Types of Exception in Java with Examples
Last Updated :
11 Sep, 2023
Java defines several types of exceptions that relate to its various class libraries. Java also allows users to define their own exceptions.
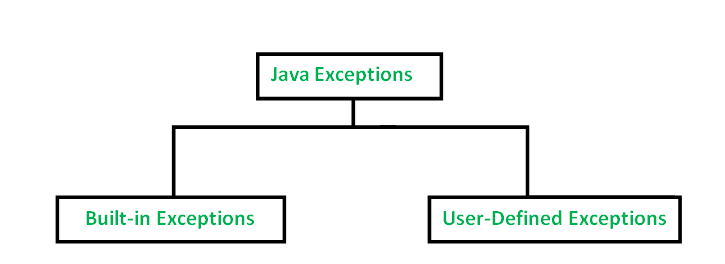
Built-in exceptions are the exceptions that are available in Java libraries. These exceptions are suitable to explain certain error situations. Below is the list of important built-in exceptions in Java.
- ArithmeticException: It is thrown when an exceptional condition has occurred in an arithmetic operation.
- ArrayIndexOutOfBoundsException: It is thrown to indicate that an array has been accessed with an illegal index. The index is either negative or greater than or equal to the size of the array.
- ClassNotFoundException: This Exception is raised when we try to access a class whose definition is not found
- FileNotFoundException: This Exception is raised when a file is not accessible or does not open.
- IOException: It is thrown when an input-output operation failed or interrupted
- InterruptedException: It is thrown when a thread is waiting, sleeping, or doing some processing, and it is interrupted.
- NoSuchFieldException: It is thrown when a class does not contain the field (or variable) specified
- NoSuchMethodException: It is thrown when accessing a method that is not found.
- NullPointerException: This exception is raised when referring to the members of a null object. Null represents nothing
- NumberFormatException: This exception is raised when a method could not convert a string into a numeric format.
- RuntimeException: This represents an exception that occurs during runtime.
- StringIndexOutOfBoundsException: It is thrown by String class methods to indicate that an index is either negative or greater than the size of the string
- IllegalArgumentException : This exception will throw the error or error statement when the method receives an argument which is not accurately fit to the given relation or condition. It comes under the unchecked exception.
- IllegalStateException : This exception will throw an error or error message when the method is not accessed for the particular operation in the application. It comes under the unchecked exception.
Examples of Built-in Exception
A. Arithmetic exception
Java
class ArithmeticException_Demo
{
public static void main(String args[])
{
try {
int a = 30 , b = 0 ;
int c = a/b;
System.out.println ( "Result = " + c);
}
catch (ArithmeticException e) {
System.out.println ( "Can't divide a number by 0" );
}
}
}
|
Output
Can't divide a number by 0
B. NullPointer Exception
Java
class NullPointer_Demo
{
public static void main(String args[])
{
try {
String a = null ;
System.out.println(a.charAt( 0 ));
} catch (NullPointerException e) {
System.out.println( "NullPointerException.." );
}
}
}
|
Output
NullPointerException..
C. StringIndexOutOfBound Exception
Java
class StringIndexOutOfBound_Demo
{
public static void main(String args[])
{
try {
String a = "This is like chipping " ;
char c = a.charAt( 24 );
System.out.println(c);
}
catch (StringIndexOutOfBoundsException e) {
System.out.println( "StringIndexOutOfBoundsException" );
}
}
}
|
Output
StringIndexOutOfBoundsException
D. FileNotFound Exception
Java
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
class File_notFound_Demo {
public static void main(String args[]) {
try {
FileReader fr = new FileReader(file);
} catch (FileNotFoundException e) {
System.out.println( "File does not exist" );
}
}
}
|
Output:
File does not exist
E. NumberFormat Exception
Java
class NumberFormat_Demo
{
public static void main(String args[])
{
try {
int num = Integer.parseInt ( "akki" ) ;
System.out.println(num);
} catch (NumberFormatException e) {
System.out.println( "Number format exception" );
}
}
}
|
Output
Number format exception
F. ArrayIndexOutOfBounds Exception
Java
class ArrayIndexOutOfBound_Demo
{
public static void main(String args[])
{
try {
int a[] = new int [ 5 ];
a[ 6 ] = 9 ;
}
catch (ArrayIndexOutOfBoundsException e){
System.out.println ( "Array Index is Out Of Bounds" );
}
}
}
|
Output
Array Index is Out Of Bounds
G. IO Exception
Java
class IOException_Demo {
public static void main(String[] args)
{
Scanner scan = new Scanner( "Hello Geek!" );
System.out.println( "" + scan.nextLine());
System.out.println( "Exception Output: "
+ scan.ioException());
scan.close();
}
}
|
Output:
Hello Geek!
