Java Ternary Operator with Examples
Last Updated :
01 Apr, 2024
Operators constitute the basic building block of any programming language. Java provides many types of operators that can be used according to the need to perform various calculations and functions, be it logical, arithmetic, relational, etc. They are classified based on the functionality they provide. Here are a few types:Â
- Arithmetic Operators
- Unary Operators
- Assignment Operator
- Relational Operators
- Logical Operators
- Ternary Operator
- Bitwise Operators
- Shift Operators
This article explains all that one needs to know regarding Ternary Operators.Â
Ternary Operator in Java
Java ternary operator is the only conditional operator that takes three operands. It’s a one-liner replacement for the if-then-else statement and is used a lot in Java programming. We can use the ternary operator in place of if-else conditions or even switch conditions using nested ternary operators. Although it follows the same algorithm as of if-else statement, the conditional operator takes less space and helps to write the if-else statements in the shortest way possible.
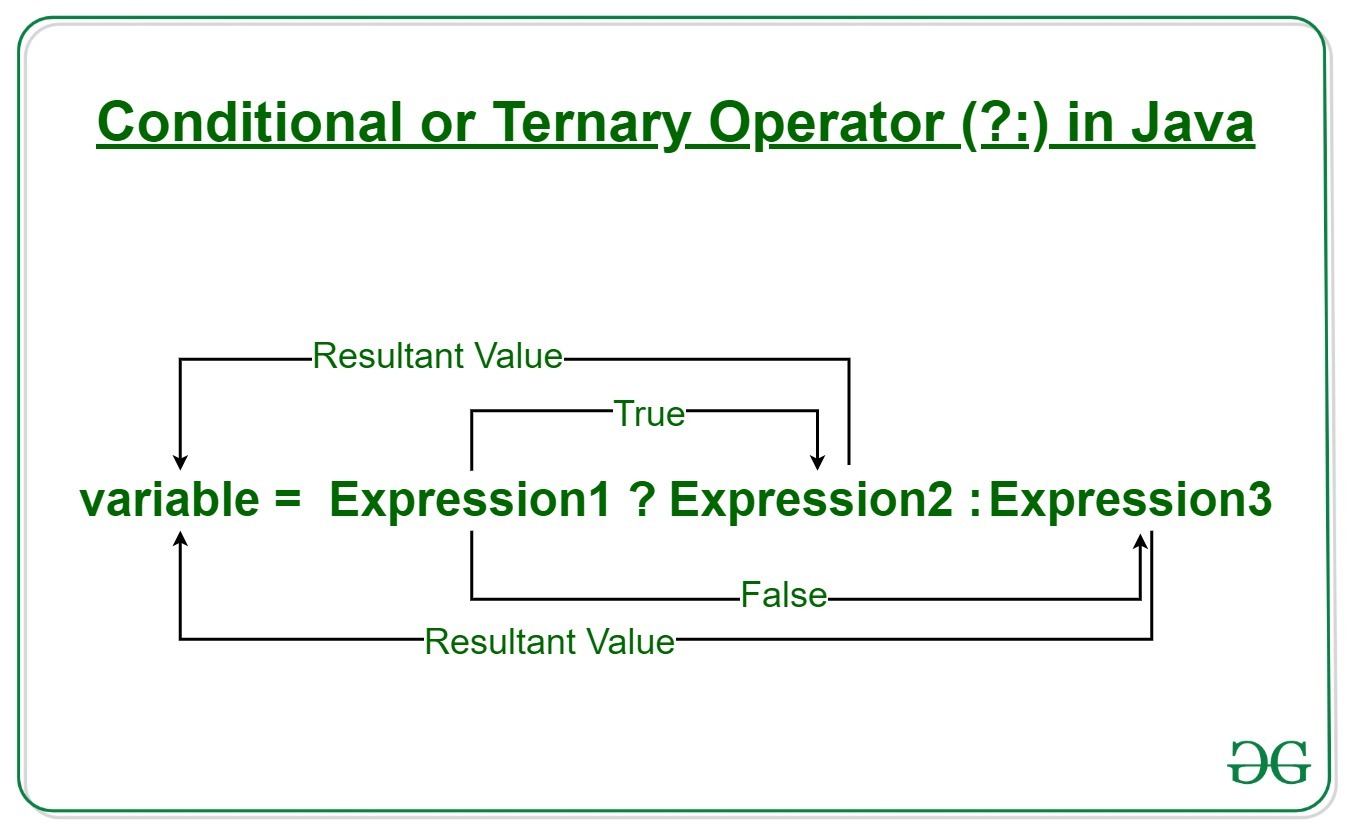
Syntax:Â
variable = Expression1 ? Expression2: Expression3
If operates similarly to that of the if-else statement as in Exression2 is executed if Expression1 is true else Expression3 is executed. Â
if(Expression1)
{
variable = Expression2;
}
else
{
variable = Expression3;
}
Example:Â Â
num1 = 10;
num2 = 20;
res=(num1>num2) ? (num1+num2):(num1-num2)
Since num1<num2,
the second operation is performed
res = num1-num2 = -10
