How does JSX differ from HTML ?
Last Updated :
13 Feb, 2024
JSX, a syntax extension for JavaScript, allows the creation of user interfaces similar to HTML but within JavaScript. Unlike HTML, JSX enables the embedding of JavaScript expressions and facilitates the creation of reusable components, promoting a more dynamic and efficient approach to web development.
JSX ( JavaScript XML)
JSX stands for JavaScript XML. It is a syntax extension for JavaScript, often used with React to describe what the UI should look like. JSX may remind you of a template language, but it comes with the full power of JavaScript.
Syntax
const element = (
<div>
<h1>Hello, world!</h1>
<p>This is a JSX element.</p>
</div>
);
Features of JSX
- Embedding Expressions: JSX allows embedding JavaScript expressions within curly braces, that enable dynamic content rendering.
- Components: JSX supports the creation of reusable components, facilitating modular development and code organization.
- Conditional Rendering: JSX supports conditional rendering through JavaScript expressions, allowing components to render different content based on conditions.
Example: Illustration of JSX code.
Javascript
import React from 'react' ;
const JSXComponent = () => {
const greeting = 'Hello, JSX!' ;
return (
<div>
<h1>{greeting}</h1>
<p>This is a pure JSX component.</p>
</div>
);
};
export default JSXComponent;
|
Output:
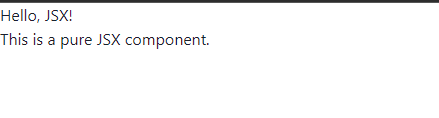
HTML ( HyperText Markup Language )
HTML, or HyperText Markup Language, is the standard markup language for documents designed to be displayed in a web browser. It can be assisted by technologies such as Cascading Style Sheets (CSS) and scripting languages such as JavaScript.
Syntax
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>Pure HTML Example</title>
</head>
<body>
<div>
<!-- Code -->
</div>
</body>
</html>
Features of HTML
- Semantics: HTML provides semantic tags like
<header>
, <nav>
, <footer>
, etc., which convey the meaning and structure of the content to both developers and browsers.
- Forms: HTML provides form elements and attributes for collecting user input, including text inputs, checkboxes, radio buttons, and more, with built-in validation features.
- Multimedia Support: HTML supports embedding multimedia content like images, audio, and video, enabling rich media experiences within web pages.
Example: Illustration of code example of HTML.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Pure HTML Example</ title >
</ head >
< body >
< div >
< h1 >Hello, HTML!</ h1 >
< p >This is a pure HTML page.</ p >
</ div >
</ body >
</ html >
|
Output:
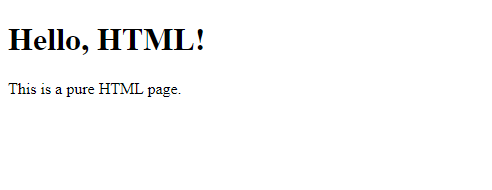
Difference between JSX and HTML
The table below illustrates the differences between the JSX and HTML.
Feature |
JSX |
HTML |
Case Sensitivity |
JSX is case-sensitive with camelCase properties. |
HTML is not case-sensitive.
|
Logic Integration |
JSX allows JavaScript expressions. |
HTML does not have logic built-in; it requires a separate script.
|
Node Structure |
JSX requires a single root node. |
HTML does not have this restriction.
|
Attribute/Property Naming |
Attributes are camelcased (e.g., onClick). |
Attributes are lowercase (e.g., click).
|
Custom Components |
JSX can have custom components (e.g., <MyComponent />). |
HTML does not support custom components natively. |
Share your thoughts in the comments
Please Login to comment...