In this article, you will learn React Hooks interview questions and answers that are most frequently asked in interviews. Before proceeding to learn React Hooks interview questions and answers, first learn the complete React Hooks.
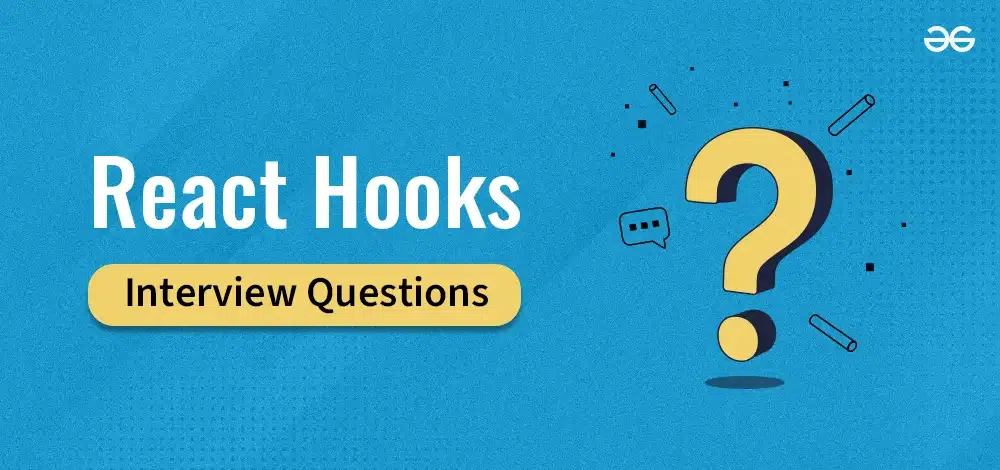
React hooks are functions that enable functional components to use state and lifecycle features that were previously only available in class components. Hooks provide functions like useState, useEffect, useContext, etc., that allow you to “hook into” React features from functional components.
React Hooks Interview Questions and Answers
Let’s discuss some common questions that you should prepare for the interviews. These questions will help clear the interviews, specially for the frontend development or full stack development role.
This set contains the basic questions asked in the interview.
React Hooks is a function that lets you use state and other React features without writing a class. They were introduced in React 16.8 to enable functional components to use state which means to make the code good and easy to understand.
2. What problems do React Hooks solve?
React Hooks solves the problems of sharing the stateful logic between components in a more modular and reusable way than class component.
The basic built-in React Hooks are useState, useEffect, useContext, useReducer, useCallback, useMomo, useRef and useImperativeHandle.
- useState enables components to manage and update their own state without using classes.
- useEffect is used to connect component to an external system.
- useContext
it is
used to consume data from a Context in a functional component.
- useReducer is used to manage complex state logic through a reducer function.
- useCallback used to memoize functions, preventing unnecessary re-renders in child components.
- useMemo is used to memoize the result of a function computation, preventing unnecessary recalculations.
- useRef is used to create mutable references that persist across renders in functional components.
- useImperativeHandler customizes the instance value that is exposed when using
ref
with functional components.
4. How does useState work?
The useState Hook enables you to add state to your functional components. It returns a stateful value and a function that can be used to update that value. By using Hooks, you can extract stateful logic from your component, which can then be tested independently.
5. When would you use useEffect?
useEffect
is typically used in React functional components to perform side effects, like data fetching, subscriptions, or DOM manipulations, after the component has rendered. It’s similar to lifecycle methods in class components, but it’s more flexible and concise.
6. What is the purpose of useCallback Hooks?
The purpose of useCallback Hooks is used to memoize functions, and prevent unnecessary re-rendering of child components that rely on those components. The useCallback function in React is mainly used to keep a reference to a function constant across multiple re-renders. This feature becomes useful when you want to prevent the unnecessary re-creation of functions, especially when you need to pass them as dependencies to other hooks such as useMemo or useEffect.
