How are events handled in React?
Last Updated :
08 Feb, 2024
Modern webpages rely on user interactions, triggering events like clicks or keypresses. React facilitates event handling with built-in methods, allowing developers to create listeners for dynamic interfaces and responses. JavaScript events, inherent to the DOM, use bubbling propagation by default, moving upwards from children to parent elements, and can be bound either inline or in external scripts.
Prerequisites:
What are events in react?
React JS Events refer to user interactions with the web application, such as clicks, keyboard input, and other actions that trigger a response in the user interface. React allows developers to handle these events using a declarative approach, making it easier to create interactive and dynamic user interfaces.
In React, event handling is similar to traditional DOM event handling but with some key differences. Instead of using inline event handlers like onclick
, React utilizes synthetic events, which are cross-browser wrappers around the browser’s native events.
Some common events in React include:
- onClick: Triggered when an element is clicked by the user.
- onChange: Fired when the value of an input element changes, such as in text input fields or select dropdowns.
- onSubmit: Invoked when a form is submitted, typically used to handle form validation and submission.
- onMouseOver and onMouseOut: Triggered when the mouse pointer enters or leaves an element’s boundaries.
- onKeyDown, onKeyPress, and onKeyUp: Fired when a key is pressed, providing opportunities for keyboard interaction.
Handling events in React
In React, events are handled similarly to how they are handled in regular HTML. However, in React, event handling is done using JSX syntax, and React provides its own synthetic event system which is compatible with most browser events.
Steps for Creating React Application to handle events:
Step 1: Create a react application using the following command.
npx create-react-app Name-for-your-app
cd Name-for-your-app
Project Structure:
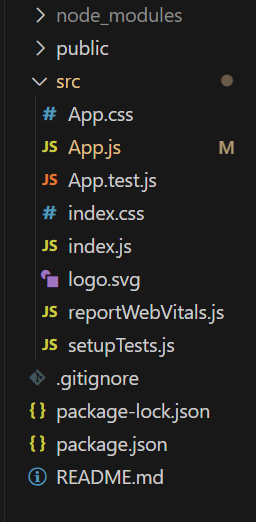
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: Below is the Example code which we have to add in app.js file:
Javascript
import React, { useState } from "react" ;
function App() {
const [count, setCount] = useState(0);
const handleClick = () => {
setCount(count + 1);
};
return (
<div className= "App" >
<h1>Hello React!</h1>
<div>
<p>Count: {count}</p>
<button onClick={handleClick}>Click me</button>
</div>
</div>
);
}
export default App;
|
Output:
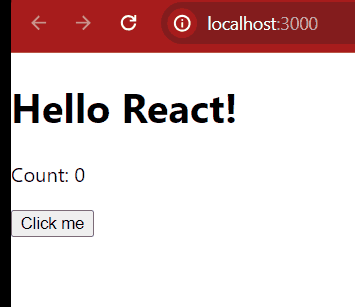
Share your thoughts in the comments
Please Login to comment...