Chakra UI Layout, width and height
Last Updated :
25 Jan, 2024
Chakra UI is the React component library for building attractive and responsive user interfaces. This library has various pre-styled components like layouts, width, and height. Using the Layout component, we can align and structure the UI elements responsively. Width and height components are important aspects to control the overall responsive sizing of the element. In this article, we will see the practical implementation of Chakra UI Layout, width, and height in terms of examples.
Prerequisites:
Approach:
We have created a UI box and four different button elements that control the Width and Height of the UI Box. We are using the Box and Flex layout to align the elements on the screen. Using the controlling logic, the user manages the width and height according to button inputs.
Steps to Create React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app chakra
Step 2: After creating your project folder(i.e. chakra), move to it by using the following command:
cd chakra
Step 3: After creating the React application, Install the required package using the following command:
npm i @chakra-ui/react @emotion/react@^11 @emotion/styled@^11 framer-motion@^6
Project Structure:
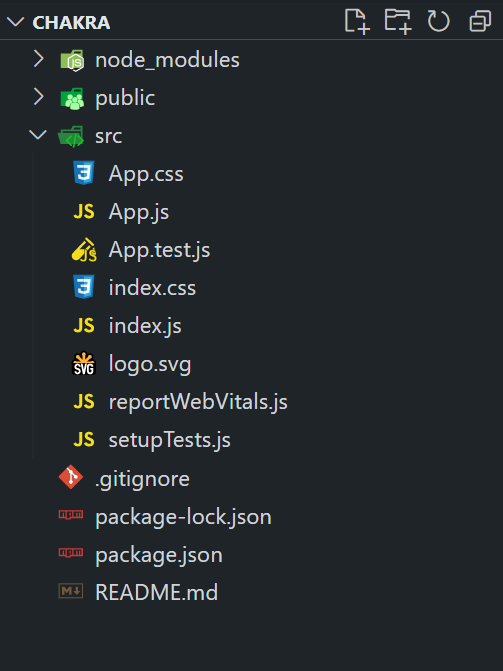
The updated dependencies are in the package.json file.
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.3",
"@emotion/styled": "^11.11.0",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"framer-motion": "^6.5.1",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: Below is the practical implementation of the Chakra UI Layout, width and height.
Javascript
import {