7. Explain the difference between useMemo and useCallback?
Here’s a difference of useMemo
and useCallback int tabular form
:
useMemo |
useCallback |
Memoizes a value (result of a function) |
Memoizes a function |
Memoized value |
Memoized function reference |
When you need to memoize a calculated value |
When you need to memoize a function |
Recalculates when any dependency changes |
Recreates the function only when any dependency changes |
Example: Memoizing the result of expensive computations |
Example: Memoizing event handler functions to prevent re-renders |
8. What is the useContext used for?
useContext is a function that enables access to context values provided by a Context.Provider component at a higher level in the component tree. This allows data to be passed down the tree without the need to manually pass props at each level.
useState |
useReducer |
Handles state with a single value |
Handles state with more complex logic and multiple values |
Simple to use, suitable for basic state needs |
More complex, suitable for managing complex state logic |
Simple state updates, like toggles or counters |
Managing state with complex transitions and logic |
Directly updates state with a new value |
Updates state based on dispatched actions and logic |
Not used |
Requires a reducer function to determine state changes |
Logic is dispersed where state is used |
Logic is centralized within the reducer function |
useEffect Hooks is used to connect component to an external system. In this the depenedeny array specifies when the effect should run based on changes in its dependencies and when the dependency array is absent in useEffect
, the effect will run after every render.
When you provide dependencies in the dependency array of useEffect
, the effect will run:
- Initially after the first render.
- Whenever any of the dependencies change between renders.
- If the dependencies are an empty array (
[]
), the effect will only run once after the initial render, similar to componentDidMount
in class components.
11. When would you use useRef?
useRef
is used in React functional components when you need to keep a mutable value around across renders without triggering a re-render. It’s commonly used for accessing DOM elements, caching values, or storing mutable variables. You can use useRef
to manage focus within your components, such as focusing on a specific input element when a condition is met without triggering re-renders.
useLayoutEffect is similar to useEffect but fires synchronously after all DOM mutations. It’s useful for reading from the DOM or performing animations before the browser paints. Due to its synchronous nature, excessive usage of useLayoutEffect
may potentially impact performance, especially if the logic within it is computationally intensive or blocking. It’s essential to use useLayoutEffect
judiciously and consider performance implications carefully.
Custom hooks in React are like your own personal tools that you can create to make building components easier. They’re functions you write to do specific tasks, and then you can reuse them in different components. They’re handy because they let you keep your code organized and share logic between different parts of your application without repeating yourself.
14. How do you create a custom Hook?
Here is to creating a custom Hooks step-by-step.
- Create a function: Start by defining a regular JavaScript function. It can have any name, but it’s a convention to prefix it with “use” to indicate that it’s a hook.
- Write the logic: Inside your custom hook function, write the logic you want to encapsulate and reuse. You can use existing hooks like
useState
, useEffect
, or other custom hooks if needed.
- Return values: Ensure your custom hook returns whatever values or functions you want to expose to components that use it.
- Use the hook: You can now use your custom hook in any functional component by calling it. React will recognize it as a hook because it starts with “use”.
15. What is the benefit of using custom Hooks?
The benefits of using custom hooks in React include code reusability, separation of concerns, abstraction of complex logic, improved testability, and the ability to compose hooks together for flexible and scalable code. They help in maintaining a clear separation between presentation and business logic, making it easier to reason about and maintain your codebase as it grows.
16. Can custom Hooks accept parameters?
Yes, custom hooks in React can indeed accept parameters. By accepting parameters, custom hooks become more flexible and can adapt their behavior based on the specific needs of different components.
Javascript
import { useEffect } from 'react' ;
function useDocumentTitle(title) {
useEffect(() => {
document.title = title;
}, [title]);
}
function MyComponent() {
useDocumentTitle( 'Hello GfG!' );
return <div>GeeksforGeeks content...</div>;
}
|
17. How do you share code between custom Hooks?
To share code between custom hooks in React, you can simply create regular JavaScript functions or utility functions and import them into your custom hooks as needed. These shared functions can encapsulate common logic or helper functions that are used across multiple custom hooks.
The rules of Hooks are:
- Only Call Hooks at the Top Level: Don’t call Hooks inside loops, conditions, or nested functions. Always use Hooks at the top level of your functional components or other custom Hooks.
- Only Call Hooks from React Functions: Ensure that you only call Hooks from within React functional components or custom Hooks. Don’t call Hooks from regular JavaScript functions.
These rules are crucial for ensuring that React can properly track the state of hooks and manage their lifecycles correctly. Violating these rules can lead to unexpected behavior and bugs in your application.
19. How do you conditionally run effects with useEffect?
To conditionally run effects with useEffect
in React, you can use the dependency array as the second argument to specify the values that the effect depends on. By updating these dependencies, you can control when the effect should run.
Javascript
import React, {
useEffect,
useState
}
from 'react' ;
import { fetchData }
from './actions/actions' ;
function App({ condition }) {
const [data, setData] = useState( null );
useEffect(() => {
if (condition) {
fetchData().then(result =>
setData(result));
}
}, [condition]);
return (
<div>
{data ? (
<p>
Data available: {data}
</p>
) : (
<p>No data available</p>
)}
</div>
);
}
export default App;
|
20. What happens if you omit dependencies in the dependency array of useEffect?
If you omit dependencies in the dependency array of useEffect
, the effect will run after every render. This means that the effect will be executed on the initial render and after every subsequent re-render, regardless of whether any specific values have changed.
