascript
class Node {
constructor(data) {
this .data = data;
this .next = null ;
}
}
class LinkedList {
constructor() {
this .head = null ;
}
append(data) {
const newNode = new Node(data);
if (! this .head) {
this .head = newNode;
} else {
let current = this .head;
while (current.next) {
current = current.next;
}
current.next = newNode;
}
}
printList() {
let current = this .head;
while (current) {
console.log(current.data);
current = current.next;
}
}
}
const linkedList = new LinkedList();
linkedList.append(1);
linkedList.append(2);
linkedList.append(3);
linkedList.printList();
|
4. Optimize code step by step
Optimize your code starting with a brute force approach, a better solution, and at last best solution.
Brute-force approach to find the maximum element in an array:
Javascript
function findMaxElement(arr) {
let maxElement = arr[0];
for (let num of arr) {
if (num > maxElement) {
maxElement = num;
}
}
return maxElement;
}
|
5. Take part in contests
Along with solving problems, you should start participating in competitions like GeeksForGeeks, Google Code Jam, Hackerrank, Codechef, and Codeforces.
Solving a simple problem on GeeksForGeeks:
Javascript
function findSum(a, b) {
return a + b;
}
|
6. Look for Better solutions
Search for more effective solutions to these problems. By reading and understanding the methods used by skilled programmers.
Comparing two solutions to find the maximum element in an array:
Javascript
function findMaxElement1(arr) {
return Math.max(...arr);
}
function findMaxElement2(arr) {
let maxElement = arr[0];
for (let num of arr) {
if (num > maxElement) {
maxElement = num;
}
}
return maxElement;
}
|
7. Practice Time Management
Set time constraints for each challenge and concentrate on enhancing your accuracy and speed.
Tips for improving in Competitive Programming:
- Practice regularly and consistently.
- Focus on problem-solving, not just reading.
- Use built-in functions and libraries to save time.
- Write clean, readable, and efficient code.
- Give contests regularly.
- Upsolve after the contest.
Pros of Using JavaScript for CP:
- JavaScript’s clear syntax and simple-to-follow code make it the perfect language for competitive programming, allowing for speedy implementation and troubleshooting.
- JavaScript has a sizable standard library that offers ready-to-use modules and functions and JavaScript is one of the most popular programming languages, with a vast community of developers.
- JavaScript runs in web browsers, allowing to test and debug the code directly in the browser. This eliminates the need for compiling and running code separately.
- JavaScript’s strength lies in its ability to interact with web pages and handle web-related tasks. This can be useful in CP challenges.
Cons of Using JavaScript for CP:
- JavaScript is an interpreted language, which can lead to slower execution compared to compiled languages like C++ or Java.
- In competitive programming platforms, JavaScript might not be the most popular or preferred language.
- JavaScript manages memory automatically using garbage collection, which can introduce overhead and make it challenging to optimize memory usage.
Conclusion:
By learning JavaScript and practicing algorithmic problem-solving techniques, programmers can know the power of JavaScript for competitive programming as well, and enhance their coding skills.
Share your thoughts in the comments
Please Login to comment...