How does React.memo() optimize functional components in React?
Last Updated :
01 Mar, 2024
React.memo() is a higher-order component provided by React that can help optimize functional components by reducing unnecessary re-renders.
Let’s explore how it works and how it optimizes functional components:
Optimizing with React.memo():
- Reducing Re-renders: When a React component renders, it compares the previous render’s output with the current render’s output to determine if any updates are needed. If the output is the same, React skips the re-rendering process for that component and its subtree, which helps improve performance.
- Memoization: `React.memo()` utilizes memoization to optimize functional components. Memoization is caching the results of expensive function calls and reusing them when the same inputs occur again.
- Comparing Props: When you wrap a functional component with `React.memo()`, React memorizes the component’s props. Before rendering the component, React compares the new props with the previous props. React reuses the previous render result if the props have not changed, skipping the re-rendering process altogether.
- Shallow Comparison: Under the hood, `React.memo()` performs a shallow comparison of props using `Object.is()` to determine if the props have changed. This means that it only compares the props at the top level and doesn’t perform a deep comparison of nested objects or arrays.
- Caveats: While `React.memo()` can be a powerful optimization tool, it’s essential to use it judiciously. Memoizing a component with `React.memo()` can be beneficial only if the component’s render output is expensive to compute and the component’s props are often the same between renders. If the props change frequently, memoization may not provide significant performance gains.
When to use React.memo() ?
When incorporating React.memo(), it’s crucial to exercise caution, as excessively memoizing components can lead to reduced performance by introducing unnecessary overhead. To optimize effectively, consider the following best practices:
- Memoize components with substantial rendering costs or intricate logic that infrequently changes, such as table rows or product cards.
- Memoize components that receive consistent props but may exhibit distinct internal states or behaviors.
- Memoize components integrated into frequently re-rendered lists, like those in tables or lists.
Benefits of memoization using React.memo():
- For a component displaying the current date and time, where re-rendering with every parent component update is unnecessary, given the infrequent changes once per second.
- When dealing with a component showcasing a list of items subject to filtering or sorting, memoization can prevent unnecessary re-renders of each list item.
Strike a balance in optimizing and memoization efforts, avoiding unnecessary complexities while enhancing performance.
How to use React.memo() ?
React.memo() is easy to use and intuitive. Here’s how to use React.memo() to memoize a functional component step-by-step:
Step 1: Import your React.memo() file at the top.
import React, { memo } from 'react';
Step 2: To define your functional component using the below syntax.
const FunctionalComponet = ({ prop1, prop2 }) => {
return <div>{prop1} {prop2}</div>;
};
Step 3: To Memoize your component by wrapping it with React.memo():
const ToMemoized = memo(FuntionalComponent);
Step 4: To use your memoized component in your parent component using the following syntax:
const ComponentParent = () => {
return (
<div>
<ToMemoized prop1="Hello" prop2="GeeksforGeeks" />
</div>
);
};
By applying React.memo() to your functional component, it is now memoized, ensuring that it will only re-render when there are changes in its props. The usage of memo is straightforward and simplifies the management of component rendering.
Example: In this example, `FuntionalComponent` is wrapped with `React.memo()`, which memoizes the component based on its props. If the `prop1` and `prop2` props remain the same between renders, React will reuse the previous render result, preventing unnecessary re-renders.
Javascript
import React from 'react' ;
const FunctionalComponent = React.memo(({ prop1, prop2 }) => {
return (
<div>
<p>Prop 1: {prop1}</p>
<p>Prop 2: {prop2}</p>
</div>
);
});
export default FunctionalComponent;
|
Javascript
import React from 'react' ;
import FunctionalComponent from './AnotherComponent' ;
const ComponentParent = () => {
const exampleProp1 = "Hello" ;
const exampleProp2 = 42;
return (
<div>
<h2>Parent Component</h2>
<FunctionalComponent
prop1={exampleProp1} prop2={exampleProp2} />
</div>
);
};
export default ComponentParent;
|
Output:
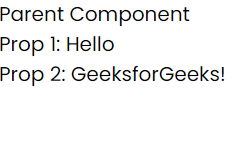
Output
Conclusion:
In summary, `React.memo()` optimizes functional components by memoizing them based on their props. By comparing the new props with the previous props and reusing the previous render result when the props haven’t changed, `React.memo()` helps reduce unnecessary re-renders and improves the performance of React applications. However, it’s essential to use memoization selectively and consider the specific use case before applying it.
Share your thoughts in the comments
Please Login to comment...