Javascript
import React, {
useEffect,
useState
}
from 'react' ;
function App() {
const [count, setCount] = useState(0);
useEffect(() => {
console.log( "See the Effect here" );
});
return (
<div>
<p>Count: {count}</p>
<button onClick={() =>
setCount(count + 1)}>
Increment
</button>
</div>
);
}
export default App;
|
21. How do you fetch data with useEffect?
You can fetch data with useEffect
in React by performing the data fetching operation inside the effect function. Typically, you use asynchronous functions like fetch
to make HTTP requests to an API endpoint and update the component state with the fetched data.
Javascript
import React,
{
useEffect,
useState
}
from 'react' ;
function App() {
const [count, setCount] = useState(0);
useEffect(() => {
console.log( "See the Effect here" );
});
return (
<div>
<p>Count: {count}</p>
<button onClick={() =>
setCount(count + 1)}>
Increment
</button>
</div>
);
}
export default App;
|
22. What is the purpose of the second argument in useState?
The purpose of the second argument in useState
is to initialize the state value. When you call useState(initialValue)
, the initialValue
provided as the second argument is used to initialize the state variable.
const [count, setCount] = useState(0);
In this '
0
'
is the initial value provided as the second argument to useState
, so count
will be initialized with ‘0
'
as its initial value.
23. Explain the concept of lazy initialization with useState?
Lazy initialization with useState allows you to initialize state lazily based on a function, which is only called on the initial render. The concept of lazy initialization with useState
in React allows you to initialize state lazily, i.e., on-demand when it’s needed, rather than eagerly during component initialization. This means that you can compute the initial state dynamically based on some conditions or heavy computations.
24. What are the benefits of using React.memo?
React.memo
is a higher-order component provided by React that can be used to optimize functional components by memoizing them. The benefits of using React.memo
include improved performance by memoizing component renders based on props, reduced re-renders, enhanced component reusability, and simplified optimization without manual memoization logic.
25. How do you test components that use Hooks?
You can test components that use hooks in React using testing libraries like @testing-library/react
or enzyme
. Write test cases to simulate component rendering, user interactions, and expected outcomes. Use testing utilities like render
, fireEvent
, and expect
to interact with components and assert their behavior, ensuring hooks are working correctly.
No, you cannot use hooks in class components directly. Hooks are a feature introduced in React 16.8 to allow stateful logic to be used in functional components. They cannot be used in class components because hooks rely on the functional component’s call order to manage state and lifecycle behaviors, which is not compatible with the class component’s lifecycle methods.
27. How do you handle forms with Hooks?
You can handle forms with hooks in React by using the useState
hook to manage form state, and the onChange
event handler to update the state as the user interacts with the form inputs. Additionally, you can use the handleSubmit
function to handle form submission and perform any necessary actions, such as sending data to a server or updating the UI based on form input.
The useImperativeHandle
hook in React allows you to customize the instance value that is exposed when using ref
with functional components. It’s typically used to expose specific methods or properties from a child component’s instance to its parent component.
29. How do you share state logic between components?
You can share state logic between components in React using techniques such as prop drilling, lifting state up, or by using state management libraries like Redux or React Context.
- Prop Drilling: Pass state and functions down through props from parent to child components. This is suitable for simple applications with a shallow component tree.
- Lifting State Up: Move shared state and related functions to the nearest common ancestor component and pass them down as props to child components. This is effective for managing state across multiple related components.
- State Management Libraries: Use state management libraries like Redux or React Context for managing global state that needs to be accessed by multiple components across the application. These libraries provide centralized state management and make it easier to share state logic across components.
30. What are some common patterns for using custom Hooks?
Some common patterns for using custom hooks in React are:
- State Management: Custom hooks can encapsulate stateful logic, making it reusable across components.
- Side Effects: Custom hooks can handle side effects such as data fetching, subscriptions, or imperative DOM manipulations.
- Abstraction of Complex Logic: Custom hooks can abstract complex logic into reusable functions, improving code readability and maintainability.
- Composition: Custom hooks can be composed together to create more complex behavior.
- Integration with Third-party Libraries: Custom hooks can integrate with third-party libraries or APIs, providing a clean and reusable interface.
Share your thoughts in the comments
Please Login to comment...