React Component is the building blocks of the React application. It is the core concept that everyone must know while working on React. In this tutorial, we will see the top React Components questions that can be asked in the interview.
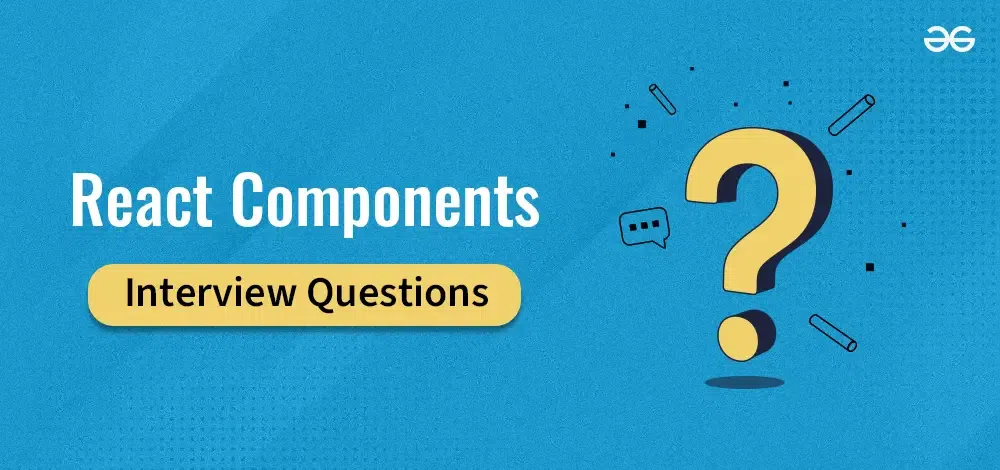
Let’s discuss some common questions that you should prepare for the interviews. These questions will be helpful in clearing the interviews, especially for the frontend development or full stack development role.
A Component is one of the core building blocks of React. In other words, we can say that every application you will develop in React will be made up of pieces called components. Components make the task of building UIs much easier. You can see a UI broken down into multiple individual pieces called components and work on them independently and merge them all in a parent component which will be your final UI.Â
The two types of React components are class components and functional components.
Class components: These are ES6 classes that extend the React.Component
class. They have a render()
method where you define what should be rendered to the DOM. Class components can manage their own state using setState()
and have access to lifecycle methods such as componentDidMount()
and componentDidUpdate()
.
Syntax:
class Democomponent extends React.Component {
render() {
return <h1>Welcome Message!</h1>;
}
}
Functional components: These are simple JavaScript functions that return JSX (a syntax extension for JavaScript used with React). They are also known as stateless functional components or just functional components.
Syntax:
function demoComponent() {
return <h1>Welcome Message!</h1>;
}
Feature |
Class Component |
Functional Component |
Syntax |
Extends React.Component class |
Simple JavaScript function |
State |
Can manage state using this.state and setState() |
Traditionally stateless, but can manage state using Hooks (useState) |
Lifecycle Methods |
Has access to lifecycle methods like componentDidMount() and componentDidUpdate() |
Does not have access to lifecycle methods (prior to Hooks) |
Boilerplate |
Typically requires more code and boilerplate |
Requires less code and is more concise |
Readability |
Can sometimes be more verbose and complex |
Generally more straightforward and readable |
4. How do you create a class component in React?
To create a class component in React, you typically define a JavaScript class that extends the React.Component
class.
1. Import React:
import React from 'react';
2. Define your class component:
class MyComponent extends React.Component {
render() {
return (
// JSX code representing the component's UI
<div>
<h1>Hello, World!</h1>
</div>
);
}
}
3. Export your component:
export default MyComponent;
5. How do you create a functional component in React?
To create a functional component in React, you simply define a JavaScript function that returns JSX .
1. Import React (not always necessary, but required if using JSX):
import React from 'react';
2. Define your functional component:
function MyFunctionalComponent() {
return (
// JSX code representing the component's UI
<div>
<h1>Hello, World!</h1>
</div>
);
}
3. Export your component:
export default MyFunctionalComponent;
The render()
method in a class component is a fundamental part of React’s component lifecycle. Its purpose is to define what should be rendered to the DOM when the component is rendered or updated.
