Migrating from Class Components to Functional Components in React
Last Updated :
15 Mar, 2024
React version 16.8.0 or higher allows users to write the functional components without the use of class components. Using class components, we need to write the whole class to interact with the state and lifecycle of the component. The code was quite complex and harder to understand which led to lower performance and limited usability.
With the help of functional components, it has become easier to manage the state and lifecycle of a component. We don’t need to define any class. Directly declare the components and use them wherever required. The code is quite simple, easy to understand, reusable, and manageable.
The State Management in React using class and functional component
Class Component state: setState:
To add a state to a class component using the setState, initialize the state in the constructor and declare the methods with the class itself. To set the state we need to create an additional function defining the update that is to be done in the state.
Example: Below is an example of class component state.
Javascript
import React from 'react';
class Gfgarticle extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
this.increment = this.increment.bind(this);
}
increment() {
this.setState({ count: this.state.count + 1 });
}
render() {
return (
<div>
<h1>Counter Program</h1>
<p>You clicked {this.state.count} times</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
export default Gfgarticle;
Functional component state: useState
While using the useState as a Functional Component, initialize the state with some initial value and declare the function which whenever called helps to make updating the state.
Example: Below is an example of functional component state.
Javascript
import React, { useState } from "react";
function Gfgarticle() {
// Here is a react Hook
const [count, setCount] = useState(0);
return (
<div>
<h1>COUNTER PROGRAM</h1>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Increment
</button>
</div>
)
}
export default Gfgarticle;
Now let’s have a check on the lifecycle in react using both the class and functional component
The Lifecycle in React using class and functional component
Class Component LifeCycle
The Lifecycle of a class component required declaration of several sets of methods which includes componentDidMount, componentDidUpdate, componentWillUnmount, etc.
Example: Below is an example of class component lifecycle.
Javascript
import React from 'react';
class Gfgarticle extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
this.increment = this.increment.bind(this);
}
static getDerivedStateFromProps(props, state) {
console.log('getDerivedStateFromProps called', props, state);
return null;
}
componentDidMount() {
console.log('componentDidMount called');
}
shouldComponentUpdate(nextProps, nextState) {
console.log('shouldComponentUpdate called',
nextProps, nextState);
return true;
}
getSnapshotBeforeUpdate(prevProps, prevState) {
console.log('getSnapshotBeforeUpdate called',
prevProps, prevState);
return null;
}
componentDidUpdate(prevProps, prevState, snapshot) {
console.log('componentDidUpdate called',
prevProps, prevState, snapshot);
}
componentWillUnmount() {
console.log('componentWillUnmount called');
}
handleClick = () => {
this.setState(prevState => ({
count: prevState.count + 1
}));
};
increment() {
this.setState({ count: this.state.count + 1 });
}
render() {
return (
<div>
<h1>Counter Program</h1>
<p>You clicked {this.state.count} times</p>
<button onClick={this.handleClick}>Increment</button>
</div>
);
}
}
export default Gfgarticle;
You can observe the lifecycle method calls in the console as you interact with the component, helping you understand the sequence of events during the component’s lifecycle.
Here is the result of the above code
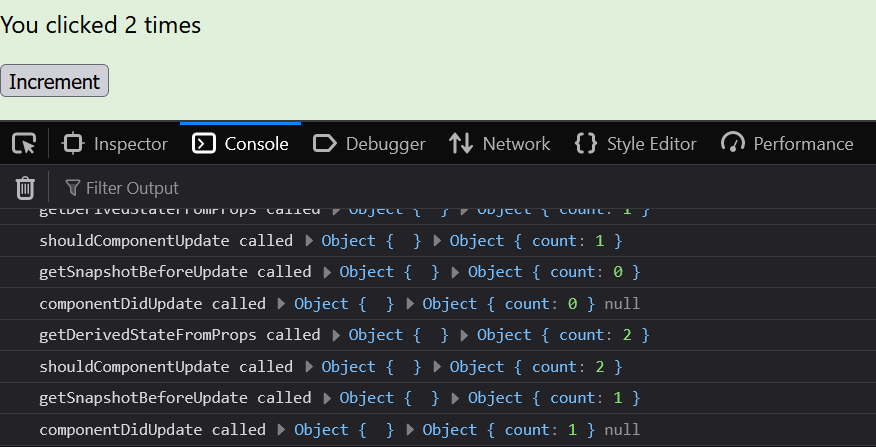
Functional Component LifeCycle
In the functional component, we simply use the useEffect hook to keep a track of the side effects and perform the cleanup tasks.
The same above lifecycle event using functional component is given as below:
Javascript
import React, { useState, useEffect } from "react";
function Gfgarticle() {
// Here is a react Hook
const [count, setCount] = useState(0);
useEffect(() => {
console.log('Component mounted');
return () => {
console.log('Component will unmount');
};
}, []);
useEffect(() => {
console.log('Count updated:', count);
}, [count]);
const handleClick = () => {
setCount(prevCount => prevCount + 1);
};
return (
<div>
<p>You clicked {count} times</p>
<button onClick={handleClick}>
Increment
</button>
</div>
)
}
export default Gfgarticle;
The life cycle using the functional component can easily be seen in the console.
Here is the result of the above code
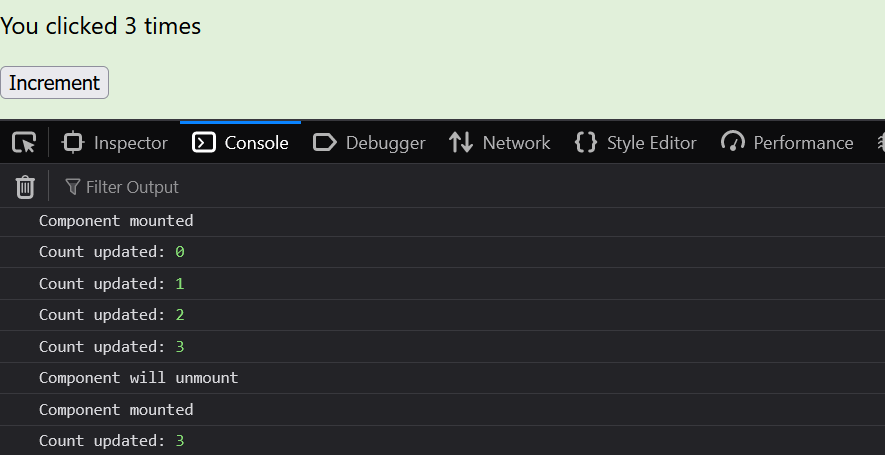
Conclusion:
In conclusion, while class components have been a cornerstone of React development for many years, they come with certain drawbacks, including complexity, limited reusability, and potential performance overhead. With the introduction of hooks in React, functional components have become the preferred way to define components, offering a simpler, more declarative, and more efficient approach to building React applications.
Share your thoughts in the comments
Please Login to comment...