Chakra UI Feedback Alert
Last Updated :
30 Jan, 2024
Chakra UI Feedback Alert is used to interrupt the user with a mandatory confirmation or action. The Alert component can be customized by changing its status, which controls the color of the alert. Various alerts could be of different colors.
Prerequisites:
Steps To Create React Appllication And Installing Module:
Step 1: First of all, create a react application by running the following command.
npx create-react-app myapp
Step 2: Now navigate to the myapp directory by running the following command:
cd myapp
Step 3: Installing the Chakra UI by running the following command:
npm install @chakra-ui/react @emotion/react @emotion/styled framer-motion
Setting up the Chakra UI Provider
Example: In your index.js or App.js file, wrap your application in the ChakraProvider component. This step is crucial as it provides the necessary context for Chakra UI components.
Javascript
import React from 'react' ;
import ReactDOM from 'react-dom' ;
import { ChakraProvider } from '@chakra-ui/react' ;
import App from './App' ;
ReactDOM.render(
<React.StrictMode>
<ChakraProvider>
<App />
</ChakraProvider>
</React.StrictMode>,
document.getElementById( 'root' )
);
|
Using the Alert in the Application:
To use an Alert, you need to import four main components from Chakra UI in our react application.
- Alert: The container for the alert components.
- AlertIcon: An icon indicating the type of alert.
- AlertTitle: A title for the alert, which is important for screen readers.
- AlertDescription: Additional information about the alert.
In the app.js file use the following code to implement the alert.
Javascript
import './App.css' ;
import {
Alert,
AlertIcon,
AlertTitle,
AlertDescription,
} from '@chakra-ui/react' ;
function App() {
return (
<div className= "App" >
<Alert status= "error" >
<AlertIcon />
<AlertTitle mr={2}>
Your browser is outdated!
</AlertTitle>
<AlertDescription>
Your Chakra experience may be degraded.
</AlertDescription>
</Alert>
</div>
);
}
export default App;
|
Step to Run the code:
- Using the following command to start your application.
npm start
Output:
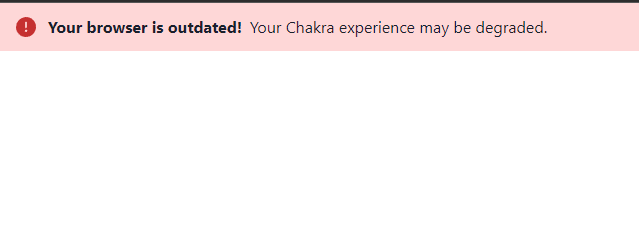
By following these steps, you can easily integrate Chakra UI’s Feedback Alert into your React application. This not only enhances the user interface but also provides a more interactive and responsive experience for users. Chakra UI’s simplicity and ease of use make it an excellent choice for developers looking to implement effective UI elements in their React applications.
Share your thoughts in the comments
Please Login to comment...