Kill a Process by name using Python
Last Updated :
30 Jun, 2021
A process is identified on the system by what is referred to as a process ID and no other process can use that number as its process ID while that first process is still running. Imagine you are a system administrator of a company and you start an application from your menu and you start using that application suddenly you notice that the application stopped working or die unexpectedly. You again try to start that application but it turns out that application never shut down completely. Since you are an administrator you type a command to Process ID and kill that process immediately. Imagine this scenario the employees of your company came to you every day complaining about the same situation since they don’t know what is PID and how to kill a process. So you came up with the idea of writing a script in Python which take input only the name of application or process and shut it down completely. You gave this script to your employees so every time this happens they don’t need to complain to you or know what is process id or how to kill the process, just enter the name, and everything will be taken care of.
Functions used:
- os.popen(): This method is used to opens a pip to and from command.
In the image below you can see that the process firefox is running
- os.kill(): This method in Python is used to send a specified signal to the process with specified process id.
Below is the implementation.
In the image below you can see that the process firefox is running.
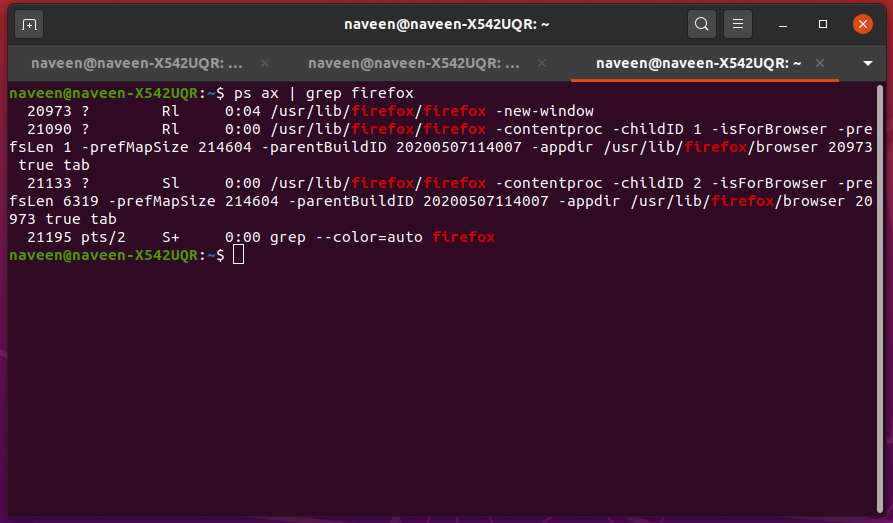
Python3
import os, signal
def process():
name = input ( "Enter process Name: " )
try :
for line in os.popen( "ps ax | grep " + name + " | grep -v grep" ):
fields = line.split()
pid = fields[ 0 ]
os.kill( int (pid), signal.SIGKILL)
print ( "Process Successfully terminated" )
except :
print ( "Error Encountered while running script" )
process()
|
Output:
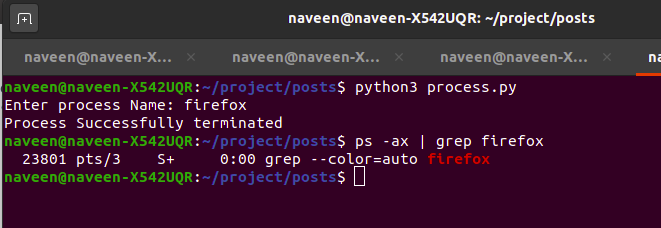
In the image above you can see that all the instance of firefox has been terminated. The one that you seeing in the image is the instance that is being called by grep command. You can now check that your firefox browser has been shut completely.
Share your thoughts in the comments
Please Login to comment...