- Returning JSX: The primary purpose of the
render()
method is to return JSX that represents the UI of the component.
- Component Re-rendering: Whenever the state or props of a component change, React will automatically call the
render()
method again to re-render the component and update the DOM with any changes.
- Virtual DOM: React uses a virtual DOM to efficiently update the actual DOM. The
render()
method generates a virtual DOM representation of the component’s UI.
To render a React component on the DOM, you typically use the ReactDOM.render()
method.
1. Import React and ReactDOM:
import React from 'react';
import ReactDOM from 'react-dom';
2. Define your React component:
function MyComponent() {
return (
// JSX code representing the component's UI
<div>
<h1>Hello, World!</h1>
</div>
);
}
3. Use ReactDOM.render()
to render your component onto the DOM:
ReactDOM.render(<MyComponent />, document.getElementById('root'));
JSX stands for JavaScript XML. It is a syntax extension for JavaScript, often used with React to describe what the UI should look like. JSX may remind you of a template language, but it comes with the full power of JavaScript.
Difference between JSX and HTML
Feature |
JSX |
HTML |
Case Sensitivity |
JSX is case-sensitive with camelCase properties. |
HTML is not case-sensitive.
|
Logic Integration |
JSX allows JavaScript expressions. |
HTML does not have logic built-in; it requires a separate script.
|
Node Structure |
JSX requires a single root node. |
HTML does not have this restriction.
|
Attribute/Property Naming |
Attributes are camelcased (e.g., onClick). |
Attributes are lowercase (e.g., click).
|
Custom Components |
JSX can have custom components (e.g., <MyComponent />). |
HTML does not support custom components natively. |
You can pass props to a React component by adding attributes to the component when you use it in JSX.
// Define a functional component
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
// Render the component with props
ReactDOM.render(<Greeting name="John" />, document.getElementById('root'));
10. How do you access props inside a functional component?
To access props inside a functional component, you simply define the function with a parameter representing the props object. Then, you can access individual props from this object.
// Define a functional component
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
11. How do you access props inside a class component?
To access props inside a class component, you can use this.props
within any method or lifecycle hook of the class.
// Define a class component
class Greeting extends React.Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
The state in React is an instance of the React Component Class that can be defined as an object of a set of observable properties that control the behavior of the component. In other words, the State of a component is an object that holds some information that may change over the lifetime of the component.
PROPS
|
STATE
|
The Data is passed from one component to another. |
The Data is passed within the component only. |
It is Immutable (cannot be modified). |
It is Mutable ( can be modified). |
Props can be used with state and functional components. |
The state can be used only with the state components/class component (Before 16.0). |
Props are read-only. |
The state is both read and write. |
In a class component in React, you initialize state in the constructor method.
Javascript
import React from 'react' ;
class MyComponent extends React.Component {
constructor(props) {
super (props);
this .state = {
count: 0,
name: 'John' ,
isLoggedIn: false
};
}
render() {
return (
<div>
<h1>Hello, { this .state.name}!</h1>
<p>Count: { this .state.count}</p>
<p>User is logged in : { this .state.isLoggedIn ? 'Yes' : 'No' }</p>
</div>
);
}
}
export default MyComponent;
|
In React, you update state using the setState()
method.
Javascript
import React, { useState } from 'react' ;
function MyComponent() {
const [count, setCount] = useState(0);
const handleClick = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={handleClick}>Increment</button>
</div>
);
}
|
The setState()
method in React is used to update the state of a component, triggering a re-render with the updated state. It manages state changes asynchronously that allows React to batch multiple updates for performance optimization. When calling setState()
, React merges the provided state updates with the current state, updating only the specified properties.
Every React Component has a lifecycle of its own, lifecycle of a component can be defined as the series of methods that are invoked in different stages of the component’s existence. A React Component can go through four stages.
18. Explain the lifecycle phases of a class component.
Class Component can go through stages of its life as follows.Â
- Mounting Phase:
constructor()
: Initialize state and bind event handlers.
render()
: Render UI based on state and props.
componentDidMount()
: Perform side effects after the component is rendered.