Flowchart of Ternary Operation Â
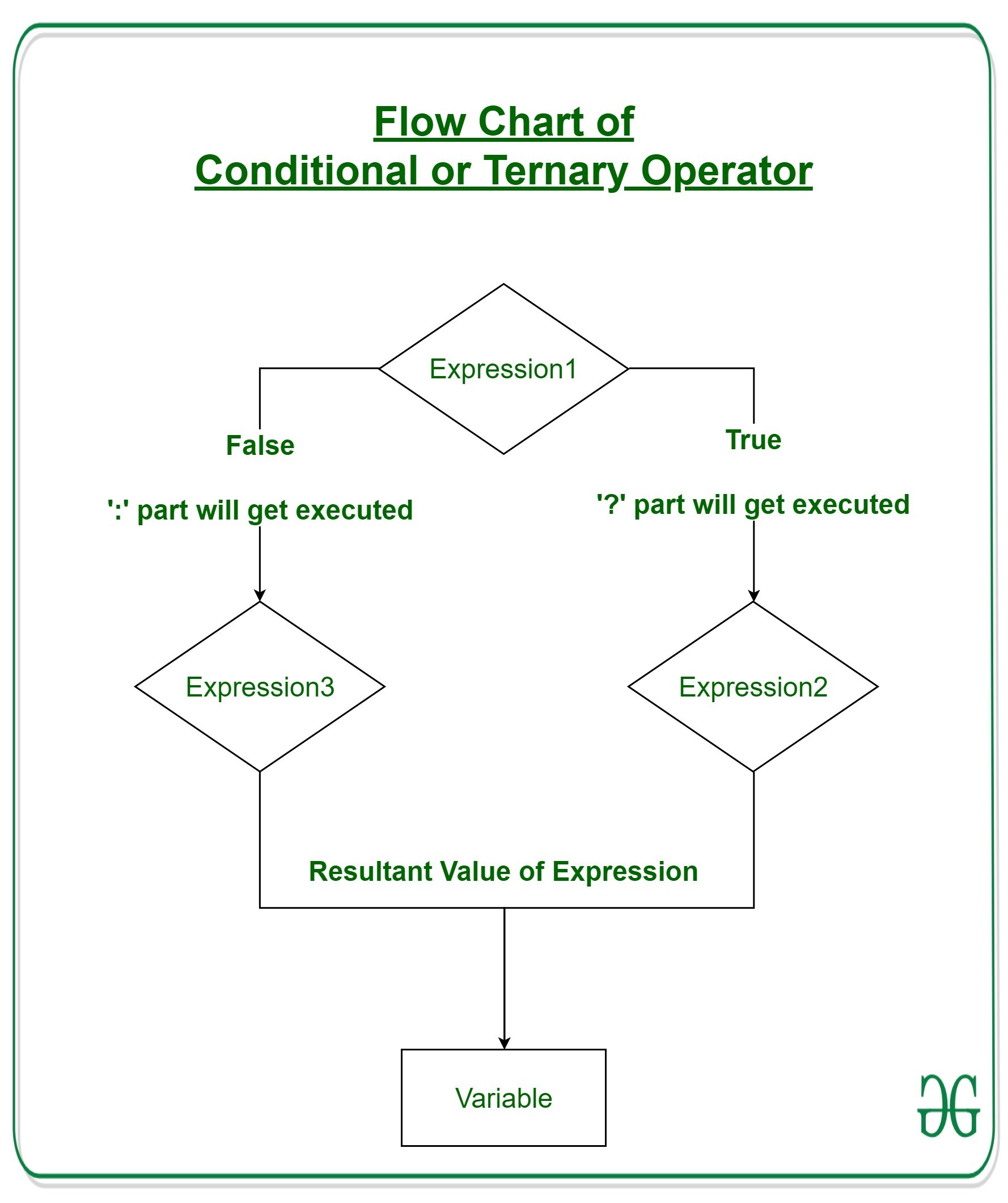
Examples of Ternary Operators in Java
Example 1:Â
Below is the implementation of the Ternary Operator:
Java
// Java program to find largest among two
// numbers using ternary operator
import java.io.*;
class Ternary {
public static void main(String[] args)
{
// variable declaration
int n1 = 5, n2 = 10, max;
System.out.println("First num: " + n1);
System.out.println("Second num: " + n2);
// Largest among n1 and n2
max = (n1 > n2) ? n1 : n2;
// Print the largest number
System.out.println("Maximum is = " + max);
}
}
OutputFirst num: 5
Second num: 10
Maximum is = 10
Complexity of the above method:
Time Complexity: O(1)
Auxiliary Space: O(1)
Example 2:Â
Below is the implementation of the above method:
Java
// Java code to illustrate ternary operator
import java.io.*;
class Ternary {
public static void main(String[] args)
{
// variable declaration
int n1 = 5, n2 = 10, res;
System.out.println("First num: " + n1);
System.out.println("Second num: " + n2);
// Performing ternary operation
res = (n1 > n2) ? (n1 + n2) : (n1 - n2);
// Print the largest number
System.out.println("Result = " + res);
}
}
OutputFirst num: 5
Second num: 10
Result = -5
Complexity of the above method:
Time Complexity: O(1)
Auxiliary Space: O(1)
Example 3:
Implementing ternary operator on Boolean values:
Java
// Java Program for Implementing
// Ternary operator on Boolean values
// Driver Class
public class TernaryOperatorExample {
// main function
public static void main(String[] args)
{
boolean condition = true;
String result = (condition) ? "True" : "False";
System.out.println(result);
}
}
Explanation of the above method:
In this program, a Boolean variable condition is declared and assigned the value true. Then, the ternary operator is used to determine the value of the result string. If the condition is true, the value of result will be “True”, otherwise it will be “False”. Finally, the value of result is printed to the console.
Advantages of Java Ternary Operator
- Compactness: The ternary operator allows you to write simple if-else statements in a much more concise way, making the code easier to read and maintain.
- Improved readability: When used correctly, the ternary operator can make the code more readable by making it easier to understand the intent behind the code.
- Increased performance: Since the ternary operator evaluates a single expression instead of executing an entire block of code, it can be faster than an equivalent if-else statement.
- Simplification of nested if-else statements: The ternary operator can simplify complex logic by providing a clean and concise way to perform conditional assignments.
- Easy to debug: If a problem occurs with the code, the ternary operator can make it easier to identify the cause of the problem because it reduces the amount of code that needs to be examined.
It’s worth noting that the ternary operator is not a replacement for all if-else statements. For complex conditions or logic, it’s usually better to use an if-else statement to avoid making the code more difficult to understand.
Share your thoughts in the comments
Please Login to comment...