Why Java doesn’t support Multiple Inheritance?
Last Updated :
20 Mar, 2024
Multiple Inheritance is a feature provided by OOPS, it helps us to create a class that can inherit the properties from more than one parent. Some of the programming languages like C++ can support multiple inheritance but Java can’t support multiple inheritance. This design choice is rooted in various reasons including complexity management, ambiguity resolution, and code management concerns.
Why Java doesn’t Support Multiple Inheritance?
The major reason behind Java’s lack of support for multiple inheritance lies in its design philosophy of simplicity and clarity over complexity. By disallowing Multiple Inheritance, Java aims to prevent the ambiguity and complexities that can arise from having multiple parent classes.
Java class can be inherited from only one superclass using the extends keyword. The single inheritance model ensures a clear hierarchical structure where each class has a single direct superclass and it can facilitate easier code maintenance and reduce the potential for conflicts.
Basic syntax:
class Parent {
// Parent class members and methods
}
class Child extends Parent {
// Child class members and methods
}
Diamond Problem
The diamond problem is the classic issue that can arise with multiple inheritance. It occurs when the class inherits from the two classes that have a common ancestor. This situation forms the diamond-shaped inheritance hierarchy. It can lead to ambiguity in the method resolution.
Consider the scenario where class D inherits from classes B and C which both inherits from Class A. This creates the diamond-shaped inheritance hierarchy.
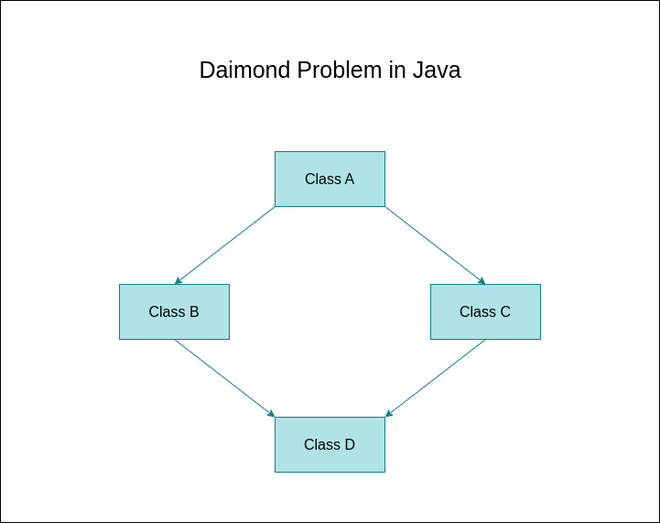
In this scenario, both the classes B and C override the check() method inherited from the class A. Now when the class D inherits from the B and C then the conflict arises because class D doesn’t know which check() method to use.
Diamond problem with example methods code:
Java
class A {
void check() {
System.out.println("Method foo() in class A");
}
}
class B extends A {
void check() {
System.out.println("Method foo() in class B");
}
}
class C extends A {
void check() {
System.out.println("Method foo() in class C");
}
}
class D extends B {
// Inherits foo() from class B
}
/*
class E extends B,C { // Error: Multiple inheritance not allowed in Java
// Some methods and members
}*/
public class Main {
public static void main(String[] args) {
D d = new D();
d.check();
// Output: Method check() in class B
// Uncommenting the below code will result in a compilation error
/*
E e = new E();
e.check(); // Compilation Error: Reference to 'check()' is ambiguous
*/
}
}
OutputMethod foo() in class B
Explanation of the above Program:
In the above example, class D can inherits from the class B and when the calling check() on the instance of the class D then it calls the check() method defined in class B. However, when defining the class E to inherit from the both B and C then it results in compilation error due to the ambiguity of which check() method to use.
This ambiguity can highlights the diamond problem which arises from potiential conflicts when the inheriting from the multiple classes with shared anecestors. So Java doesn’t allow the multiple inheritance helps to prevent such issues and ensures more robust and predictable class hierarchy.
How to Avoid Multiple Inheritance in Java?
java can provides the alternative approaches to achieve the code reuse and extensibility without resorting to the multiple inheritance.
1. Interfaces:
In Java, We can use Interfaces to define the contract for the set of behaviors without implementing them. Classes can implement the multiple interfaces and it can allowing them to inherit the method signatures from the multiple sources.
Below is the Example to Avoid Multiple Inheritance in Java with Interface:
Java
// Java Program to Implement
// MultiLevel Inheritance
// Interface 1
interface InterfaceA {
void methodA();
}
// Interface 2
interface InterfaceB {
void methodB();
}
// Class
class MyClass implements InterfaceA, InterfaceB {
public void methodA() {
// Implementation of methodA
System.out.println("A");
}
public void methodB() {
// Implementation of methodB
System.out.println("B");
}
}
class Main{
public static void main(String args[]){
// Inheriting Properties from InterfaceA
InterfaceA x=new MyClass();
// Inheriting Properties from InterfaceB
InterfaceB y=new MyClass();
x.methodA();
y.methodB();
}
}
2. Composition:
We can utilize the Composition where the objects are composed of the other objects to achieve the code reuse without the complexities of the inheritance. This can promotes the better encapsulation and flexibility of the code.
Below is the Example to Avoid Multiple Inheritance in Java with Composition:
Java
// Java Program to implement
// Java MultInheritance using Composition
import java.io.*;
// Class Engine(Parent)
class Engine {
void start() {
// Implementation of start method
System.out.println("Engine starting.....");
}
}
// Class Car(Child 1)
class Car {
// Parent Class Object
Engine engine = new Engine();
void startCar() {
// Accessing Methods of Parent Class
System.out.print("Car ... ");
engine.start();
}
}
// Class Bike(Child 3)
class Bike{
// Parent Class Object
Engine engine = new Engine();
void startBike() {
// Accessing Methods of Parent Class
System.out.print("Bike ... ");
engine.start();
}
}
// Driver Class
class GFG {
// Main Method
public static void main (String[] args) {
// Creating Objects
Car x=new Car();
Bike y=new Bike();
// Accessing Methods in Class
x.startCar();
y.startBike();
}
}
OutputCar ... Engine starting.....
Bike ... Engine starting.....
By utilizing the interfaces and composition, Java developers can circumvent the complexities of the multiple inheritance while still we can achieving the code reuse, maintainability and flexibility in the projects.
Conclusion
Java doesn’t support multiple inheritance aligns with its core prinicples of the simplicity, reliability and maintainability empowering the developer can build the concise class hierarchy. Java can provides the alternative mechanisms such as the interfaces and composition to achieve code reuse and extensiblity while avoiding the multiple inheritance.
Share your thoughts in the comments
Please Login to comment...