ChakraProvider, Box,
Button, Heading, Flex
} from "@chakra-ui/react" ;
import React,
{
useState
} from "react" ;
function App() {
const [box_wid, set_box_wid] = useState( "200px" );
const [box_height, set_box_height] = useState( "200px" );
const widthIncreaseFn =
() => {
set_box_wid(`
${parseInt(box_wid) + 10}px
`);
};
const widthDecreaseFn =
() => {
set_box_wid(`
${parseInt(box_wid) - 10}px
`);
};
const heightIncreaseFn =
() => {
set_box_height(`
${parseInt(box_height) + 10}px
`);
};
const heightDecreaseFn =
() => {
set_box_height(`
${parseInt(box_height) - 10}px
`);
};
return (
<ChakraProvider>
<Box p={4} textAlign= "center" >
<Heading as= "h1"
color= "green.500" mb={4}>
GeeksforGeeks
</Heading>
<Heading as= "h3" >
Chakra UI Layout,
width and height
</Heading>
<Flex justifyContent= "center"
alignItems= "center" flexDirection= "column" >
<Box
width={box_wid}
height={box_height}
backgroundColor= "blue.500"
color= "white"
textAlign= "center"
p={4}
borderRadius= "md"
boxShadow= "md"
mb={4}>
<p>Width: {box_wid}</p>
<p>Height: {box_height}</p>
</Box>
<Flex>
<Button onClick={widthIncreaseFn} mr={2}>
Increase Box Width
</Button>
<Button onClick={widthDecreaseFn} mr={2}>
Decrease Box Width
</Button>
<Button onClick={heightIncreaseFn} mr={2}>
Increase Box Height
</Button>
<Button onClick={heightDecreaseFn}>
Decrease Box Height
</Button>
</Flex>
</Flex>
</Box>
</ChakraProvider>
);
}
export default App;
|
Step to run the application: Run the application using the following command:
npm run start
Output: Now go to http://localhost:3000 in your browser:
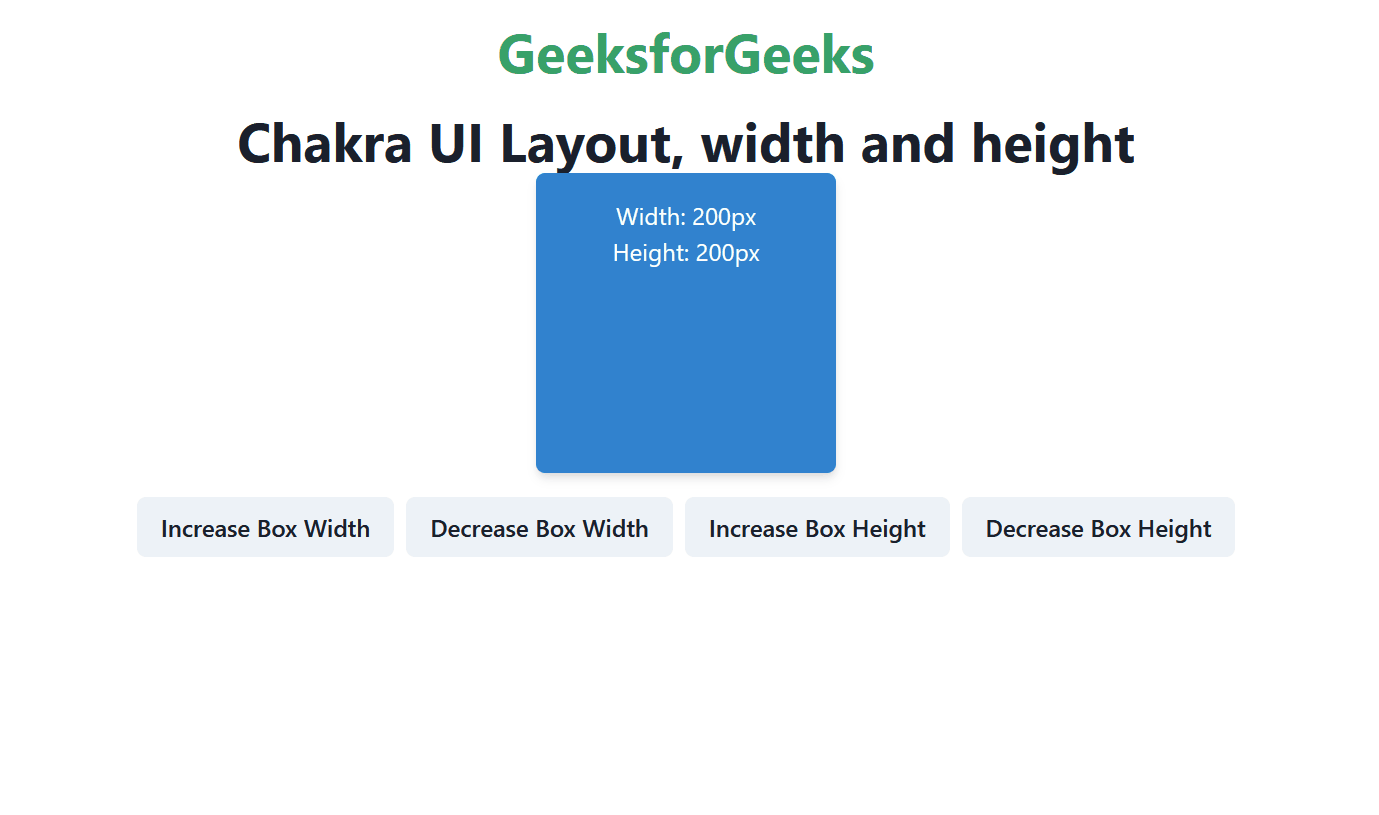
Share your thoughts in the comments
Please Login to comment...