How to Perform Debugging in Python PyCharm?
Last Updated :
20 Mar, 2024
Debugging is like finding and fixing mistakes in your computer code. PyCharm is a tool that helps with this, especially for Python code. It has special tools to make debugging easier. Whether you’re new to programming or have been doing it for a while, getting good at debugging in PyCharm can make you a better coder. In this article, we will see how to Perform debugging in PyCharm.
Perform Debugging in Python PyCharm
When we run the Python code it shows two modes.
Now in this article let’s focus on debugging the Python script file using PyCharm. While operating debugging there are some steps to be followed.
Step 1: Access Project Files in PyCharm
Open the project file and click the left button of the mouse. Then the following display will appear.
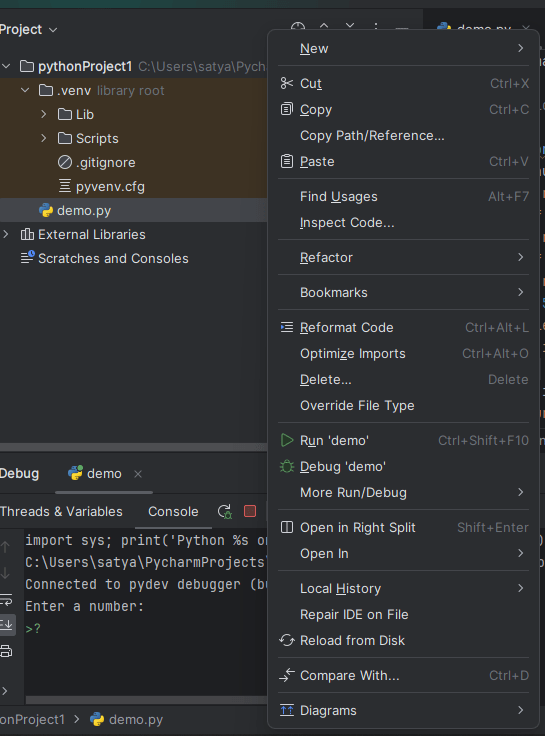
Step 2: Start Debugging in PyCharm
After clicking on a option Debug or press ‘Shift+F9’ , The Window firewall displays a pop-up message for debugging the project file for line by line compilation.
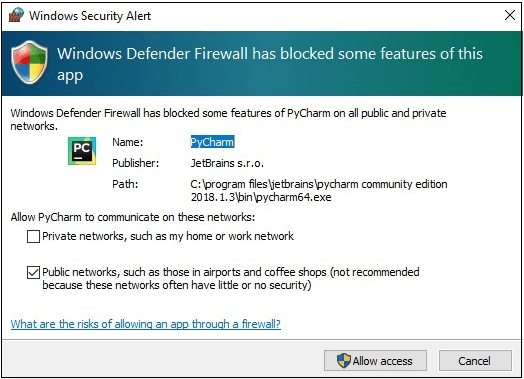
Step 3: Access Debugging Controls
After setting the permission then PyCharm starts debugging, the debugging executes till it reaches the break point, all the debugging controls will displayed on debug tool window. Lets take an example of a Python program in which we perform an operation of debugging in python.
Python3
import math
# Function to check if a number is prime
def is_prime(num):
if num <= 1:
return False
elif num <= 3:
return True
elif num % 2 == 0 or num % 3 == 0:
return False
i = 5
while i <= math.isqrt(num):
if num % i == 0 or num % (i + 2) == 0:
return False
i += 6
return True
# Take input from the user
user_input = int(input("Enter a number: "))
print("Checking if", user_input, "is prime...")
# Check if the input is prime
if is_prime(user_input):
print(user_input, "is a prime number.")
else:
print(user_input, "is not a prime number.")
-min-min.png)
Place a Breakpoints in the code by clicking on the number to suspend the program when Exception or its subclasses are thrown.

Step 4: Controls in PyCharm’s Debug Tool Window
In the debug tool window, we will find various controls to help us navigate through our code while debugging. Now let us see some of the most commonly used tools.

- Step Over (F8): Executes the current line of code and moves to the next line. If the current line contains a function call, it will execute the entire function and pause on the next line after the function call.
- Step Into (F7): Moves the debugger into the function call, allowing you to step through the function’s code line by line.
- Step Out (Shift+F8): Executes the remaining lines of the current function and returns to the function that is called.
- Resume Program (F9): Continues execution until the next breakpoint is encountered or if no break point was found it executes till the end of a program.
Step 5 : Inspect variable and Expression Evaluation in Debugging
We can inspect the values of variables in our code by hovering over them with our mouse or by using the “Variables” pane present in the debug tool window. Similarly we can also evaluate expressions and watch variables by typing them into the “Evaluate Expression” field in the debug tool window.
Step 6: Terminate Debugging
If any error was found simply edit the code in the editor window and PyCharm will automatically save the changes. After completing the debugging session terminate the session by clicking the “Stop” icon in debug tool window and or by pressing “Ctrl+F2”.
Inline Debugging
Inline Debugging a technique where we can inspect the values of variables and expressions directly in our code. This is used for quick state of code at specific points during execution.
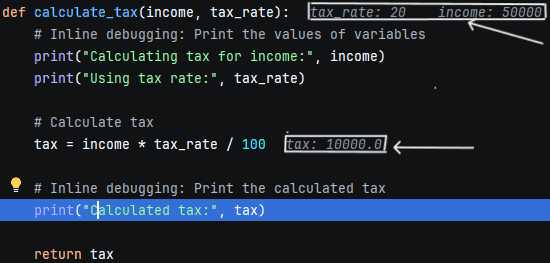
Inline Debugging
The above indicated points are the inline debugging which shows expression directly in our code. By following Process we can performing the debugging in PyCharm and by debugging tools built into PyCharm, we can easily identify and fix issues in our code.
Share your thoughts in the comments
Please Login to comment...