Use of render() method in a Class Component.
Last Updated :
06 Feb, 2024
In React class components play an important role in building robust and scalable applications. In the class component render method is used for rendering user interface elements on the screen. In this article, we will understand the use of the render method.
Prerequisites:
Steps to Create React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Project Structure:
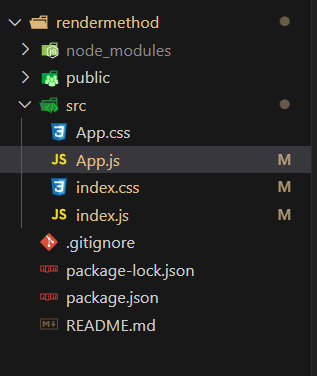
Project Structure
The updated dependencies in package.json file will look like.
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
The render Method can be implemented in several ways and each approach serves a different purpose. Let’s explore all possible approaches –
Now let’s understand all of these ways in detail –
Approach 1: Basic rendering Method:
The most fundamental use of the render method involves returning JSX elements to be displayed on the screen. Here is an example for understanding this-
Javascript
import React, { Component } from 'react' ;
class BasicRenderComponent extends Component {
render() {
return (
<div>
<h1>Hello, React!</h1>
<p>This is a basic render method example.</p>
</div>
);
}
}
export default BasicRenderComponent;
|
Output:
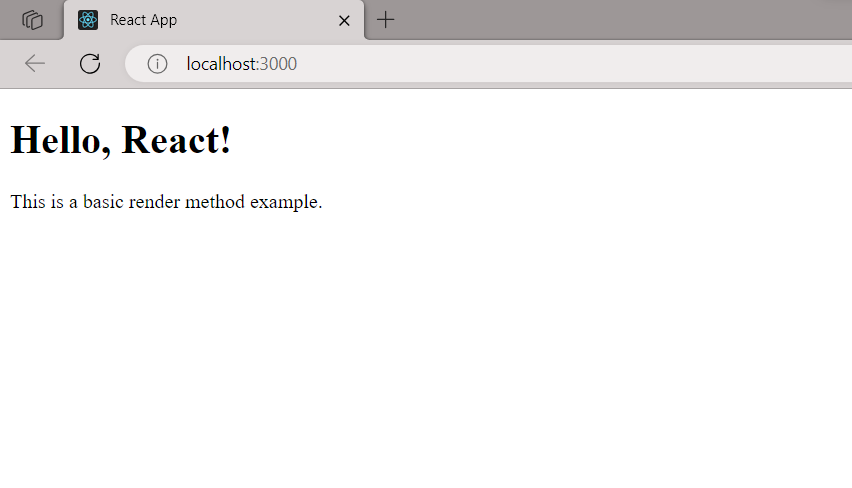
Approach 2: Conditional rendering :
The render method allows for conditional rendering, enabling components to display different content based on certain conditions:
Javascript
import React, { Component } from 'react' ;
class ConditionalRenderComponent extends Component {
render() {
const isLoggedIn = true ;
return (
<div>
{isLoggedIn ? <p>Welcome User!</p> : <p>Please log in .</p>}
</div>
);
}
}
export default ConditionalRenderComponent;
|
Output:
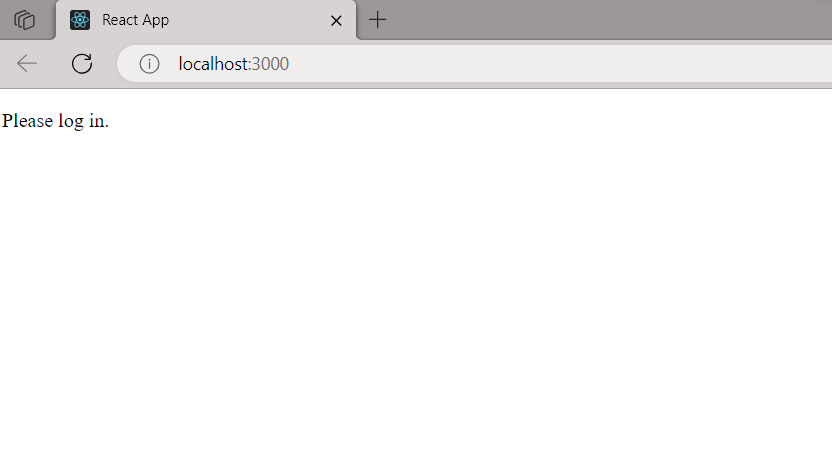
Approach 3: Iterative Rendering :
Rendering a list of elements is another powerful capability of the render method:
Javascript
import React, { Component } from 'react' ;
class IterativeRenderComponent extends Component {
render() {
const items = [ 'Item 1' , 'Item 2' , 'Item 3' ];
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
}
}
export default IterativeRenderComponent;
|
Output:
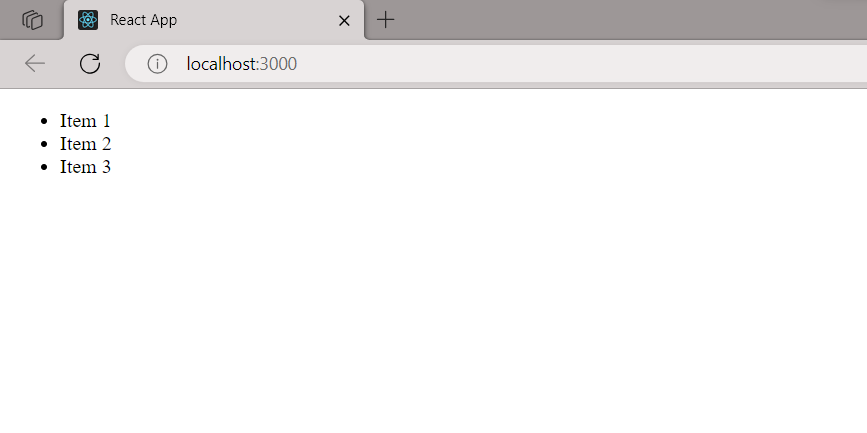
Share your thoughts in the comments
Please Login to comment...