- Updating Phase:
render()
: Re-render UI based on state or prop changes.
componentDidUpdate()
: Perform side effects after re-rendering.
- Unmounting Phase:
componentWillUnmount()
: Clean up resources before the component is removed from the DOM.
- Error Handling:
componentDidCatch()
: Handle errors during rendering or in lifecycle methods of child components.
Purpose of componentDidMount lifecycle method
- Initialization: componentDidMount is a crucial lifecycle method in React that gets invoked after a component has been successfully inserted into the DOM (Document Object Model).
- Asynchronous Operations:Â It is particularly useful for handling asynchronous operations such as data fetching.
- Interacting with the DOM:Â If your component needs to interact directly with the DOM or other JavaScript frameworks, componentDidMount is the appropriate stage for such operations.
- Integration with External Libraries:Â When integrating React with external libraries or non-React code, componentDidMount allows you to safely initiate these integrations.
To fetch data from an API inside a React component, you typically use the fetch()
API or a library like Axios.
componentDidMount() {
// Fetch data from the API
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
// Update state with the fetched data
this.setState({ data, loading: false });
})
.catch(error => {
console.error('Error fetching data:', error);
this.setState({ loading: false });
});
}
21. What is the purpose of the componentWillUnmount()
method?
Purpose of the componentWillUnmount()
method:
- Cleanup Tasks:
componentWillUnmount()
is commonly used to perform cleanup tasks such as removing event listeners, canceling network requests, or unsubscribing from external data sources.
- Release Resources:
componentWillUnmount()
provides a hook to release resources which are no longer needed and ensure that they are properly cleaned up before the component is removed from the DOM.
- Prevent Memory Leaks:
componentWillUnmount()
allows you to release resources and unsubscribe from external data sources, preventing memory leaks and ensuring that resources are properly managed throughout the component’s lifecycle.
In React, you handle events using JSX by passing event handler functions as props to React elements.
function MyComponent() {
const handleClick = () => {
console.log('Button clicked');
};
return (
<button onClick={handleClick}>Click Me</button>
);
}
In React, you can conditionally render elements by using JavaScript expressions inside JSX.
function MyComponent({ isLoggedIn }) {
if (isLoggedIn) {
return <p>Welcome, user!</p>;
} else {
return <p>Please log in to continue.</p>;
}
}
The purpose of the &&
operator in JSX is to conditionally render elements based on a boolean expression. It allows you to render an element only if the expression evaluates to true, and if it evaluates to false, it will not render anything.
function MyComponent({ isLoggedIn }) {
return (
<div>
{isLoggedIn && <p>Welcome, user!</p>}
</div>
);
}
In React, you can use the ternary operator (condition ? trueValue : falseValue
) for conditional rendering. This allows you to render different JSX elements based on a condition.
function MyComponent({ isLoggedIn }) {
return (
<div>
{isLoggedIn ? (
<p>Welcome, user!</p>
) : (
<p>Please log in to continue.</p>
)}
</div>
);
}
The key
prop in React is a special attribute used to uniquely identify elements within a collection (such as an array) when rendering lists of elements. It provides efficient updates to the UI by helping React determine which items have changed, been added, or been removed from the list. Keys should be unique among siblings and stable between renders, and they are essential for optimizing rendering performance and enabling efficient reconciliation of list updates.
27. How do you render a list of items in React?
In React, you can render a list of items by mapping over an array of data and returning JSX elements for each item.
function MyList({ items }) {
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
}
In React, the map()
function is used for transforming arrays of data into arrays of JSX elements, and provides dynamic rendering of lists or collections of items in components. By iterating over each item in an array, map()
allows you to perform operations on data, generate unique keys for efficient list rendering, and construct JSX elements based on data.
29. What is component composition in React?
Component composition in React is used for building complex user interfaces by combining smaller, reusable components together. This helps breaking down the UI into smaller, more manageable pieces, each responsible for specific functionality or UI elements. By combining these components hierarchically and passing data and behavior through props, you can create scalable, maintainable, and customizable UIs.
