How to begin with Competitive Programming?
Last Updated :
10 Mar, 2024
Â
At the very beginning to competitive programming, barely anyone knows the coding style to be followed. Below is an example to help you understand how problems are crafted in competitive programming.
Â
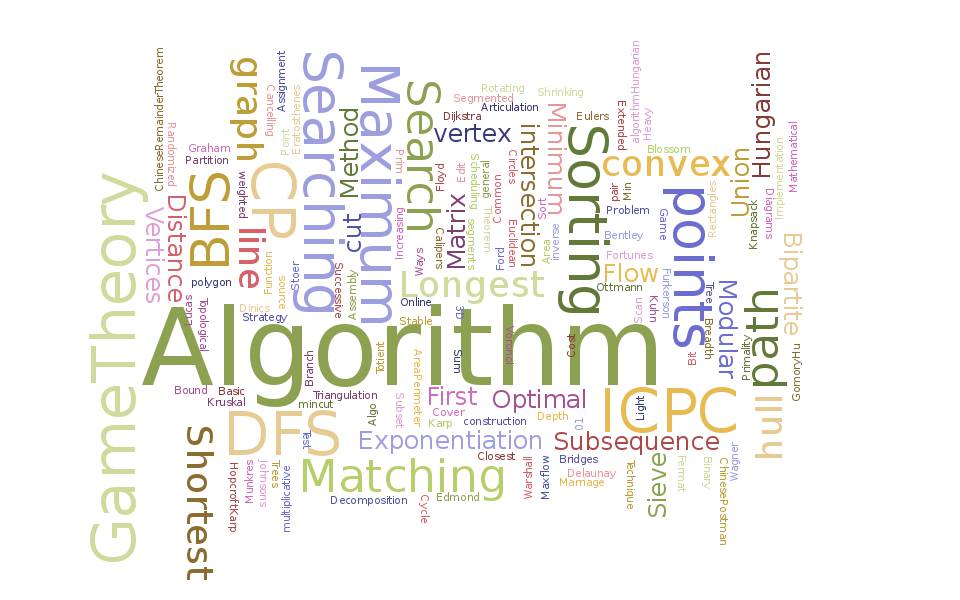
Let us consider below problem statement as an example.Â
Problem Statement:Â
Â
Linear Search: Given an integer array and an element x, find if element is present in array or not. If element is present, then print index of its first occurrence. Else print -1.
Input:Â
First line contains an integer, the number of test cases ‘T’. Each test case should be an integer. Size of the array ‘n’ in the second line. In the third line, input the integer elements of the array in a single line separated by space. Element X should be input in the fourth line, i.e., after entering the elements of array. Repeat the above steps second line onwards for multiple test cases.
Output:Â
Print the output in a separate line returning the index of the element X. If the element is not present, then print -1.
Constraints:Â
1 <= T <= 100Â
1 <= n <= 100Â
1 <= arr[i] <= 100
Example Input and Output for Your Program:Â
Â
Input:
2
4
1 2 3 4
3
5
10 90 20 30 40
40
Output:
2
4
Explanation:Â
Â
There are 2 test cases (Note 2 at the beginning of input)
Test Case 1: Input: arr[] = {1, 2, 3, 4},
Element to be searched = 3.
Output: 2
Explanation: 3 is present at index 2.
Test Case 2: Input: arr[] = {10, 90, 20, 30, 40},
Element to be searched = 40.
Output: 4
Explanation: 40 is present at index 4.