Exception Output: null
H. NoSuchMethod Exception
Java
public class NoSuchElementException_Demo {
public static void main(String[] args)
{
Set exampleleSet = new HashSet();
Hashtable exampleTable = new Hashtable();
exampleleSet.iterator().next();
exampleTable.elements().nextElement();
}
}
|
I. IllegalArgumentException: This program, checks whether the person is eligible for voting or not. If the age is greater than or equal to 18 then it will not throw any error. If the age is less than 18 then it will throw an error with the error statement.
Also, we can specify “throw new IllegalArgumentException()” without the error message. We can also specify Integer.toString(variable_name) inside the IllegalArgumentException() and It will print the argument name which is not satisfied the given condition.
Java
import java.io.*;
class GFG {
public static void print( int a)
{
if (a>= 18 ){
System.out.println( "Eligible for Voting" );
}
else {
throw new IllegalArgumentException( "Not Eligible for Voting" );
}
}
public static void main(String[] args) {
GFG.print( 14 );
}
}
|
Output :
Exception in thread "main" java.lang.IllegalArgumentException: Not Eligible for Voting
at GFG.print(File.java:13)
at GFG.main(File.java:19)
J. IllegalStateException: This program, displays the addition of numbers only for Positive integers. If both the numbers are positive then only it will call the print method to print the result otherwise it will throw the IllegalStateException with an error statement. Here, the method is not accessible for non-positive integers.
Also, we can specify the “throw new IllegalStateException()” without the error statement.
Java
import java.io.*;
class GFG {
public static void print( int a, int b)
{
System.out.println( "Addition of Positive Integers :" +(a+b));
}
public static void main(String[] args) {
int n1= 7 ;
int n2=- 3 ;
if (n1>= 0 && n2>= 0 )
{
GFG.print(n1,n2);
}
else
{
throw new IllegalStateException( "Either one or two numbers are not Positive Integer" );
}
}
}
|
Output :
Exception in thread "main" java.lang.IllegalStateException: Either one or two numbers are not Positive Integer
at GFG.main(File.java:20)
k. ClassNotFound Exception :
Java
public class ClassNotFoundException_Demo
{
public static void main(String[] args) {
try {
Class.forName( "Class1" );
}
catch (ClassNotFoundException e){
System.out.println(e);
System.out.println( "Class Not Found..." );
}
}
}
|
Output
java.lang.ClassNotFoundException: Class1
Class Not Found...
User-Defined Exceptions
Sometimes, the built-in exceptions in Java are not able to describe a certain situation. In such cases, the user can also create exceptions which are called ‘user-defined Exceptions’.
The following steps are followed for the creation of a user-defined Exception.
- The user should create an exception class as a subclass of the Exception class. Since all the exceptions are subclasses of the Exception class, the user should also make his class a subclass of it. This is done as:
class MyException extends Exception
- We can write a default constructor in his own exception class.
MyException(){}
- We can also create a parameterized constructor with a string as a parameter.
We can use this to store exception details. We can call the superclass(Exception) constructor from this and send the string there.
MyException(String str)
{
super(str);
}
- To raise an exception of a user-defined type, we need to create an object to his exception class and throw it using the throw clause, as:
MyException me = new MyException(“Exception details”);
throw me;
- The following program illustrates how to create your own exception class MyException.
- Details of account numbers, customer names, and balance amounts are taken in the form of three arrays.
- In main() method, the details are displayed using a for-loop. At this time, a check is done if in any account the balance amount is less than the minimum balance amount to be apt in the account.
- If it is so, then MyException is raised and a message is displayed “Balance amount is less”.
Example
Java
class MyException extends Exception
{
private static int accno[] = { 1001 , 1002 , 1003 , 1004 };
private static String name[] =
{ "Nish" , "Shubh" , "Sush" , "Abhi" , "Akash" };
private static double bal[] =
{ 10000.00 , 12000.00 , 5600.0 , 999.00 , 1100.55 };
MyException() { }
MyException(String str) { super (str); }
public static void main(String[] args)
{
try {
System.out.println( "ACCNO" + "\t" + "CUSTOMER" +
"\t" + "BALANCE" );
for ( int i = 0 ; i < 5 ; i++)
{
System.out.println(accno[i] + "\t" + name[i] +
"\t" + bal[i]);
if (bal[i] < 1000 )
{
MyException me =
new MyException( "Balance is less than 1000" );
throw me;
}
}
}
catch (MyException e) {
e.printStackTrace();
}
}
}
|
Runtime Error
MyException: Balance is less than 1000
at MyException.main(fileProperty.java:36)
Output:
ACCNO CUSTOMER BALANCE
1001 Nish 10000.0
1002 Shubh 12000.0
1003 Sush 5600.0
1004 Abhi 999.0
Related Articles:
Share your thoughts in the comments
Please Login to comment...