In React, you compose multiple components together by nesting them within each other’s JSX. This hierarchical composition allows you to build complex user interfaces by combining smaller, reusable components into larger ones.
Javascript
import React from 'react' ;
function Button({ onClick, children }) {
return <button onClick={onClick}>{children}</button>;
}
function App() {
const handleClick = () => {
console.log( 'Button clicked' );
};
return (
<div>
<h1>Welcome to My App</h1>
<Button onClick={handleClick}>Click Me</Button>
</div>
);
}
export default App;
|
Higher-order components or HOC is the advanced method of reusing the component functionality logic. It simply takes the original component and returns the enhanced component.
Syntax:
const EnhancedComponent = higherOrderComponent(OriginalComponent);
Reason to use Higher-Order component:
- Easy to handle
- Get rid of copying the same logic in every component
- Makes code more readable
Error boundaries are a React component that catches JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of crashing the entire component tree. They are used to ensure that errors in one part of the UI don’t affect the rest of the application.
Purpose and usage of the componentDidCatch()
method:
- Error Handling: When an error occurs within the component tree of a component, React will call this method with two arguments:
error
(the error that occurred) and info
.
- Error Logging: Inside the
componentDidCatch()
method, you can log the error, inspect the info
object to determine where the error originated in the component tree, and perform any necessary error handling or recovery actions.
- Fallback UI: One common use case for
componentDidCatch()
is to render a fallback UI in place of the component tree where the error occurred. This fallback UI can inform the user about the error and provide options to recover from it or report it.
- Error Boundary: Components that implement
componentDidCatch()
are often referred to as “error boundaries.” They act as a barrier that catches errors occurring within their child component tree and provides a fallback UI.
In React, you can handle errors in a component using the componentDidCatch()
lifecycle method.
Javascript
class ErrorBoundary extends Component {
constructor(props) {
super (props);
this .state = { hasError: false };
}
componentDidCatch(error, info) {
console.error( 'Error caught by error boundary:' , error, info);
this .setState({ hasError: true });
}
render() {
if ( this .state.hasError) {
return <p>Something went wrong. Please try again later.</p>;
}
return this .props.children;
}
}
const App = () => {
return (
<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
);
};
export default App;
|
We can perform some actions and precautions to optimize the performance.
1. Use binding functions in constructors: By adding an arrow function in a class, we add it as an object and not as the prototype property of the class. The most reliable way to use functions is to bind them with the constructor.
2. Avoid inline style attributes: The browser often invests a lot of time rendering, when styles are implied inline. Creating a separate style.js file and importing it into the component is a faster method.
3. Avoid bundling all of the front end code in a single file: By splitting the files into resource and on-demand code files we can reduce the time consumed in presenting bundled files to the browser transformers.
4. Avoid inline function in the render method: If we use the inline function, the function will generate a new instance of the object in every render and there will be multiple instances of these functions which will lead to consuming more time in garbage collection.
Memoization in React is the process of optimizing functional components by caching the result of expensive computations and reusing it when the component is re-rendered with the same props. This technique helps to avoid unnecessary re-computation of values, thereby improving performance by reducing the workload on the rendering process.
Code-splitting in React is a technique used to improve the performance of web applications by splitting the JavaScript bundle into smaller chunks, which are loaded asynchronously at runtime. This allows the browser to download only the necessary code for the current view, reducing the initial load time and improving the overall performance of the application.
There are various way by which we can style our react components:
Inline CSS: In inline styling basically, we create objects of style. And render it inside the components in style attribute using the React technique to incorporate JavaScript variable inside the JSX (Using ‘{ }’ )
Styled Components:Â The styled-components allows us to style the CSS under the variable created in JavaScript. styled-components allow us to create custom reusable components which can be less of a hassle to maintain.
CSS Modules: A CSS module is a simple CSS file but a key difference is by default when it is imported every class name and animation inside the CSS module is scoped locally to the component that is importing it also CSS file name should follow the format ‘filename.module.css’.Â
Share your thoughts in the comments
Please Login to comment...