Â
C++
#include<iostream>
using namespace std;
int search( int arr[], int n, int x)
{
for ( int i = 0; i < n; i++)
{
if (arr[i] == x)
return i;
}
return -1;
}
int main()
{
int arr[100], x, t, n;
cout << "Enter the number of test cases: " ;
cin >> t;
while (t--)
{
cout << "Enter the size of array for this test case: " ;
cin >> n;
cout << "Enter " << n << " elements of array separated by space: " ;
for ( int i = 0; i < n; i++)
cin >> arr[i];
cout << "Enter the element to be searched: " ;
cin >> x;
cout << search(arr, n, x) << "\n" ;
}
return 0;
}
|
C
#include<stdio.h>
int search( int arr[], int n, int x)
{
int i;
for (i = 0; i < n; i++)
{
if (arr[i] == x)
return i;
}
return -1;
}
int main()
{
int arr[100], x, t, n, i;
scanf ( "%d" , &t);
while (t--)
{
scanf ( "%d" , &n);
for (i=0; i<n; i++)
scanf ( "%d" ,&arr[i]);
scanf ( "%d" , &x);
printf ( "%d\n" , search(arr, n, x));
}
return 0;
}
|
Java
import java.util.*;
import java.lang.*;
import java.io.*;
class LinearSearch
{
static int search( int arr[], int n, int x)
{
for ( int i = 0 ; i < n; i++)
{
if (arr[i] == x)
return i;
}
return - 1 ;
}
public static void main (String[] args) throws IOException
{
int [] arr = new int [ 100 ];
BufferedReader br = new BufferedReader( new InputStreamReader(System.in));
int t = Integer.parseInt(br.readLine());
StringBuffer sb = new StringBuffer();
while (t > 0 )
{
int n = Integer.parseInt(br.readLine());
String line = br.readLine();
String[] strs = line.trim().split( "\\s+" );
for ( int i = 0 ; i < n; i++)
arr[i] = Integer.parseInt(strs[i]);
int x = Integer.parseInt(br.readLine());
sb.append(search(arr, n, x)+ "\n" );
t--;
}
System.out.print(sb);
}
}
|
C#
using System;
class Program
{
static int Search( int [] arr, int n, int x)
{
for ( int i = 0; i < n; i++)
{
if (arr[i] == x)
return i;
}
return -1;
}
static void Main( string [] args)
{
int [] arr = new int [100];
int x, t, n;
Console.Write( "Enter the number of test cases: " );
if (! int .TryParse(Console.ReadLine(), out t))
{
Console.WriteLine( "Invalid input. Please enter a valid integer." );
return ;
}
while (t-- > 0)
{
Console.Write( "Enter the size of array for this test case: " );
if (! int .TryParse(Console.ReadLine(), out n))
{
Console.WriteLine( "Invalid input. Please enter a valid integer." );
continue ;
}
Console.Write($ "Enter {n} elements of array separated by space: " );
string [] input = Console.ReadLine().Split();
if (input.Length != n)
{
Console.WriteLine( "Invalid input. Please enter exactly n elements separated by space." );
continue ;
}
for ( int i = 0; i < n; i++)
{
if (! int .TryParse(input[i], out arr[i]))
{
Console.WriteLine( "Invalid input. Please enter valid integers separated by space." );
continue ;
}
}
Console.Write( "Enter the element to be searched: " );
if (! int .TryParse(Console.ReadLine(), out x))
{
Console.WriteLine( "Invalid input. Please enter a valid integer." );
continue ;
}
Console.WriteLine(Search(arr, n, x));
}
}
}
|
Javascript
function search(arr, x) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === x)
return i;
}
return -1;
}
function main() {
let t;
console.log( "Enter the number of test cases: " );
t = parseInt(prompt());
while (t--) {
let n;
console.log(`Enter the size of array for test case ${t + 1}: `);
n = parseInt(prompt());
let arr = new Array(n);
console.log(`Enter ${n} elements of array separated by space: `);
let elements = prompt().split( ' ' );
for (let i = 0; i < n; i++) {
arr[i] = parseInt(elements[i]);
}
let x;
console.log( "Enter the element to be searched: " );
x = parseInt(prompt());
console.log(`Result for test case ${t + 1}: ${search(arr, x)}`);
}
}
main();
|
Python3
def search(arr, x):
n = len (arr)
for j in range ( 0 ,n):
if (x = = arr[j]):
return j
return - 1
print ( "Enter the number of test cases: " )
t = int ( input ())
for i in range ( 0 ,t):
print (f "Enter the size of array for test case {i + 1}: " )
n = int ( input ())
print (f "Enter {n} elements of array separated by space: " )
arr = list ( map ( int , input ().split()))
print ( "Enter the element to be searched: " )
x = int ( input ())
print (search(arr, x))
|
Common mistakes by beginnersÂ
Â
- Program should not print any extra character. Writing a statement like printf(“Enter value of n”) would cause rejection on any platform.
- Input and output format specifications must be read carefully. For example, most of the problems expect a new line after every output. So if we don’t write printf(“\n”) or equivalent statement in a loop that runs for all test cases, the program would be rejected.
How to Begin Practice?Â
You can begin with above problem itself. Try submitting one of the above solutions here. It is recommended solve problems on Practice for cracking any coding interview.
Now you know how to write your first program in Competitive Programming Environment, you can start with School Practice Problems for Competitive Programming or Basic Practice Problems for Competitive Programming.
Platforms for practicing the Competitive ProgrammingÂ
Related Courses
Unleash the competitive programmer within you with our Competitive Programming – Live Course. In this course, you’ll learn important topics of DSA, improving problem-solving & coding skills, various techniques for competitive programming, and efficient implementation of mathematical algorithms. As soon as you start, better will be your programming skills. Enrol Now! We’ll see you inside the course!
Share your thoughts in the comments
Please Login to comment...